http://poj.org/problem?id=2935
Time Limit: 1000MS | Memory Limit: 65536K | |||
Total Submissions: 3220 | Accepted: 1457 | Special Judge |
Description
In this problem you have to solve a very simple maze consisting of:
- a 6 by 6 grid of unit squares
- 3 walls of length between 1 and 6 which are placed either horizontally or vertically to separate squares
- one start and one end marker
A maze may look like this:
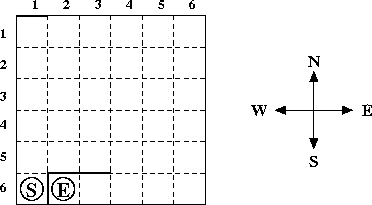
You have to find a shortest path between the square with the start marker and the square with the end marker. Only moves between adjacent grid squares are allowed; adjacent means that the grid squares share an edge and are not separated by a wall. It is not allowed to leave the grid.
Input
The input consists of several test cases. Each test case consists of five lines: The first line contains the column and row number of the square with the start marker, the second line the column and row number of the square with the end marker. The third, fourth and fifth lines specify the locations of the three walls. The location of a wall is specified by either the position of its left end point followed by the position of its right end point (in case of a horizontal wall) or the position of its upper end point followed by the position of its lower end point (in case of a vertical wall). The position of a wall end point is given as the distance from the left side of the grid followed by the distance from the upper side of the grid.
You may assume that the three walls don’t intersect with each other, although they may touch at some grid corner, and that the wall endpoints are on the grid. Moreover, there will always be a valid path from the start marker to the end marker. Note that the sample input specifies the maze from the picture above.
The last test case is followed by a line containing two zeros.
Output
For each test case print a description of a shortest path from the start marker to the end marker. The description should specify the direction of every move (‘N’ for up, ‘E’ for right, ‘S’ for down and ‘W’ for left).
There can be more than one shortest path, in this case you can print any of them.
Sample Input
1 6 2 6 0 0 1 0 1 5 1 6 1 5 3 5 0 0
Sample Output
NEEESWW
Source
1 #include<iostream> 2 #include<cstring> 3 #include<cstdio> 4 #include<algorithm> 5 #include<queue> 6 using namespace std; 7 #define inf 0x3f3f3f3f 8 bool can[10][10][10][10]; 9 bool vis[10][10]; 10 int path[10][10]; 11 int fx[4][2]={1,0,-1,0,0,1,0,-1}; 12 char idx[4]={'N','S','W','E'}; 13 struct node{int x,y,bs;}P[15]; 14 void print(int x,int y,int bs) 15 { 16 if(bs==0) return; 17 for(int i=0;i<4;++i) 18 { 19 int dx=x+fx[i][0]; 20 int dy=y+fx[i][1]; 21 if(dx<1||dy<1||dx>6||dy>6||path[dx][dy]+1!=bs||can[dx][dy][x][y]) continue; 22 else{ 23 print(dx,dy,bs-1); 24 printf("%c",idx[i]); 25 return; 26 } 27 } 28 } 29 void bfs(node s,node e) 30 { 31 memset(vis,0,sizeof(vis)); 32 memset(path,inf,sizeof(path)); 33 path[s.x][s.y]=0; 34 queue<node>q; 35 s.bs=0; 36 q.push(s); 37 while(!q.empty()){ 38 node t=q.front();q.pop(); 39 if(t.x==e.x&&t.y==e.y) {print(t.x,t.y,t.bs);return;} 40 if(vis[t.x][t.y]) continue; 41 vis[t.x][t.y]=1; 42 for(int i=0;i<4;++i) 43 { 44 node tt=t; 45 int dx=tt.x+fx[i][0]; 46 int dy=tt.y+fx[i][1]; 47 if(dx<1||dy<1||dx>6||dy>6||vis[dx][dy]||can[tt.x][tt.y][dx][dy]||path[dx][dy]<=tt.bs+1) continue; 48 path[dx][dy]=tt.bs+1; 49 q.push(node{dx,dy,tt.bs+1}); 50 } 51 } 52 } 53 int main() 54 { 55 while(scanf("%d%d",&P[1].y,&P[1].x)!=EOF){ 56 if(P[1].x==0&&P[1].y==0) break; 57 scanf("%d%d",&P[2].y,&P[2].x); 58 memset(can,0,sizeof(can)); 59 for(int i=0;i<3;++i) 60 { 61 int x1,y1,x2,y2; 62 scanf("%d%d%d%d",&y1,&x1,&y2,&x2); 63 if(x1==x2){ 64 int miny=min(y1,y2)+1,maxy=max(y1,y2); 65 for(int j=miny;j<=maxy;++j) 66 can[x1][j][x1+1][j]=can[x1+1][j][x1][j]=1; 67 } 68 else{ 69 int minx=min(x1,x2)+1,maxx=max(x1,x2); 70 for(int j=minx;j<=maxx;++j) 71 { 72 can[j][y1][j][y1+1]=can[j][y1+1][j][y1]=1; 73 } 74 } 75 } 76 bfs(P[1],P[2]); 77 puts(""); 78 } 79 return 0; 80 }