威胁分析平台恶意软件规则的总结
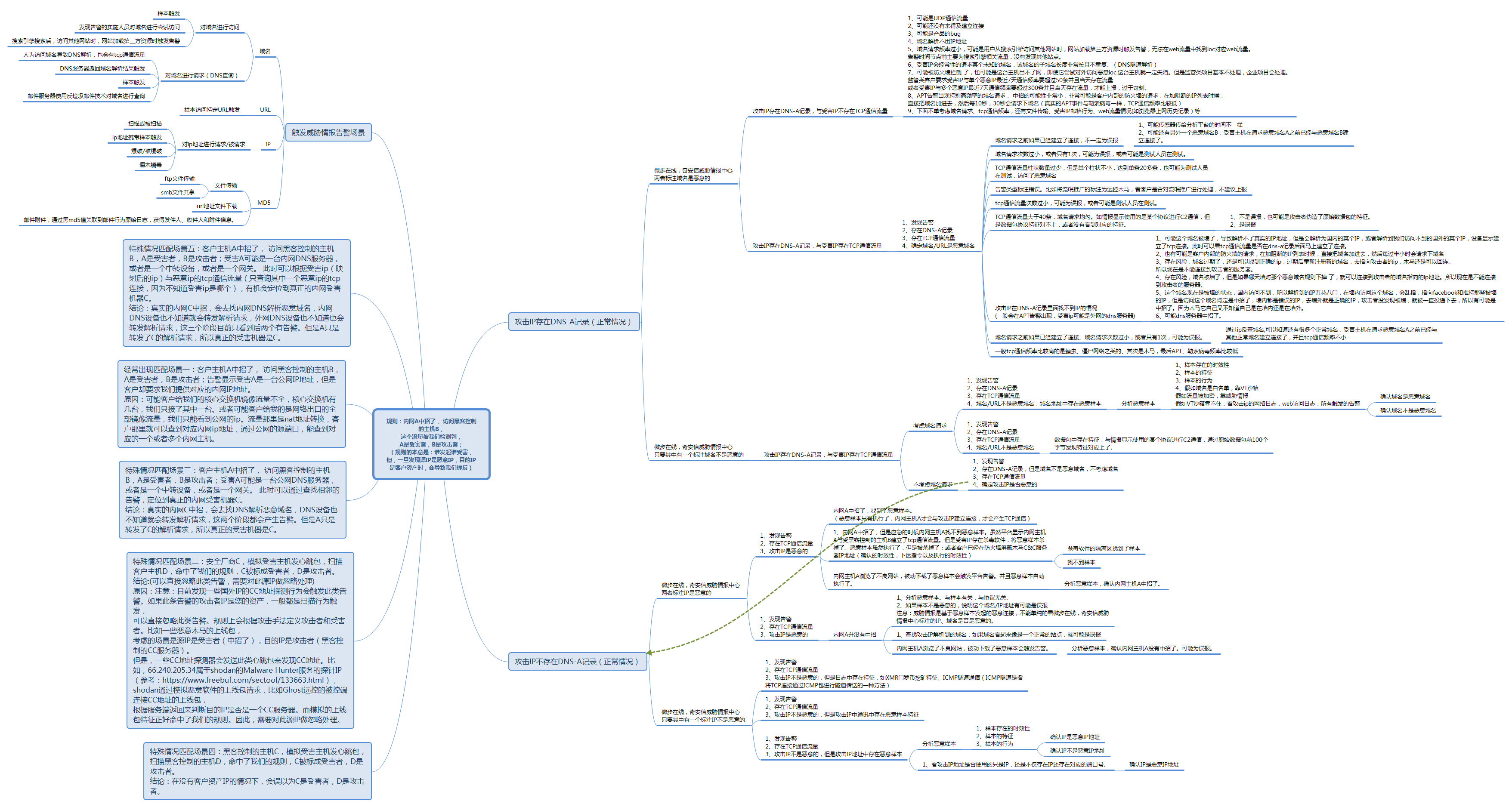
威胁分析平台恶意软件规则分析辅助脚本
1、有域名请求并且有TCP通信流量
1 #!/usr/bin/python3
2 #-*- coding:utf-8 -*-
3
4 #编写环境 windows 7 x64 + Notepad++ + Python3.7.0
5
6 '''
7 1、发现告警
8 2、存在TCP通信流量 sip:"10.10.10.10" AND dip:"100.100.100.100"(一周内存在流量,当天存在流量)
9 3、存在DNS-A记录 dip:"10.10.10.10" AND host:"恶意域名" AND addr:*
10 4、确定域名是恶意的
11 '''
12
13
14 import urllib3
15 urllib3.disable_warnings()
16 import sys
17 import time
18 import datetime
19
20 import requests
21 import xlrd
22 import json
23
24
25
26 #威胁分析平台地址
27 host="https://10.10.10.10"
28
29 headers ={
30 'Accept': 'application/json, text/plain, */*',
31 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/75.0.3770.100 Safari/537.36',
32 'Accept-Encoding': 'gzip, deflate',
33 'Accept-Language': 'zh-CN,zh;q=0.9',
34 'Cookie': 'session=1111111111111173ff9417aaed5f8e063a5218e',
35 }
36
37 ''' stime etime
38 2020-05-02 00:00:00-2020-05-02 14:27:13 1588348800000 1588400833356
39 2020-05-01 00:00:00-2020-05-01 23:59:59 1588262400000 1588348799999
40 2020-04-30 00:00:00-2020-04-30 23:59:59 1588176000000 1588262399999
41 2020-04-29 00:00:00-2020-04-29 23:59:59 1588089600000 1588175999999
42 2020-04-28 00:00:00-2020-04-28 23:59:59 1588003200000 1588089599999
43 2020-04-27 00:00:00-2020-04-27 23:59:59 1587916800000 1588003199999
44 2020-04-26 00:00:00-2020-04-26 23:59:59 1587830400000 1587916799999
45 '''
46
47 def tcp(tcp_detail):
48
49 #查询受害IP与C&C服务器通信次数
50 alarm_sip,alarm_dip,ioc = tcp_detail
51
52 try:
53 total_week = 0
54 etime = int(round(time.time()*1000))
55 for i in range(7):
56
57 ymd = time.strftime('%Y-%m-%d',time.localtime(etime/1000))#%Y-%m-%d %H:%M:%S
58 stime = int(time.mktime(time.strptime(str(ymd),"%Y-%m-%d")))*1000
59
60 url = host +'/xxx/zzz/getsearch?branch_id=223232&stime={stime}&etime={etime}&keyword=sip:%22{alarm_sip}%22+AND+dip:%22{alarm_dip}%22&index=xxx-tcpflow&category=xxx&interval=3h&page=1&size=20&asset_group_ids=&mode=advance_model&key_fields=@timestamp&graph_conf=%7B%7D'.format(stime=stime,etime=etime,alarm_sip=alarm_sip,alarm_dip=alarm_dip)
61
62 req = requests.get(url, headers=headers, verify=False).json()
63 #print(req)
64 total_week += req['data']['search']['total']
65 if i==1:
66 total_oneday = total_week
67 etime = stime-1
68
69 if total_week > 30 and total_oneday > 0:
70 return [alarm_sip, alarm_dip,ioc,total_week]
71 return [alarm_sip, alarm_dip,ioc,False]
72 except Exception as e:
73 print("tcp wrong")
74 print(e)
75 sys.exit()
76
77
78
79 #查询dns-A记录
80 def dns_a(threat_detail):
81 # 获取ioc对应的dns a记录,如果不存在解析记录返回列表包含false,存在解析记录获取全部返回页全部解析ip,去重后返回list
