【LeetCode & 剑指offer 刷题笔记】目录(持续更新中...)
Valid Sudoku
Determine if a 9x9 Sudoku board is valid. Only the filled cells need to be validated according to the following rules:
-
Each row must contain the digits 1-9 without repetition.
-
Each column must contain the digits 1-9 without repetition.
-
Each of the 9 3x3 sub-boxes of the grid must contain the digits 1-9 without repetition.
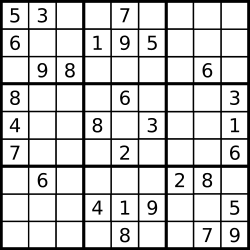
A partially filled sudoku which is valid.
The Sudoku board could be partially filled, where empty cells are filled with the character '.'.
Example 1:
Input:
[
["5","3",".",".","7",".",".",".","."],
["6",".",".","1","9","5",".",".","."],
[".","9","8",".",".",".",".","6","."],
["8",".",".",".","6",".",".",".","3"],
["4",".",".","8",".","3",".",".","1"],
["7",".",".",".","2",".",".",".","6"],
[".","6",".",".",".",".","2","8","."],
[".",".",".","4","1","9",".",".","5"],
[".",".",".",".","8",".",".","7","9"]
]
Output: true
Example 2:
Input:
[
["8","3",".",".","7",".",".",".","."],
["6",".",".","1","9","5",".",".","."],
[".","9","8",".",".",".",".","6","."],
["8",".",".",".","6",".",".",".","3"],
["4",".",".","8",".","3",".",".","1"],
["7",".",".",".","2",".",".",".","6"],
[".","6",".",".",".",".","2","8","."],
[".",".",".","4","1","9",".",".","5"],
[".",".",".",".","8",".",".","7","9"]
]
Output: false
Explanation: Same as Example 1, except with the 5 in the top left corner being
modified to 8. Since there are two 8's in the top left 3x3 sub-box, it is invalid.
Note:
-
A Sudoku board (partially filled) could be valid but is not necessarily solvable.
-
Only the filled cells need to be validated according to the mentioned rules.
-
The given board contain only digits 1-9 and the character '.'.
-
The given board size is always 9x9.
//问题:判断数独表的有效性
/*
方法:新建3个hash set,由于set中不能出现相同key值的元素,可以利用这点来判断
(题目中已经约定符号只有1-9和‘.’,所以问题较简单)
(用三个数组应该也可以实现,分别检查行、列、块)
*/
/*
class Solution
{
public:
bool isValidSudoku(vector<vector<char>>& board)
{
for(int i = 0; i<9; i++)
{
unordered_set<char> rowtable;
unordered_set<char> coltable;
unordered_set<char> blocktable;
// 某个block起始行列索引
// i = 0~2,r_b = 0,c_b = 0,3,6;
// i = 3~5,r_b = 3,c_b =0,3,6;
// i = 6~8,r_b = 6,c_b = 0,3,6.
int row_block = (i/3) * 3;
int col_block = (i%3) * 3;
for(int j = 0; j<9; j++)
{
int a1 = board[i][j]; //第i行所有元素
int a2 = board[j][i]; //第i列所有元素
int a3 = board[row_block+j/3][col_block+j%3]; //j/3:0,0,0,1,1,1,2,2,2 j%3:0,1,2,0,1,2,0,1,2 用于遍历某一块(/号缓变,%号快变)
if(a1 !='.' && rowtable.find(a1) != rowtable.end()) return false; //检查行
if(a2 !='.' && coltable.find(a2) != coltable.end()) return false; //检查列
if(a3 !='.' && blocktable.find(a3) != blocktable.end()) return false; //检查块
rowtable.insert(a1);
coltable.insert(a2);
blocktable.insert(a3);
}
}
return true;
}
};*/
//方法:用三个数组,借助key-value的思想,将数字转化为索引,值为出现次数
class Solution
{
public:
bool isValidSudoku(vector<vector<char>>& board)
{
· //每行中各元素出现次数统计,每列中各元素出现的次数统计,第二维表示数字(key)
int rowtable[9][9] = {0}, coltable[9][9] = {0}, blocktable[9][9] = {0};
for(int i = 0; i< 9; i++)
{
for(int j = 0; j<9; j++)
{
if(board[i][j] != '.')
{
int num = board[i][j] - '0' -1; //将数字与索引联系起来(索引以0开始)
int k = i/3*3 + j/3; //块索引,0~8,块每“大行”3个,故第一项要乘3,块坐标为(i/3,j/3),若将board看成3*3
if(rowtable[i][num] || coltable[j][num] || blocktable[k][num])
return false;
else
rowtable[i][num] = coltable[j][num] = blocktable[k][num] = 1;
}
}
}
return true;
}
};