1 package javatestywh; 2 3 public class ScoreInformation 4 { 5 private String stunumber; 6 private String name; 7 private double mathematicsscore=4; 8 private double englishscore=3; 9 private double networkscore=4; 10 private double databasescore=3; 11 private double softwarescore=2; 12 public ScoreInformation() 13 { 14 super(); 15 16 } 17 public ScoreInformation(String stunumber, String name, double mathematicsscore, double englishscore, 18 double networkscore, double databasescore, double softwarescore) 19 { 20 super(); 21 this.stunumber = stunumber; 22 this.name = name; 23 this.mathematicsscore = mathematicsscore; 24 this.englishscore = englishscore; 25 this.networkscore = networkscore; 26 this.databasescore = databasescore; 27 this.softwarescore = softwarescore; 28 } 29 public String getStunumber() 30 { 31 return stunumber; 32 } 33 public void setStunumber(String stunumber) 34 { 35 this.stunumber = stunumber; 36 } 37 public String getName() 38 { 39 return name; 40 } 41 public void setName(String name) 42 { 43 this.name = name; 44 } 45 public double getMathematicsscore() 46 { 47 return mathematicsscore; 48 } 49 public void setMathematicsscore(double mathematicsscore) 50 { 51 this.mathematicsscore = mathematicsscore; 52 } 53 public double getEnglishscore() 54 { 55 return englishscore; 56 } 57 public void setEnglishscore(double englishscore) 58 { 59 this.englishscore = englishscore; 60 } 61 public double getNetworkscore() 62 { 63 return networkscore; 64 } 65 public void setNetworkscore(double networkscore) 66 { 67 this.networkscore = networkscore; 68 } 69 public double getDatabasescore() 70 { 71 return databasescore; 72 } 73 public void setDatabasescore(double databasescore) 74 { 75 this.databasescore = databasescore; 76 } 77 public double getSoftwarescore() 78 { 79 return softwarescore; 80 } 81 public void setSoftwarescore(double softwarescore) 82 { 83 this.softwarescore = softwarescore; 84 } 85 @Override 86 public String toString() 87 { 88 return "学号" + stunumber + ", 姓名=" + name + ", 数学=" + mathematicsscore 89 + ", 英语=" + englishscore + ", 网络=" + networkscore + ", 数据库=" 90 + databasescore + ", 软件=" + softwarescore ; 91 } 92 93 94 95 96 } 97 package javatestywh; 98 99 import java.util.Scanner; 100 101 public class scoreManagement 102 { 103 public static void main(String[] args) 104 { 105 Scanner sc = new Scanner(System.in); 106 // 定义数组预存五个学生的姓名以及学号; 107 ScoreInformation[] stu = new ScoreInformation[5]; 108 stu[0] = new ScoreInformation("20183606", "yanwenhui", 4, 3, 4, 3, 2); 109 stu[1] = new ScoreInformation("20183610", "fengfeng", 4, 3, 4, 3, 2); 110 stu[2] = new ScoreInformation("20183607", "heihei", 4, 3, 4, 3, 2); 111 stu[3] = new ScoreInformation("20183608", "fengshun", 4, 3, 4, 3, 2); 112 stu[4] = new ScoreInformation("20183609", "beauty", 4, 3, 4, 3, 2); 113 mainshow(stu); 114 } 115 116 // 主界面 117 public static void mainshow(ScoreInformation[] stu) 118 { 119 System.out.print("*********************************************************** " + "石家庄铁道大学软件工程系 " 120 + "学生学籍管理系统 2019 版 " + "*********************************************************** " 121 + "1、 学生考试成绩录入 " + "2、 学生考试成绩修改 " + "3、 计算学生成绩绩点 " + "4、退出学籍管理系统 " 122 + "**********************************************************"); 123 Scanner sc = new Scanner(System.in);// 输入选项 124 int number = sc.nextInt(); 125 switch (number) 126 { 127 case 1: 128 getgrade(stu); 129 break; 130 case 2: 131 change(stu); 132 break; 133 case 3: 134 calculate(stu); 135 break; 136 case 4: 137 exited(); 138 break; 139 case 5: 140 showgrade(stu); 141 break; 142 default: 143 { 144 System.out.println("信息错误,该选项不存在"); 145 mainshow(stu); 146 break; 147 } 148 149 } 150 151 152 } 153 154 public static void getgrade(ScoreInformation[] stu) 155 { 156 Scanner sc = new Scanner(System.in); 157 System.out.print( 158 "*********************************************************** " + "石家庄铁道大学软件工程系学生学籍管理系统 2019 版 " 159 + "学生考试成绩录入 " + "*********************************************************** " 160 + "请输入学生学号:XXXXXXXX " + "**********************************************************"); 161 String number = sc.nextLine(); 162 int flag = 0; 163 for (int i = 0; i < 5; i++) 164 { 165 if (stu[i].getStunumber().equals(number)) 166 { 167 System.out.print("*********************************************************** " 168 + "std软件工程系学生学籍管理系统 2019 版 " + "学生考试成绩录入界面 " 169 + "*********************************************************** " + "学生学号:" 170 + stu[i].getStunumber() + " " + "学生姓名:" + stu[i].getName() + " " + "请输入高等数学成绩:XXX " 171 + "**********************************************************"); 172 double math = sc.nextDouble(); 173 System.out.println(stu[i].getName() + "的高数成绩是:" + math); 174 System.out.println("请输入该生的英语成绩"); 175 double english = sc.nextDouble(); 176 System.out.println(stu[i].getName() + "的英语成绩是:" + english); 177 System.out.println("请输入该生的网络成绩"); 178 double network = sc.nextDouble(); 179 System.out.println(stu[i].getName() + "的网络成绩是:" + network); 180 System.out.println("请输入该生的数据库成绩"); 181 double data = sc.nextDouble(); 182 System.out.println(stu[i].getName() + "的网络成绩是:" + data); 183 System.out.println("请输入该生的软件成绩"); 184 double soft = sc.nextDouble(); 185 System.out.print("*********************************************************** " 186 + "std软件工程系学生学籍管理系统 2019 版 " + "学生考试成绩录入 " 187 + "*********************************************************** " + "学生学号:" 188 + stu[i].getStunumber() + " " + "学生姓名:" + stu[i].getName() + " " + "高等数学成绩:" + math 189 + " " + "大学英语成绩:" + english + " " + "计算机网络成绩:" + network + " " + "数据库成绩:" + data 190 + " " + "软件工程成绩:" + soft + " " + "该学生成绩已录入完毕,是否提交(Y/N) " 191 + "**********************************************************"); 192 193 if (sc.next().equals("Y")) 194 { 195 stu[i].setEnglishscore(english); 196 stu[i].setMathematicsscore(math); 197 stu[i].setDatabasescore(data); 198 stu[i].setNetworkscore(network); 199 stu[i].setSoftwarescore(soft); 200 mainshow(stu); 201 } else 202 { 203 System.out.println("返回录入"); 204 getgrade(stu); 205 } 206 207 flag = 1; 208 } 209 } 210 // 如果没有该学生信息,提示重新输入; 211 if (flag == 0) 212 { 213 System.out.println("该学号不存在,请重新输入"); 214 getgrade(stu); 215 } 216 217 } 218 219 public static void change(ScoreInformation[] stu) 220 { 221 Scanner sc = new Scanner(System.in); 222 223 System.out.print( 224 "*********************************************************** " + "std软件工程系学生学籍管理系统 2019 版 " 225 + "学生考试成绩修改界面 " + "*********************************************************** " 226 + "请输入学生学号:XXXXXXXX " + "**********************************************************"); 227 String number = sc.nextLine(); 228 int flag = 0; 229 for (int i = 0; i < 5; i++) 230 { 231 if (stu[i].getStunumber().equals(number)) 232 { 233 System.out.print("*********************************************************** " 234 + "std软件工程系学生学籍管理系统 2019 版 " + "学生考试成绩录入 " 235 + "*********************************************************** " + "学生学号:" 236 + stu[i].getStunumber() + " " + "学生姓名:" + stu[i].getName() + " " + "1、高等数学成绩:" 237 + stu[i].getMathematicsscore() + " " + "2、大学英语成绩:" + stu[i].getEnglishscore() + " " 238 + "3、计算机网络成绩:" + stu[i].getNetworkscore() + " " + "4、数据库成绩:" + stu[i].getDatabasescore() 239 + " " + "5、软件工程成绩:" + stu[i].getSoftwarescore() + " " 240 +"请输入要修改的选项"+" " 241 + "**********************************************************"); 242 int select = sc.nextInt(); 243 double changed; 244 switch (select) 245 { 246 case 1: 247 System.out.print("学生考试成绩录入界面 " + 248 "*********************************************************** " + 249 "学生学号:"+stu[i].getStunumber()+" " + 250 "学生姓名:"+stu[i].getName()+" " + 251 "请输入修改后高数成绩:XXX " + 252 "**********************************************************"); 253 changed=sc.nextDouble(); 254 System.out.print("*********************************************************** " 255 + "std软件工程系学生学籍管理系统 2019 版 " + "学生考试成绩录入 " 256 + "*********************************************************** " + "学生学号:" 257 + stu[i].getStunumber() + " " + "学生姓名:" + stu[i].getName() + " " + "1、高等数学成绩:" 258 + changed + " " + "2、大学英语成绩:" + stu[i].getEnglishscore() + " " 259 + "3、计算机网络成绩:" + stu[i].getNetworkscore() + " " + "4、数据库成绩:" + stu[i].getDatabasescore() 260 + " " + "5、软件工程成绩:" + stu[i].getSoftwarescore() + " " 261 +"该学生成绩已修改完毕,是否提交(Y/N)"+" " 262 + "**********************************************************"); 263 if(sc.next().equals("Y")) { 264 stu[i].setMathematicsscore(changed); 265 mainshow(stu); 266 } 267 else { 268 change(stu); 269 } 270 break; 271 case 2: 272 System.out.print("学生考试成绩录入界面 " + 273 "*********************************************************** " + 274 "学生学号:"+stu[i].getStunumber()+" " + 275 "学生姓名:"+stu[i].getName()+" " + 276 "请输入修改后成绩:英语 " + 277 "**********************************************************"); 278 changed=sc.nextDouble(); 279 System.out.print("*********************************************************** " 280 + "std软件工程系学生学籍管理系统 2019 版 " + "学生考试成绩录入 " 281 + "*********************************************************** " + "学生学号:" 282 + stu[i].getStunumber() + " " + "学生姓名:" + stu[i].getName() + " " + "1、高等数学成绩:" 283 + stu[i].getMathematicsscore() + " " + "2、大学英语成绩:" + changed + " " 284 + "3、计算机网络成绩:" + stu[i].getNetworkscore() + " " + "4、数据库成绩:" + stu[i].getDatabasescore() 285 + " " + "5、软件工程成绩:" + stu[i].getSoftwarescore() + " " 286 +"该学生成绩已修改完毕,是否提交(Y/N)"+" " 287 + "**********************************************************"); 288 if(sc.next().equals("Y")) { 289 