82 #etime结束时间 stime开始时间
83 dns_a_list = []
84 alarm_dip, alarm_sip, ioc, count= threat_detail
85 etime = int(round(time.time() * 1000))
86 #stime = etime - (4 * 60 * 60 * 1000) # 搜索前4小时的dns解析记录
87 stime = etime - (24 * 60 * 60 * 1000) # 搜索今天的dns解析记录
88
89 url = host + '/xxx/xxxx/xxxsearch?stime={stime}&etime={etime}&keyword=dip:%22{alarm_dip}%22+AND+host:%22{ioc}%22+AND+addr:*&index=xxx-dns&category=xxx&interval=3h&page=1&size=20&asset_group_ids=&mode=advance_model&key_fields=@timestamp'.format(stime=stime,etime=etime,alarm_dip=alarm_dip,ioc=ioc)
90 #print(url)
91
92 try:
93 req = requests.get(url, headers=headers, verify=False).json()
94
95 if req['data']['search']['total'] <= 0:
96 print('can not find dns a record',end = '
',flush = True)
97 return [alarm_dip, ioc , False]
98
99 for d in req['data']['search']['hits']:
100 for k,v in d.items():
101 if k=='_source':
102 for x,y in d[k].items():
103 if x=='addr':
104 #print(d[k][x])
105 dns_a_list.append(d[k][x])
106 #sorted(dns_a_list)
107 print('find dns a record', end='
',flush= True)
108 #去重
109 b=[]
110 for item in dns_a_list:
111 if item not in b:
112 b.append(item)
113 b=b[0]
114 return [alarm_dip, ioc ,b]
115
116
117 except Exception as e:
118 print("dns_a wrong")
119 print(e)
120 sys.exit()
121
122
123 #取xlsx第5列(受害IP)和第7列(攻击IP)存放到列表中(去重)
124 def get_xlsx_alarm_list():
125
126 try:
127 alarm_list = []
128 print(sys.argv[1])
129
130 #打开Excel文件读取数据
131 data = xlrd.open_workbook(sys.argv[1])
132
133 #获取一个工作表
134 table = data.sheets()[0] #通过索引顺序获取
135
136 #获取行数和列数
137 #nrows = table.nrows
138 #nclos = table.ncols
139
140 #遍历每一行
141 for i in range(1,table.nrows):
142 alarm_list.append((table.row_values(i)[4],table.row_values(i)[6],table.row_values(i)[9]))
143 return list(set(alarm_list))
144 except Exception as e:
145 print(e)
146 print('xlsx wrong')
147 sys.exit()
148
149
150 def login():
151 #https://10.10.10.10/xxx/ssxx/view
152 url =host + '/xxx/ssxx/view'; #找到返回结果是"未登陆或登陆超时!"的地址(JSON数据)
153
154 #获取威胁分析平台的版本信息
155 #https://10.10.10.10/xxxx/xxx/channel_version
156 #xxx_version = requests.get(host + '/xxx/admin/channel_version',headers=headers,verify=False).json()['data']['license_info']['version']
157 #print(xxx_version)
158
159 try:
160 #用requests来抓取网页里面的json数据
161 req1 =requests.get(url=url,headers=headers,verify=False).json()
162 if req1.get('status') == 200:
163 print('login success')
164 return True
165 else:
166 print(req1.get('message'))
167 return False
168 except Exception as e:
169 print(e)
170
171 def tcp_write():
172
