While Mike was walking in the subway, all the stuff in his back-bag dropped on the ground. There were several fax messages among them. He concatenated these strings in some order and now he has string s.
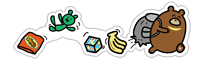
He is not sure if this is his own back-bag or someone else's. He remembered that there were exactly k messages in his own bag, each was a palindrome string and all those strings had the same length.
He asked you to help him and tell him if he has worn his own back-bag. Check if the given string s is a concatenation of k palindromes of the same length.
The first line of input contains string s containing lowercase English letters (1 ≤ |s| ≤ 1000).
The second line contains integer k (1 ≤ k ≤ 1000).
Print "YES"(without quotes) if he has worn his own back-bag or "NO"(without quotes) otherwise.
saba
2
NO
saddastavvat
2
YES
Palindrome is a string reading the same forward and backward.
In the second sample, the faxes in his back-bag can be "saddas" and "tavvat".
题目思路:看串是否能被 k 整除,如果能,在每段里判断这段是否是回文串。

#include <cstring> #include <cstdio> #include <algorithm> #include <iostream> using namespace std; int main() { int k; char str[1005]; char s1[1005]; gets(str); scanf("%d", &k); int len = strlen(str); bool flag = false; if(len % k == 0) { int l = len / k; for(int i = 0; i != len && !flag; i += l) { for(int j = 0; j != l && !flag; ++j) { if(str[i+j] != str[i+l-1-j]) { flag = true; } } } if(flag == true) { cout << "NO" << endl; } else { cout << "YES" << endl; } } else { cout << "NO" << endl; } return 0; }
Mike and some bears are playing a game just for fun. Mike is the judge. All bears except Mike are standing in an n × m grid, there's exactly one bear in each cell. We denote the bear standing in column number j of row number i by (i, j). Mike's hands are on his ears (since he's the judge) and each bear standing in the grid has hands either on his mouth or his eyes.
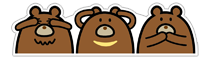
They play for q rounds. In each round, Mike chooses a bear (i, j) and tells him to change his state i. e. if his hands are on his mouth, then he'll put his hands on his eyes or he'll put his hands on his mouth otherwise. After that, Mike wants to know the score of the bears.
Score of the bears is the maximum over all rows of number of consecutive bears with hands on their eyes in that row.
Since bears are lazy, Mike asked you for help. For each round, tell him the score of these bears after changing the state of a bear selected in that round.
The first line of input contains three integers n, m and q (1 ≤ n, m ≤ 500 and 1 ≤ q ≤ 5000).
The next n lines contain the grid description. There are m integers separated by spaces in each line. Each of these numbers is either 0 (for mouth) or 1 (for eyes).
The next q lines contain the information about the rounds. Each of them contains two integers i and j (1 ≤ i ≤ n and 1 ≤ j ≤ m), the row number and the column number of the bear changing his state.
After each round, print the current score of the bears.
5 4 5
0 1 1 0
1 0 0 1
0 1 1 0
1 0 0 1
0 0 0 0
1 1
1 4
1 1
4 2
4 3
3
4
3
3
4
思路:给你个图,统计每行中,最多有几个连续的“1”,然后再在没行里找个最大的。 注意不能再具体查询的时候再对图进行暴力,复杂度太高。

#include <cstdio> #include <iostream> #include <cstring> #include <algorithm> using namespace std; int arr[510][510]; int sum[501][501]; int ans[501]; int main() { int n, m, q; cin >> n >> m >> q; memset(sum, 0, sizeof(sum)); memset(ans, 0, sizeof(ans)); for(int i = 0; i != n; ++i) { scanf("%d", &arr[i][0]); sum[i][0] = (arr[i][0] == 1) ? 1 : 0; for(int j = 1; j != m; ++j) { scanf("%d", &arr[i][j]); if(arr[i][j]) sum[i][j] = sum[i][j-1] + 1; else sum[i][j] = 0; } for(int j = 0; j != m; ++j){ ans[i] = max(ans[i], sum[i][j]); } } int x, y, Max = 0; while(q--) { scanf("%d %d", &x, &y); arr[x-1][y-1] = arr[x-1][y-1] == 0 ? 1 : 0; sum[x-1][0] = (arr[x-1][0] == 1) ? 1 : 0; for(int i = 1; i != m; ++i) { if(arr[x-1][i]) sum[x-1][i] = sum[x-1][i-1] + 1; else sum[x-1][i] = 0; } ans[x-1] = 0; for(int i = 0; i != m; ++i) { ans[x-1] = max(ans[x-1], sum[x-1][i]); } for(int i = 0; i != n; ++i) { Max = max(Max, ans[i]); } printf("%d ", Max); Max = 0; } return 0; }