stu[i].setEnglishscore(changed); 290 mainshow(stu); 291 } 292 else { 293 change(stu); 294 } 295 break; 296 case 3: 297 System.out.print("学生考试成绩录入界面 " + 298 "*********************************************************** " + 299 "学生学号:"+stu[i].getStunumber()+" " + 300 "学生姓名:"+stu[i].getName()+" " + 301 "请输入修改后网络成绩: " + 302 "**********************************************************"); 303 changed=sc.nextDouble(); 304 System.out.print("*********************************************************** " 305 + "std软件工程系学生学籍管理系统 2019 版 " + "学生考试成绩录入 " 306 + "*********************************************************** " + "学生学号:" 307 + stu[i].getStunumber() + " " + "学生姓名:" + stu[i].getName() + " " + "1、高等数学成绩:" 308 + stu[i].getMathematicsscore() + " " + "2、大学英语成绩:" + stu[i].getEnglishscore() + " " 309 + "3、计算机网络成绩:" + changed + " " + "4、数据库成绩:" + stu[i].getDatabasescore() 310 + " " + "5、软件工程成绩:" + stu[i].getSoftwarescore() + " " 311 +"该学生成绩已修改完毕,是否提交(Y/N)"+" " 312 + "**********************************************************"); 313 if(sc.next().equals("Y")) { 314 stu[i].setNetworkscore(changed); 315 mainshow(stu); 316 } 317 else { 318 change(stu); 319 } 320 break; 321 case 4: 322 System.out.print("学生考试成绩录入界面 " + 323 "*********************************************************** " + 324 "学生学号:"+stu[i].getStunumber()+" " + 325 "学生姓名:"+stu[i].getName()+" " + 326 "请输入修改后数据库成绩:XXX " + 327 "**********************************************************"); 328 changed=sc.nextDouble(); 329 System.out.print("*********************************************************** " 330 + "std软件工程系学生学籍管理系统 2019 版 " + "学生考试成绩录入 " 331 + "*********************************************************** " + "学生学号:" 332 + stu[i].getStunumber() + " " + "学生姓名:" + stu[i].getName() + " " + "1、高等数学成绩:" 333 + stu[i].getMathematicsscore() + " " + "2、大学英语成绩:" + stu[i].getEnglishscore() + " " 334 + "3、计算机网络成绩:" + stu[i].getNetworkscore() + " " + "4、数据库成绩:" + changed 335 + " " + "5、软件工程成绩:" + stu[i].getSoftwarescore() + " " 336 +"该学生成绩已修改完毕,是否提交(Y/N)"+" " 337 + "**********************************************************"); 338 if(sc.next().equals("Y")) { 339 stu[i].setDatabasescore(changed); 340 mainshow(stu); 341 } 342 else { 343 change(stu); 344 } 345 break; 346 case 5: 347 System.out.print("学生考试成绩录入界面 " + 348 "*********************************************************** " + 349 "学生学号:"+stu[i].getStunumber()+" " + 350 "学生姓名:"+stu[i].getName()+" " + 351 "请输入修改后软件成绩:XXX " + 352 "**********************************************************"); 353 changed=sc.nextDouble(); 354 System.out.print("*********************************************************** " 355 + "std软件工程系学生学籍管理系统 2019 版 " + "学生考试成绩录入 " 356 + "*********************************************************** " + "学生学号:" 357 + stu[i].getStunumber() + " " + "学生姓名:" + stu[i].getName() + " " + "1、高等数学成绩:" 358 + stu[i].getMathematicsscore() + " " + "2、大学英语成绩:" + stu[i].getEnglishscore() + " " 359 + "3、计算机网络成绩:" + stu[i].getNetworkscore() + " " + "4、数据库成绩:" + stu[i].getDatabasescore() 360 + " " + "5、软件工程成绩:" + changed + " " 361 +"该学生成绩已修改完毕,是否提交(Y/N)"+" " 362 + "**********************************************************"); 