173 alarm_list=get_xlsx_alarm_list()
174 #print(alarm_list)#[sip,dip]
175
176 for alarm in alarm_list:
177 tcprecord = tcp(alarm)
178 print(tcprecord)#['192.168.10.10', '181.61.119.171','ext.88.com', 12]
179
180 if tcprecord[3]:
181 dnsrecord = dns_a(tcprecord)
182 print(dnsrecord)#['192.168.10.10', 'ext.88.com', ['123.11.6.111', '113.11.16.103']]
183 if dnsrecord[2]:
184 print(dnsrecord)
185 dnsfile = open('tcp_dns_a_DNS.txt','a')
186 dnsfile.write(str(dnsrecord)+"
")
187
188 tcpfile = open('tcp_dns_a_TCP.txt','a')
189 tcpfile.write(str(tcprecord)+"
")
190
191 def main():
192 print("eg:python3 tcp.py 告警_列表导出2020-01-29 00_00_00至2020-02-04 20_07_59.xlsx")
193 starttime = time.time()
194 if(login()):
195 tcp_write()
196 endtime = time.time()
197 print('一共运行了{0}秒'.format((endtime-starttime)))
198
199 if __name__ == '__main__':
200 main()
2、只有TCP通信流量
1 #!/usr/bin/python3
2 #-*- coding:utf-8 -*-
3
4 #编写环境 windows 7 x64 + Notepad++ + Python3.7.0
5
6 '''
7 1、存在TCP通信流量 sip:"10.10.10.10" AND dip:"攻击IP"(有流量)
8 '''
9
10
11 import urllib3
12 urllib3.disable_warnings()
13 import sys
14 import time
15 import datetime
16
17 import requests
18 import xlrd
19 import json
20
21
22
23 #威胁分析平台地址
24 host="https://10.10.10.10"
25
26 headers ={
27 'Accept': 'application/json, text/plain, */*',
28 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/75.0.3770.100 Safari/537.36',
29 'Accept-Encoding': 'gzip, deflate',
30 'Accept-Language': 'zh-CN,zh;q=0.9',
31 'Cookie': 'session=1111111111156654f8005689b64e5afa244073ff1111111111111111',
32 }
33
34 ''' stime etime
35 2020-05-02 00:00:00-2020-05-02 14:27:13 1588348800000 1588400833356
36 2020-05-01 00:00:00-2020-05-01 23:59:59 1588262400000 1588348799999
37 2020-04-30 00:00:00-2020-04-30 23:59:59 1588176000000 1588262399999
38 2020-04-29 00:00:00-2020-04-29 23:59:59 1588089600000 1588175999999
39 2020-04-28 00:00:00-2020-04-28 23:59:59 1588003200000 1588089599999
40 2020-04-27 00:00:00-2020-04-27 23:59:59 1587916800000 1588003199999
41 2020-04-26 00:00:00-2020-04-26 23:59:59 1587830400000 1587916799999
42 '''
43 def tcp(tcp_detail):
44
45 #查询受害IP与C&C服务器通信次数
46 alarm_sip,alarm_dip = tcp_detail
47
48 try:
49 total_week=0
50 etime = int(round(time.time()*1000))
51 for i in range(7):
52
53 ymd = time.strftime('%Y-%m-%d',time.localtime(etime/1000))#%Y-%m-%d %H:%M:%S
54 stime = int(time.mktime(time.strptime(str(ymd),"%Y-%m-%d")))*1000
55
56 url = host +'/xxx/zzz/getsearch?branch_id=232&stime={stime}&etime={etime}&keyword=sip:%22{alarm_sip}%22+AND+dip:%22{alarm_dip}%22&index=xxx-tcpflow&category=xxx&interval=3h&page=1&size=20&asset_group_ids=&mode=advance_model&key_fields=@timestamp&graph_conf=%7B%7D'.format(stime=stime,etime=etime,alarm_sip=alarm_sip,alarm_dip=alarm_dip)
