During the breaks between competitions, top-model Izabella tries to develop herself and not to be bored. For example, now she tries to solve Rubik's cube 2x2x2.
It's too hard to learn to solve Rubik's cube instantly, so she learns to understand if it's possible to solve the cube in some state using 90-degrees rotation of one face of the cube in any direction.
To check her answers she wants to use a program which will for some state of cube tell if it's possible to solve it using one rotation, described above.
Cube is called solved if for each face of cube all squares on it has the same color.
In first line given a sequence of 24 integers ai (1 ≤ ai ≤ 6), where ai denotes color of i-th square. There are exactly 4 occurrences of all colors in this sequence.
Print «YES» (without quotes) if it's possible to solve cube using one rotation and «NO» (without quotes) otherwise.
2 5 4 6 1 3 6 2 5 5 1 2 3 5 3 1 1 2 4 6 6 4 3 4
NO
5 3 5 3 2 5 2 5 6 2 6 2 4 4 4 4 1 1 1 1 6 3 6 3
YES
In first test case cube looks like this:

In second test case cube looks like this:
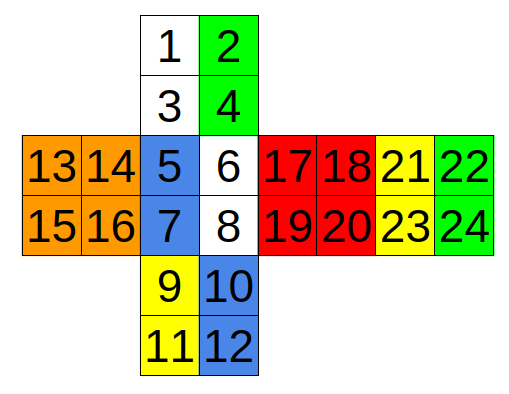
It's possible to solve cube by rotating face with squares with numbers 13, 14, 15, 16.
题意:每组数据 24 个数字,第 i 个数字 表示 编号为 i 的方格的颜色 , 问魔方能不能通过 转动 90度 来得到 所有面颜色都相等的 魔方
思路:要想转动 90 度 得到目标魔方 , 则 必有 两个相对的 面的四个块的颜色是一样的 , 其他的面 每个面只能有 两种颜色 。
则 有 三对 面的 颜色可以使一样的 , 另外 每种情况 向不同方向转动会有 两种情况 , 总共 6 种情况

#include <cstdio> #include <iostream> #include <algorithm> #include <cstring> using namespace std; int a[25]; bool ok1() { if(a[13]==a[14]&&a[14]==a[15]&&a[15]==a[16]&&a[17]==a[18]&&a[18]==a[19]&&a[19]==a[20]) { if(a[21]==a[23]&&a[23]==a[9]&&a[9]==a[11]&&a[22]==a[24]&&a[24]==a[2]&&a[2]==a[4]) { if(a[1]==a[3]&&a[3]==a[6]&&a[6]==a[8]&&a[5]==a[7]&&a[7]==a[10]&&a[10]==a[12]) { return true; } } } return false; } bool ok2() { if(a[13]==a[14]&&a[14]==a[15]&&a[15]==a[16]&&a[17]==a[18]&&a[18]==a[19]&&a[19]==a[20]) { if(a[6]==a[8]&&a[8]==a[9]&&a[9]==a[11]&&a[10]==a[12]&&a[12]==a[22]&&a[22]==a[24]) { if(a[21]==a[23]&&a[23]==a[1]&&a[1]==a[3]&&a[2]==a[4]&&a[4]==a[5]&&a[5]==a[7]) { return true; } } } return false; } bool ok3() { if(a[1]==a[2]&&a[2]==a[3]&&a[3]==a[4]&&a[9]==a[10]&&a[10]==a[11]&&a[11]==a[12]) { if(a[13]==a[14]&&a[14]==a[7]&&a[7]==a[8]&&a[5]==a[6]&&a[6]==a[19]&&a[19]==a[20]) { if(a[17]==a[18]&&a[18]==a[23]&&a[23]==a[24]&&a[21]==a[22]&&a[22]==a[15]&&a[15]==a[16]) { return true; } } } return false; } bool ok4() { if(a[1]==a[2]&&a[2]==a[3]&&a[3]==a[4]&&a[9]==a[10]&&a[10]==a[11]&&a[11]==a[12]) { if(a[13]==a[14]&&a[14]==a[23]&&a[23]==a[24]&&a[5]==a[6]&&a[6]==a[15]&&a[15]==a[16]) { if(a[17]==a[18]&&a[18]==a[7]&&a[7]==a[8]&&a[21]==a[22]&&a[22]==a[19]&&a[19]==a[20]) { return true; } } } return false; } bool ok5() { if(a[5]==a[6]&&a[6]==a[7]&&a[7]==a[8]&&a[21]==a[22]&&a[22]==a[23]&&a[23]==a[24]) { if(a[1]==a[2]&&a[2]==a[17]&&a[17]==a[19]&&a[18]==a[20]&&a[20]==a[9]&&a[9]==a[10]) { if(a[11]==a[12]&&a[12]==a[14]&&a[14]==a[16]&&a[13]==a[15]&&a[15]==a[3]&&a[3]==a[4]) { return true; } } } return false; } bool ok6() { if(a[5]==a[6]&&a[6]==a[7]&&a[7]==a[8]&&a[21]==a[22]&&a[22]==a[23]&&a[23]==a[24]) { if(a[1]==a[2]&&a[2]==a[14]&&a[14]==a[16]&&a[18]==a[20]&&a[20]==a[3]&&a[3]==a[4]) { if(a[11]==a[12]&&a[12]==a[17]&&a[17]==a[19]&&a[13]==a[15]&&a[15]==a[9]&&a[9]==a[10]) { return true; } } } return false; } bool ok() { if(ok1() || ok2() || ok3() || ok4() || ok5() || ok6()) return true; return false; } int main() { for(int i = 1; i <= 24; i ++) cin >> a[i]; if(ok()) printf("YES "); else printf("NO "); return 0; }