363 if(sc.next().equals("Y")) { 364 stu[i].setSoftwarescore(changed); 365 mainshow(stu); 366 } 367 else { 368 change(stu); 369 } 370 break; 371 default: 372 System.out.println("请选择正确信息输入"); 373 change(stu); 374 break; 375 376 } 377 378 379 flag = 1; 380 } 381 382 } 383 if (flag == 0) 384 { 385 System.out.println("学号输入错误,请重新输入学号"); 386 change(stu); 387 } 388 389 } 390 391 public static void calculate(ScoreInformation[] stu) 392 393 { 394 Scanner sc=new Scanner(System.in); 395 System.out.print("*********************************************************** " + 396 "std软件工程系学生学籍管理系统 2019 版 " + 397 "学生考试成绩绩点计算界面 " + 398 "*********************************************************** " + 399 "请输入学生学号:XXXXXXXX " + 400 "**********************************************************"); 401 String number = sc.nextLine(); 402 float math; 403 float english; 404 float net; 405 float data; 406 float soft; 407 int flag = 0; 408 for (int i = 0; i < 5; i++) 409 { 410 if (stu[i].getStunumber().equals(number)) 411 { 412 double pingjun; 413 pingjun=(judge(stu[i].getMathematicsscore())*4+judge(stu[i].getEnglishscore())*3+ 414 judge(stu[i].getNetworkscore())*4+judge(stu[i].getDatabasescore())*3+judge(stu[i].getSoftwarescore())*2) 415 /16; 416 System.out.print("学生学号:"+stu[i].getStunumber()+" " + 417 "学生姓名:"+stu[i].getName()+" " + 418 "1、高等数学成绩绩点:"+judge(stu[i].getMathematicsscore())+" " + 419 "2、大学英语成绩绩点:"+judge(stu[i].getEnglishscore())+" " + 420 "3、计算机网络成绩绩点:"+judge(stu[i].getNetworkscore())+" " + 421 "4、数据库成绩绩点:"+judge(stu[i].getDatabasescore())+" " + 422 "5、软件工程成绩绩点:"+judge(stu[i].getSoftwarescore())+" " ); 423 System.out.println("你的平均学分绩点为:"+String.format("%.2f", pingjun)+" " ); 424 if(pingjun>=2) { 425 System.out.println("提示信息:您的学分绩点已达到毕业要求"); 426 System.out.println("是否返回主界面:Y/N"); 427 if(sc.next().equals("Y")) { 428 mainshow(stu); 429 } 430 } 431 else { 432 System.out.println("提示信息:您的学分绩点未达到毕业要求"); 433 System.out.println("是否返回主界面:Y/N"); 434 if(sc.next().equals("Y")) { 435 mainshow(stu); 436 } 437 } 438 439 flag=1; 440 } 441 } 442 if(flag==0) { 443 System.out.println("输入信息错误,请重新输入"); 444 mainshow(stu); 445 } 446 } 447 448 public static void exited() 449 450 { 451 System.out.print("*********************************************************** " + 452 "谢谢使用石家庄铁道大学软件工程系学生学籍管理系统 2019 版 " + 453 "制作人:yanwenhui " + 454 "***********************************************************"); 455 456 } 457 public static void showgrade(ScoreInformation [] stu) { 458 for(int i=0;i<stu.length;i++) { 459 System.out.println(stu[i]); 460 } 461 mainshow(stu); 462 463 } 464 465 public static double judge(double x) { 466 double t=0; 467 if(x>=90) t=4; 468 else if(x>=85&&x<=89.9) t=3.7; 469 else if(x>=82&&x<=84.9) t=3.3; 470 else if(x>=78&&x<=81.9) t=3.0; 471 else if(x>=75&&x<=77.9) t=2.3; 472 else if(x>=72&&x<=74.9) t=2.3; 473 else if(x>=68&&x<=71.9) t=2.0; 474 else if(x>=66&&x<=67.9) t=1.7; 475 else if(x>=64&&x<=65.9) t=1.5; 476 else if(x>=60&&x<=63.9) t=1.0; 477 else t=0; 478 479 return t; 480 } 481 }
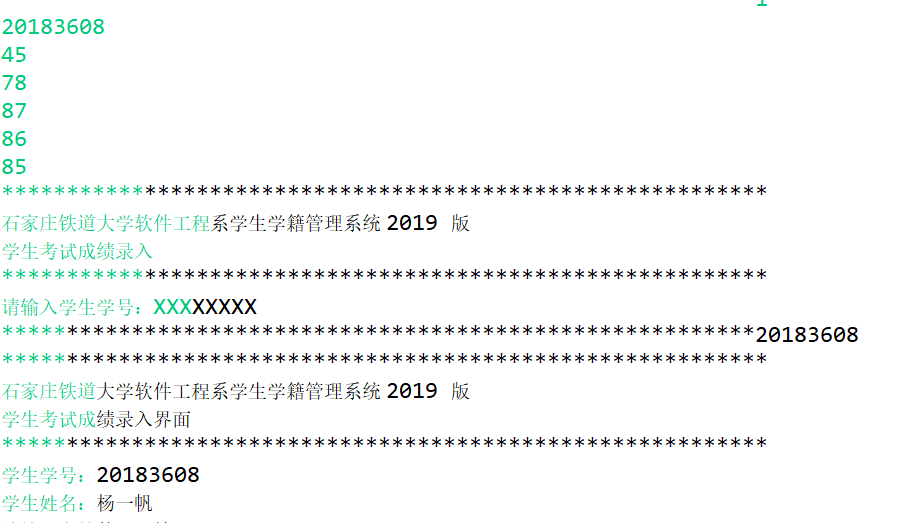
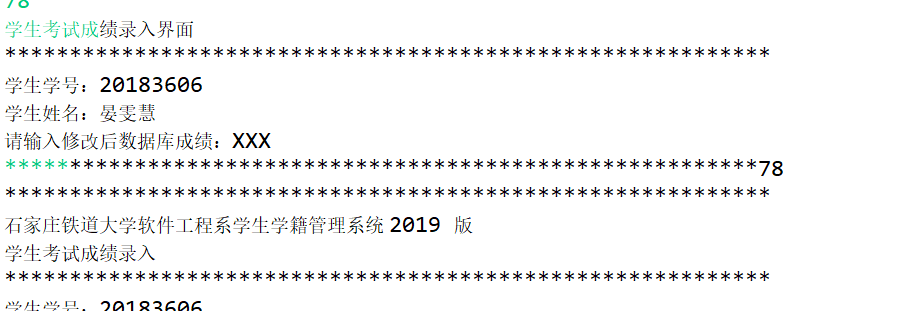
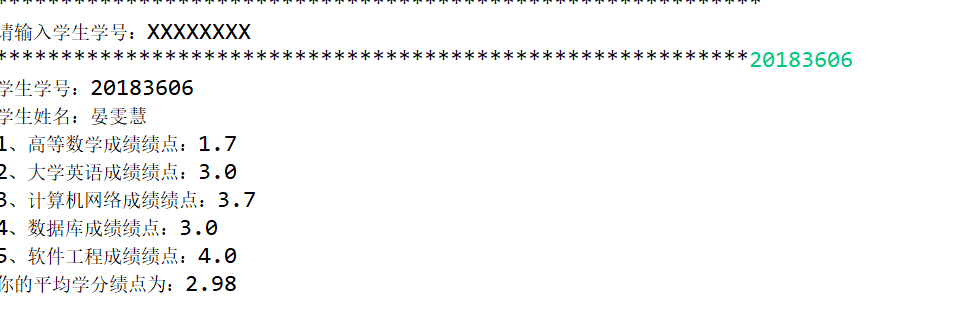

心得:第一次完成的一个系统,哈哈哈;