57
58 req = requests.get(url, headers=headers, verify=False).json()
59 #print(req)
60 total_week += req['data']['search']['total']
61 if i==1:
62 total_oneday=total_week
63 etime=stime-1
64
65 if total_week > 30 and total_oneday > 0:
66 return [alarm_sip, alarm_dip,total_week]
67 return [alarm_sip, alarm_dip,False]
68 except Exception as e:
69 print("tcp wrong")
70 print(e)
71 sys.exit()
72
73
74 #取xlsx第5列(受害IP)和第7列(攻击IP)存放到列表中(去重)
75 def get_xlsx_alarm_list():
76
77 try:
78 alarm_list = []
79 print(sys.argv[1])
80
81 #打开Excel文件读取数据
82 data = xlrd.open_workbook(sys.argv[1])
83
84 #获取一个工作表
85 table = data.sheets()[0] #通过索引顺序获取
86
87 #获取行数和列数
88 #nrows = table.nrows
89 #nclos = table.ncols
90
91 #遍历每一行
92 for i in range(1,table.nrows):
93 alarm_list.append((table.row_values(i)[4],table.row_values(i)[6]))
94
95 return list(set(alarm_list))
96 except Exception as e:
97 print(e)
98 print('xlsx wrong')
99 sys.exit()
100
101
102 def login():
103 #https://10.10.10.10/xxx/zzz/view
104 url =host + '/xxx/zzz/view'; #找到返回结果是"未登陆或登陆超时!"的地址(JSON数据)
105
106 #获取威胁分析平台的版本信息
107 #https://10.10.10.10/xxx/zzz/channel_version
108 #xxx_version = requests.get(host + '/xxx/zzz/channel_version',headers=headers,verify=False).json()['data']['license_info']['version']
109 #print(xxx_version)
110
111 try:
112 #用requests来抓取网页里面的json数据
113 req1 =requests.get(url=url,headers=headers,verify=False).json()
114 if req1.get('status') == 200:
115 print('login success')
116 return True
117 else:
118 print(req1.get('message'))
119 return False
120 except Exception as e:
121 print(e)
122
123 def tcp_write():
124
125 alarm_list=get_xlsx_alarm_list()
126 #print(alarm_list)#[sip,dip]
127 for alarm in alarm_list:
128 tcprecord = tcp(alarm)
129 print(tcprecord) #['192.168.216.39', '183.60.159.171', 12]
130 if tcprecord[2]:
131 file = open('tcp.txt','a')
132 file.write(str(tcprecord)+"
")
133
134 def main():
135 print("eg:python3 tcp.py 告警_列表导出2020-01-29 00_00_00至2020-02-04 20_07_59.xlsx")
136 starttime = time.time()
137 if(login()):
138 tcp_write()
139 endtime = time.time()
140 print('一共运行了{0}秒'.format((endtime-starttime)))
141
142 if __name__ == '__main__':
143 main()
3、只有TCP通信流量(多线程版)
1 #!/usr/bin/python3
2 #-*- coding:utf-8 -*-
3
4 #编写环境 windows 7 x64 + Notepad++ + Python3.7.0
5
6 '''
7 1、存在TCP通信流量 sip:"10.10.10.10" AND dip:""(有流量)
8
9 (1)死锁(2)很多线程未被处理,占用资源
10 https://www.cnblogs.com/zengjfgit/p/6259429.html?utm_source=itdadao&utm_medium=referral
