#画笑脸-。-
#RandomSmileys.py
import random
import turtle
t = turtle.Pen()
t.speed(0)
t.hideturtle()
turtle.bgcolor("black")
def draw_smiley(x,y):
t.penup()
t.setpos(x,y)
t.pendown()
# Head
t.pencolor("yellow")
t.fillcolor("yellow")
t.begin_fill()
t.circle(50)
t.end_fill()
# Left eye
t.setpos(x-15, y+60)
t.fillcolor("blue")
t.begin_fill()
t.circle(10)
t.end_fill()
# Right eye
t.setpos(x+15, y+60)
t.begin_fill()
t.circle(10)
t.end_fill()
# Mouth
t.setpos(x-25, y+40)
t.pencolor("black")
t.width(10)
t.goto(x-10, y+20)
t.goto(x+10, y+20)
t.goto(x+25, y+40)
t.width(1)
for n in range(50):
x = random.randrange(-turtle.window_width()//2,
turtle.window_width()//2)
y = random.randrange(-turtle.window_height()//2,
turtle.window_height()//2)
draw_smiley(x,y)
# 跳动的笑脸-。-
# 图片:
# SmileyBounce2.py
import pygame # Setup
pygame.init()
screen = pygame.display.set_mode([800,600])
keep_going = True
pic = pygame.image.load("CrazySmile.jpg")
colorkey = pic.get_at((0,0))
pic.set_colorkey(colorkey)
picx = 0
picy = 0
BLACK = (0,0,0)
timer = pygame.time.Clock()
speedx = 5
speedy = 5
while keep_going: # Game loop
for event in pygame.event.get():
if event.type == pygame.QUIT:
keep_going = False
picx += speedx
picy += speedy
if picx <= 0 or picx + pic.get_width() >= 800:
speedx = -speedx
if picy <= 0 or picy + pic.get_height() >= 600:
speedy = -speedy
screen.fill(BLACK)
screen.blit(pic, (picx, picy))
pygame.display.update()
timer.tick(60)
pygame.quit() # Exit
# 笑脸爆炸图(PS:可以自己换图图片)
# 图片: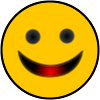
# SmileyExplosion.py
import pygame
import random
BLACK = (0,0,0)
pygame.init()
screen = pygame.display.set_mode([800,600])
pygame.display.set_caption("Smiley Explosion")
mousedown = False
keep_going = True
clock = pygame.time.Clock()
pic = pygame.image.load("CrazySmile.jpg")
colorkey = pic.get_at((0,0))
pic.set_colorkey(colorkey)
sprite_list = pygame.sprite.Group()
class Smiley(pygame.sprite.Sprite):
pos = (0,0)
xvel = 1
yvel = 1
scale = 100
def __init__(self, pos, xvel, yvel):
pygame.sprite.Sprite.__init__(self)
self.image = pic
self.scale = random.randrange(10,100)
self.image = pygame.transform.scale(self.image, (self.scale,self.scale))
self.rect = self.image.get_rect()
self.pos = pos
self.rect.x = pos[0] - self.scale/2
self.rect.y = pos[1] - self.scale/2
self.xvel = xvel
self.yvel = yvel
def update(self):
self.rect.x += self.xvel
self.rect.y += self.yvel
if self.rect.x <= 0 or self.rect.x > screen.get_width() - self.scale:
self.xvel = -self.xvel
if self.rect.y <= 0 or self.rect.y > screen.get_height() - self.scale:
self.yvel = -self.yvel
while keep_going:
for event in pygame.event.get():
if event.type == pygame.QUIT:
keep_going = False
if event.type == pygame.MOUSEBUTTONDOWN:
mousedown = True
if event.type == pygame.MOUSEBUTTONUP:
mousedown = False
screen.fill(BLACK)
sprite_list.update()
sprite_list.draw(screen)
clock.tick(60)
pygame.display.update()
if mousedown:
speedx = random.randint(-5, 5)
speedy = random.randint(-5, 5)
newSmiley = Smiley(pygame.mouse.get_pos(),speedx,speedy)
sprite_list.add(newSmiley)
pygame.quit()
# 接笑脸小游戏
# SmileyPong1.py
import pygame # Setup
pygame.init()
screen = pygame.display.set_mode([800,600])
pygame.display.set_caption("Smiley Pong")
keepGoing = True
pic = pygame.image.load("CrazySmile.jpg")
colorkey = pic.get_at((0,0))
pic.set_colorkey(colorkey)
picx = 0
picy = 0
BLACK = (0,0,0)
WHITE = (255,255,255)
timer = pygame.time.Clock()
speedx = 5
speedy = 5
paddlew = 200
paddleh = 25
paddlex = 300
paddley = 550
picw = 100
pich = 100
points = 0
lives = 5
font = pygame.font.SysFont("Times", 24)
while keepGoing: # Game loop
for event in pygame.event.get():
if event.type == pygame.QUIT:
keepGoing = False
picx += speedx
picy += speedy
if picx <= 0 or picx + pic.get_width() >= 800:
speedx = -speedx
if picy <= 0:
speedy = -speedy
if picy >= 500:
lives -= 1
speedy = -speedy
screen.fill(BLACK)
screen.blit(pic, (picx, picy))
# Draw paddle
paddlex = pygame.mouse.get_pos()[0]
paddlex -= paddlew/2
pygame.draw.rect(screen, WHITE, (paddlex, paddley, paddlew, paddleh))
