206.逆转链表
Input: 1->2->3->4->5->NULL Output: 5->4->3->2->1->NULL
方法一:迭代

# Definition for singly-linked list. # class ListNode: # def __init__(self, x): # self.val = x # self.next = None class Solution: def reverseList(self, head: ListNode) -> ListNode: pre, cur = None, head while cur: pre, cur, cur.next = cur, cur.next, pre return pre
方法二:递归

# Definition for singly-linked list. # class ListNode: # def __init__(self, x): # self.val = x # self.next = None class Solution: def reverseList(self, head: ListNode) -> ListNode: if not head or not head.next: return head p = self.reverseList(head.next) head.next.next = head head.next=None return p
24.两两交换链表
Given1->2->3->4
, you should return the list as2->1->4->3
.

# Definition for singly-linked list. # class ListNode: # def __init__(self, x): # self.val = x # self.next = None class Solution: def swapPairs(self, head: ListNode) -> ListNode: pre,pre.next = self,head while pre.next and pre.next.next: a = pre.next b = a.next a,b.next,b = b,a,b.next pre = a return self.next
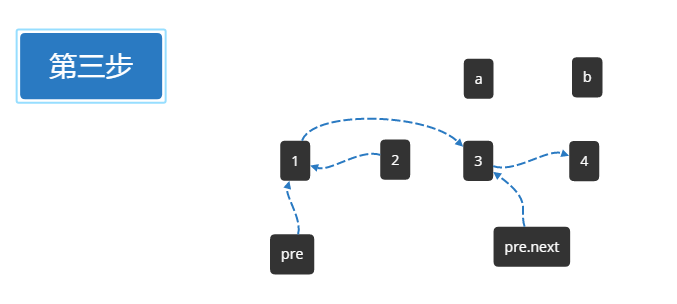
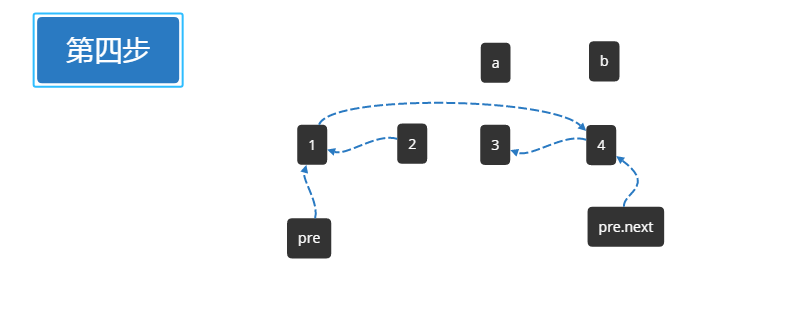
141.回环链表
Input: head = [3,2,0,-4], pos = 1 Output: true Explanation: There is a cycle in the linked list, where tail connects to the second node.
方法一:设置时间暴力循环
方法二:判断是否添加过该元素

# Definition for singly-linked list. # class ListNode: # def __init__(self, x): # self.val = x # self.next = None class Solution: def hasCycle(self, head: ListNode) -> bool: l = [] while head != None: if head.val in l: return True l.append(head) head=head.next return False
方法三:双步运行

# Definition for singly-linked list. # class ListNode: # def __init__(self, x): # self.val = x # self.next = None class Solution: def hasCycle(self, head: ListNode) -> bool: fir = sec =head while fir and sec and sec.next: fir = fir.next sec = sec.next.next if fir == sec: return True return False
25. Reverse Nodes in k-Group
Given this linked list: 1->2->3->4->5
For k = 2, you should return: 2->1->4->3->5
For k = 3, you should return: 3->2->1->4->5

# Definition for singly-linked list. # class ListNode: # def __init__(self, x): # self.val = x # self.next = None class Solution: def reverseKGroup(self, head: ListNode, k: int) -> ListNode: if head: node = head for _ in range(k-1): node = node.next if not node: return head prev = self.reverseKGroup(node.next,k) while prev != node: prev, head.next, head = head, prev, head.next return prev