11 https://blog.csdn.net/qq_40279964/article/details/82902159
12 http://ixuling.com/?p=187
13 '''
14
15
16 import urllib3
17 urllib3.disable_warnings()
18 import sys
19 import time
20 import datetime
21
22 import requests
23 import xlrd
24 import json
25 import threading
26 import urllib
27
28
29 #威胁分析平台地址
30 host="https://10.10.10.10"
31
32 headers ={
33 'Accept': 'application/json, text/plain, */*',
34 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/75.0.3770.100 Safari/537.36',
35 'Accept-Encoding': 'gzip, deflate',
36 'Accept-Language': 'zh-CN,zh;q=0.9',
37 'Cookie': 'session=111114349434b881f11111111',
38 }
39
40 Lock=threading.Lock()
41
42
43 def tcp(tcp_detail):
44
45
46 #查询受害IP与C&C服务器通信次数
47 alarm_sip,alarm_dip = tcp_detail
48 etime = int(round(time.time()*1000))
49 #stime = etime - (7*24 * 60 * 60 * 1000) # 搜索前7天的TCP流量
50 stime = etime - (24 * 60 * 60 * 1000) # 搜索今天的TCP流量
51
52 url = host +'/xxxx/zzzz/getsearch?branch_id=3232323&stime={stime}&etime={etime}&keyword=sip:%22{alarm_sip}%22+AND+dip:%22{alarm_dip}%22&index=xxxx-tcpflow&category=xxxxx&interval=3h&page=1&size=20&asset_group_ids=&mode=advance_model&key_fields=@timestamp&graph_conf=%7B%7D'.format(stime=stime,etime=etime,alarm_sip=alarm_sip,alarm_dip=alarm_dip)
53
54
55 try:
56
57 req = requests.get(url, headers=headers, verify=False).json()
58 #print(req)
59
60 if req['data']['search']['total'] > 30:
61 return [alarm_sip, alarm_dip,req['data']['search']['total']]
62 return [alarm_sip, alarm_dip,False]
63 except Exception as e:
64 print("tcp wrong")
65 print(e)
66 sys.exit()
67
68
69 #取xlsx第5列(受害IP)和第7列(攻击IP)存放到列表中(去重)
70 def get_xlsx_alarm_list():
71
72 try:
73 alarm_list = []
74 print(sys.argv[1])
75
76 #打开Excel文件读取数据
77 data = xlrd.open_workbook(sys.argv[1])
78
79 #获取一个工作表
80 table = data.sheets()[0] #通过索引顺序获取
81
82 #获取行数和列数
83 #nrows = table.nrows
84 #nclos = table.ncols
85
86 #遍历每一行
87 for i in range(1,table.nrows):
88 alarm_list.append((table.row_values(i)[4],table.row_values(i)[6]))
89
90 return list(set(alarm_list))
91 except Exception as e:
92 print(e)
93 print('xlsx wrong')
94 sys.exit()
95
96 def login():
97 #https://10.10.10.10/xxx/zzz/view
98 url =host + '/xxx/zzzz/view'; #找到返回结果是"未登陆或登陆超时!"的地址(JSON数据)
99
100 #获取威胁分析平台的版本信息
101 #https://10.10.10.10/xxx/zzz/channel_version
102 #xxx_version = requests.get(host + '/xxx/zzz/channel_version',headers=headers,verify=False).json()['data']['license_info']['version']
103 #print(xxx_version)
104
105 try:
106 #用requests来抓取网页里面的json数据
