在开发中经常会有这样的需求:
Activity A启动Activity B,在B中输入一个数据或者选择一个后 将结果返回给A 。要实现该功能也很简单可以使用startActivityForResult来实现。
代码贴上:
在A 中:
-
package com.example.helloworld2; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.TextView; public class A_Activity extends Activity { private TextView text; private final static int REQUESTCODE = 1; private final static int RESULTCODE = 2; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); text = (TextView) findViewById(R.id.text); } public void go(View v){ Intent intent = new Intent(A_Activity.this,B_Activity.class); startActivityForResult(intent, REQUESTCODE); } @Override protected void onActivityResult(int requsetCode, int resultCode, Intent intent) { // TODO Auto-generated method stub super.onActivityResult(requsetCode, resultCode, intent); if(resultCode==RESULTCODE){ Bundle bundle = intent.getExtras(); String result = bundle.getString("result"); text.setText("返回的值:"+result+""); } } }
在B中:
-
public class B_Activity extends Activity { private EditText text2; protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_b); text2 = (EditText) findViewById(R.id.text2); } /** * 返回 * @param v */ public void go(View v){ Intent intent = new Intent(); intent.putExtra("result", text2.getText().toString().trim()); setResult(2, intent); //将结果出给A finish();//此处一定要调用finish()方法 } }
布局文件:
A:
-
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="com.example.helloworld.MainActivity" > <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:onClick="go" android:text="跳转"/> <TextView android:id="@+id/text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/hello_world" /> </LinearLayout>
B:
-
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context="com.example.helloworld.MainActivity" > <Button android:layout_width="match_parent" android:layout_height="wrap_content" android:onClick="go" android:text="返回"/> <EditText android:id="@+id/text2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="100" /> </LinearLayout>
在AndroidManifest.xml文件中注册activity
<application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".A_Activity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name="com.example.helloworld2.B_Activity" > </activity> </application>
好了,到此为止,从A跳转B,再从B将结果回传个A的功能似乎是实现。嘎嘎,高兴一下。
A界面
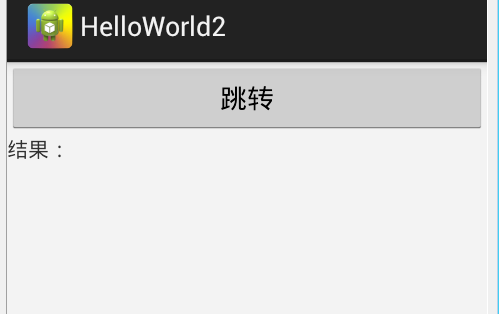
跳转B
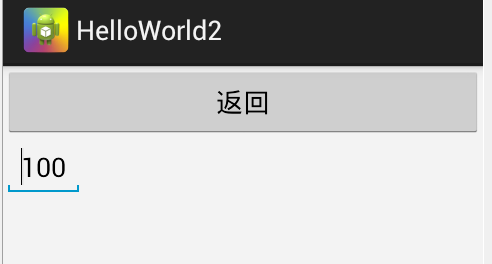
从B返回A
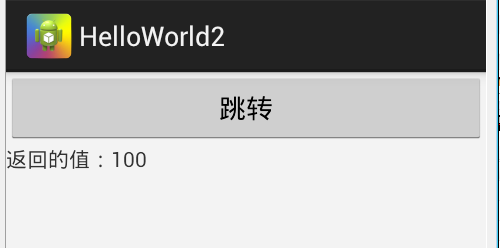
xx天后,又遇到类似的需求,心想这还不简单,照着上面很快实现,跑起来一看,结果 从B返回A如下图,并没有将至传回到A,
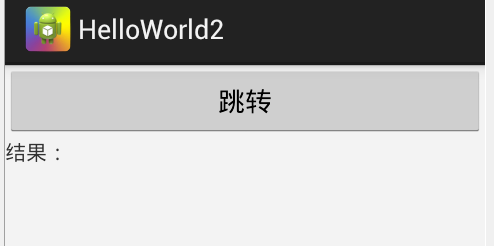
奇怪,好吧,出现问题啦,只好一步步调试了,通过在A中输出log跟踪发现,从B中返回到A的时候并没有调用onActivityResult方法,这又是为什么呢,终于在网上找到一篇文章http://www.codes51.com/article/detail_127761.html 上面讲了onActivityResult 与Activity的启动模式有关,一看自己的程序,果然我将程序中所有的Activity全部设置了singleTask模式,经过试验如果要通过onActivityResult回传值似乎需要将B设为standard模式或者singleTop模式才能实现,就这样将程序bug解决,尽管这种解决方案好像不太优雅。
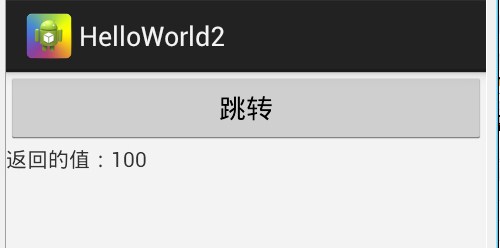
至此onActivityResult的使用基本已经完成啦。
又过了XXX天,公司给配了一个android 5.0.2版本的手机,将之前的项目在新手机上面跑了下,突然发现,之前A,B设置为singleTask并没有出现onActivityResult 不被调用的情况,这个应该是Android 5.0系统与之前版本对区别之一吧,具体原因还有待研究,同时也希望高手指点,为什么会出现这种情况。