前 言
前端
AngularJS是为了克服HTML在构建应用上的不足而设计的。(引用百度百科)
本篇学习主要有两个部分:
①【AngularJS 过滤器】
②【AngularJS 服务 】
1、AngularJS 过滤器 |
【常用指令】
AngularJS 过滤器:过滤器可以使用一个管道字符(|)添加到表达式和指令中。
>>>系统内置过滤器:
currency 格式化数字为货币格式。
filter 从数组项中选择一个子集。
lowercase 格式化字符串为小写。
orderBy 根据某个表达式排列数组。
uppercase 格式化字符串为大写。
[lowercase、uppercase、currency]
<body ng-app="app" ng-controller="ctrl"> <p>{{"aBcDeF"|lowercase}}</p> // 输出为abcdef <p>{{"aBcDeF"|uppercase}}</p> //输出为ABCDEF <p>{{123456|currency}}</p> //输出为$123,456.00 </body> <script language="JavaScript" src="angular-1.5.6/angular.js"></script> <script type="text/javascript"> angular.module("app",[]) .controller("ctrl",function($scope){ }) </script>
【filter和orderBy】
写到这里就不得不说一下angular中的
写到这里就不得不说一下angular中的
ng-repeat
指令
ng-repeat 指令用于循环输出指定次数的 HTML 元素。
集合必须是数组或对象。
1 <!DOCTYPE html> 2 <html> 3 <head> 4 <meta charset="UTF-8"> 5 <title></title> 6 <link rel="stylesheet" href="libs/bootstrap.css" /> 7 </head> 8 9 <body ng-app="app" ng-controller="ctrl"> 10 11 <form class="form-horizontal"> 12 13 </form> 14 <div class="form-group"> 15 <label>请输入筛选信息:</label> 16 <input type="text" ng-model="search" /> 17 </div> 18 <table class="table table-bordered"> 19 <thead> 20 <tr> 21 <th>姓名</th> 22 <th>年龄</th> 23 <th>成绩</th> 24 </tr> 25 </thead> 26 <tr ng-repeat="item in classes| filter:search | orderBy:'grade'"> 27 <td>{{item.name}}</td> 28 <td>{{item.age}}</td> 29 <td>{{item.grade}}</td> 30 </tr> 31 </table> 32 </body> 33 <script src="libs/angular.js"></script> 34 <script type="text/javascript"> 35 var app = angular.module("app",[]) 36 .controller("ctrl",function($scope){ 37 $scope.classes = [ 38 {name:"张二",age:"22",grade:"88"}, 39 {name:"张三",age:"21",grade:"89"}, 40 {name:"李四",age:"20",grade:"90"}, 41 {name:"李五",age:"21",grade:"91"}, 42 {name:"王大麻子",age:"18",grade:"92"}, 43 {name:"王二麻子",age:"17",grade:"93"} 44 ]; 45 }) 46 47 48 </script> 49 </html>
2、AngularJS 服务 |
【服务Service】
$location:它可以返回当前页面的 URL 地址。
$http 服务: 服务向服务器发送请求,应用响应服务器传送过来的数据。
$timeout 服务: JS window.setTimeout 函数。
$interval 服务: JS window.setInterval 函数
效果图:


1 <body ng-app="app" ng-controller="ctrl"> 2 <pre>{{local}}</pre> 3 <p ng-bind="myHeader"></p> 4 <p ng-bind="num"></p> 5 <p>255转为16进制为{{gongneng}}</p> 6 <p>{{hex}}</p> 7 <p>{{123|filt}}</p> 8 9 </body> 10 <script src="../libs/angular.js"></script> 11 <script> 12 13 angular.module("app",[]) 14 .controller("ctrl",function($scope,$location,$timeout,$interval,$hexafy){ 15 $scope.local = $location.$$host; 16 $timeout(function () { 17 $scope.myHeader = "How are you today?"; 18 }, 2000); 19 $scope.num = 0; 20 $interval(function(){ 21 $scope.num ++; 22 },1000); 23 24 $scope.gongneng = $hexafy.$$gongneng; 25 $scope.hex = $hexafy.myFunc(255); 26 27 }) 28 /*自定义服务*/ 29 .service('$hexafy', function() { 30 this.$$gongneng = "将转入的数字,转为16进制"; 31 this.myFunc = function (x) { 32 return x.toString(16); 33 } 34 }) 35 .filter("filt",function(){ 36 return function(x){ 37 return x.toString(16); 38 } 39 }) 40 </script>
【自定义服务factory】
factory 是一个函数用于返回值,通常我们使用 factory 函数来计算或返回值。(factory使用上,与service差距不大)
效果图:


1 <body ng-app="app" ng-controller="ctrl"> 2 <p> 3 [功能]<br/> 4 {{gongneng}} 5 </p> 6 <p> 7 255转成16进制为:{{num}} 8 </p> 9 </body> 10 <script src="../libs/angular.js"></script> 11 <script> 12 13 angular.module("app",[]) 14 .controller("ctrl",function($scope,hexafy){ 15 $scope.gongneng = hexafy.gongneng; 16 $scope.num = hexafy.myFunc(255); 17 }) 18 .factory('hexafy',function(){ 19 var obj = { 20 gongneng : "将转入的数字,转为16进制", 21 myFunc:function(x){ 22 return x.toString(16); 23 } 24 }; 25 return obj; 26 }) 27 /*自定义服务*/ 28 // .service('hexafy', function() { 29 // this.gongneng = "将转入的数字,转为16进制"; 30 // this.myFunc = function (x) { 31 // return x.toString(16); 32 // } 33 // }) 34 35 36 </script>
【自定义服务provide】
1、在AngularJS中,Service,factory都是基于provider实现的。
2、在provider中,通过$get()方法提供了factory的写法,用于返回 value/service/factory。;
3、provider是三种自定义服务中,唯一可以写进config配置阶段的一种。
效果图:
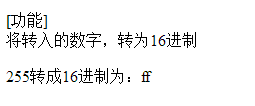

1 <body ng-app="app" ng-controller="ctrl"> 2 <p> 3 [功能]<br/> 4 {{gongneng}} 5 </p> 6 <p> 7 255转成16进制为:{{num}} 8 </p> 9 </body> 10 <script src="../libs/angular.js"></script> 11 <script> 12 13 angular.module("app",[]) 14 .controller("ctrl",function($scope,hexafy){ 15 $scope.gongneng = hexafy.gongneng; 16 $scope.num = hexafy.myFunc(255); 17 }) 18 19 /*定义一个provider服务*/ 20 .provider('hexafy',function(){ 21 // this.gongneng = "将转入的数字,转为16进制"; 22 this.$get = function(){ 23 var obj = { 24 gongneng : "将转入的数字,转为16进制", 25 myFunc : function(x){ 26 return x.toString(16); 27 } 28 } 29 return obj; 30 } 31 }) 32 33 // .factory('hexafy',function(){ 34 // var obj = { 35 // gongneng : "将转入的数字,转为16进制", 36 // myFunc:function(x){ 37 // return x.toString(16); 38 // } 39 // }; 40 // return obj; 41 // }) 42 43 /*自定义服务*/ 44 // .service('hexafy', function() { 45 // this.gongneng = "将转入的数字,转为16进制"; 46 // this.myFunc = function (x) { 47 // return x.toString(16); 48 // } 49 // }) 50 51 52 </script>
学习时候的笔记,可能会有一些错误的地方,欢迎各位的批评指点。
反思,复盘,每天收获一点---------------------期待更好的自己