107 req1 =requests.get(url=url,headers=headers,verify=False).json()
108 if req1.get('status') == 200:
109 print('login success')
110 return True
111 else:
112 print(req1.get('message'))
113 return False
114 except Exception as e:
115 print(e)
116
117 def tcp_record(alarm):
118 Lock.acquire()
119 try:
120 tcprecord = tcp(alarm)
121 print(tcprecord) #['192.168.2.3', '283.10.159.111', 12]
122
123 if tcprecord[2]:
124 file = open('thread_tcp.txt','a')
125 file.write(str(tcprecord)+"
")
126 finally:
127 Lock.release()
128
129 def tcp_write():
130 opener = urllib.request.build_opener()
131 # 设置最大线程数
132 thread_max = threading.BoundedSemaphore(300)#这个值适当的调整,注意这里不是越大越好,适合的才是最好的。
133
134 alarm_list=get_xlsx_alarm_list()
135 #print(alarm_list)#[sip,dip]
136
137 threads = []
138 for alarm in alarm_list:
139 # 如果线程达到最大值则等待前面线程跑完空出线程位置
140 thread_max.acquire()
141 t = threading.Thread(target=tcp_record,args=(alarm,))
142 t.start()
143 threads.append(t)
144
145 for t in threads:
146 t.join()
147 # 任务跑完移除线程
148 thread_max.release()
149
150 def main():
151 print("eg:python3 thread_tcp.py 告警_列表导出2020-01-29 00_00_00至2020-02-04 20_07_59.xlsx")
152 starttime = time.time()
153 if(login()):
154 tcp_write()
155 endtime = time.time()
156 print('一共运行了{0}秒'.format((endtime-starttime)))
157
158 if __name__ == '__main__':
159 main()
4、有域名请求
1 #!/usr/bin/python3
2 #-*- coding:utf-8 -*-
3
4 #编写环境 windows 7 x64 Notepad++ + Python3.5.0
5
6 '''
7 1、发现告警
8 2、存在DNS-A记录 dip:"10.10.10.10" AND host:"恶意域名" AND addr:*
9 3、确定恶意域名
10 '''
11
12
13 import urllib3
14 urllib3.disable_warnings()
15 import sys
16 import time
17 import datetime
18
19 import requests
20 import xlrd
21
22
23
24 #威胁分析平台地址
25 host="https://10.10.10.10"
26
27 headers ={
28 'Accept': 'application/json, text/plain, */*',
29 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/75.0.3770.100 Safari/537.36',
30 'Accept-Encoding': 'gzip, deflate',
31 'Accept-Language': 'zh-CN,zh;q=0.9',
32 'Cookie': 'session=3546a6ffe89d43e9966cea7c1797bf2ecfd29f22762a0a72970899a69635a24a61479ccd8290bc30',
33 }
34
35
36 #查询dns-A记录
37 def dns_a(threat_detail):
38 # 获取ioc对应的dns a记录,如果不存在解析记录返回列表包含false,存在解析记录获取全部返回页全部解析ip,去重后返回list
39 #etime结束时间 stime开始时间
40 dns_a_list = []
41 alarm_dip, ioc = threat_detail
42 etime = int(round(time.time() * 1000))
43 #stime = etime - (4 * 60 * 60 * 1000) # 搜索前4小时的dns解析记录
44 stime = etime - (24 * 60 * 60 * 1000) # 搜索今天的dns解析记录
45
46 url = host + '/xxx/zzz/getsearch?stime={stime}&etime={etime}&keyword=dip:%22{alarm_dip}%22+AND+host:%22{ioc}%22+AND+addr:*&index=xxx-dns&category=xxx&interval=3h&page=1&size=20&asset_group_ids=&mode=advance_model&key_fields=@timestamp'.format(stime=stime,etime=etime,alarm_dip=alarm_dip,ioc=ioc)
47 #print(url)
48
49
50
51 try:
52 req = requests.get(url, headers=headers, verify=False).json()
53
54 if req['data']['search']['total'] <= 0:
55 print('can not find dns a record',end = '
',flush = True)
56 return [alarm_dip, ioc , False]
57
58 for d in req['data']['search']['hits']:
59 for k,v in d.items():
60 if k=='_source':
61 for x,y in d[k].items():
62 if x=='addr':
63 #print(d[k][x])
64 dns_a_list.append(d[k][x])
65 #sorted(dns_a_list)
66 print('find dns a record', end='
',flush= True)
67 #去重
68 b=[]
69 for item in dns_a_list:
70 if item not in b:
71 b.append(item)
72 b=b[0]
73 return [alarm_dip, ioc ,b]
74
75
76 except Exception as e:
77 print("dns_a wrong")
78 print(e)
79 sys.exit()
80
81
82 #取xlsx第5列(受害IP)和第9列(域名/IOC)存放到列表中(去重)
83 def get_xlsx_alarm_list():
84
85 try:
86 alarm_list = []
87 print(sys.argv[1])
88
89 #打开Excel文件读取数据
90 data = xlrd.open_workbook(sys.argv[1])
91
92 #获取一个工作表
93 table = data.sheets()[0] #通过索引顺序获取
94
95 #获取行数和列数
96 #nrows = table.nrows
97 #nclos = table.ncols
98
99 #遍历每一行
100 for i in range(1,table.nrows):
101 alarm_list.append((table.row_values(i)[4],table.row_values(i)[9]))
102
103 return list(set(alarm_list))
104 except Exception as e:
105 print(e)
106 print('xlsx wrong')
107 sys.exit()
108
109
110 def login():
111 #https://10.10.10.10/xxx/dashboard/view
112 url =host + '/xxx/dashboard/view'; #找到返回结果是"未登陆或登陆超时!"的地址(JSON数据)
113
114 #获取威胁分析平台的版本信息
115 #https://10.10.10.10/xxx/zzz/channel_version
116 #xxx_version = requests.get(host + '/xxx/zzz/channel_version',headers=headers,verify=False).json()['data']['license_info']['version']
117 #print(xxx_version)
118
119 try:
120 #用requests来抓取网页里面的json数据
121 req1 =requests.get(url=url,headers=headers,verify=False).json()
122 if req1.get('status') == 200:
123 print('login success')
124 return True
125 else:
126 print(req1.get('message'))
127 return False
128 except Exception as e:
129 print(e)
130
131 def dns_write():
132 alarm_list=get_xlsx_alarm_list()
133 #print(alarm_list)#[dip,host]
134
135 for alarm in alarm_list:
136 dnsrecord = dns_a(alarm)
137 print(dnsrecord)
138 if dnsrecord[2]:
139 file = open('dns_a.txt','a')
140 file.write(str(dnsrecord)+"
")
141
142 def main():
143 print("eg:python3 dns_a.py 告警_列表导出2020-01-29 00_00_00至2020-02-04 20_07_59.xlsx")
144 starttime = time.time()
145 if(login()):
146 dns_write()
147 endtime = time.time()
148 print('一共运行了{0}秒'.format((endtime-starttime)))
149
150 if __name__ == '__main__':
151 main()
152