# Check for paddle bounce
if picy + pich >= paddley and picy + pich <= paddley + paddleh
and speedy > 0:
if picx + picw / 2 >= paddlex and picx + picw / 2 <= paddlex +
paddlew:
points += 1
speedy = -speedy
# Draw text on screen
draw_string = "Lives: " + str(lives) + " Points: " + str(points)
text = font.render(draw_string, True, WHITE)
text_rect = text.get_rect()
text_rect.centerx = screen.get_rect().centerx
text_rect.y = 10
screen.blit(text, text_rect)
pygame.display.update()
timer.tick(60)
pygame.quit() # Exit
# 接笑脸2(速度与激情版:越来越快)
# SmileyPong2.py
import pygame # Setup
pygame.init()
screen = pygame.display.set_mode([800,600])
pygame.display.set_caption("Smiley Pong")
keepGoing = True
pic = pygame.image.load("CrazySmile.jpg")
colorkey = pic.get_at((0,0))
pic.set_colorkey(colorkey)
picx = 0
picy = 0
BLACK = (0,0,0)
WHITE = (255,255,255)
timer = pygame.time.Clock()
speedx = 5
speedy = 5
paddlew = 200
paddleh = 25
paddlex = 300
paddley = 550
picw = 100
pich = 100
points = 0
lives = 5
font = pygame.font.SysFont("Times", 24)
while keepGoing: # Game loop
for event in pygame.event.get():
if event.type == pygame.QUIT:
keepGoing = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_F1: # F1 = New Game
points = 0
lives = 5
picx = 0
picy = 0
speedx = 5
speedy = 5
picx += speedx
picy += speedy
if picx <= 0 or picx >= 700:
speedx = -speedx * 1.1
if picy <= 0:
speedy = -speedy + 1
if picy >= 500:
lives -= 1
speedy = -5
speedx = 5
picy = 499
screen.fill(BLACK)
screen.blit(pic, (picx, picy))
# Draw paddle
paddlex = pygame.mouse.get_pos()[0]
paddlex -= paddlew/2
pygame.draw.rect(screen, WHITE, (paddlex, paddley, paddlew, paddleh))
# Check for paddle bounce
if picy + pich >= paddley and picy + pich <= paddley + paddleh
and speedy > 0:
if picx + picw/2 >= paddlex and picx + picw/2 <= paddlex +
paddlew:
speedy = -speedy
points += 1
# Draw text on screen
draw_string = "Lives: " + str(lives) + " Points: " + str(points)
# Check whether the game is over
if lives < 1:
speedx = speedy = 0
draw_string = "Game Over. Your score was: " + str(points)
draw_string += ". Press F1 to play again. "
text = font.render(draw_string, True, WHITE)
text_rect = text.get_rect()
text_rect.centerx = screen.get_rect().centerx
text_rect.y = 10
screen.blit(text, text_rect)
pygame.display.update()
timer.tick(60)
pygame.quit() # Exit
# 爆炸笑脸(升级版:左键创造,右键消除)
# SmileyPop2.py
import pygame
import random
BLACK = (0,0,0)
WHITE = (255,255,255)
pygame.init()
screen = pygame.display.set_mode([800,600])
pygame.display.set_caption("Pop a Smiley")
mousedown = False
keep_going = True
clock = pygame.time.Clock()
pic = pygame.image.load("CrazySmile.jpg")
colorkey = pic.get_at((0,0))
pic.set_colorkey(colorkey)
sprite_list = pygame.sprite.Group()
pygame.mixer.init() # Add sounds
pop = pygame.mixer.Sound("pop.wav")
font = pygame.font.SysFont("Arial", 24)
count_smileys = 0
count_popped = 0
class Smiley(pygame.sprite.Sprite):
pos = (0,0)
xvel = 1
yvel = 1
scale = 100
def __init__(self, pos, xvel, yvel):
pygame.sprite.Sprite.__init__(self)
self.image = pic
self.scale = random.randrange(10,100)
self.image = pygame.transform.scale(self.image,
(self.scale,self.scale))
self.rect = self.image.get_rect()
self.pos = pos
self.rect.x = pos[0] - self.scale/2
self.rect.y = pos[1] - self.scale/2
self.xvel = xvel
self.yvel = yvel
def update(self):
self.rect.x += self.xvel
self.rect.y += self.yvel
if self.rect.x <= 0 or self.rect.x > screen.get_width() - self.scale:
self.xvel = -self.xvel
if self.rect.y <= 0 or self.rect.y > screen.get_height() - self.scale:
self.yvel = -self.yvel
while keep_going:
for event in pygame.event.get():
if event.type == pygame.QUIT:
keep_going = False
if event.type == pygame.MOUSEBUTTONDOWN:
if pygame.mouse.get_pressed()[0]: # Left mouse button, draw
mousedown = True
elif pygame.mouse.get_pressed()[2]: # Right mouse button, pop
pos = pygame.mouse.get_pos()
clicked_smileys = [s for s in sprite_list if
s.rect.collidepoint(pos)]
sprite_list.remove(clicked_smileys)
if len(clicked_smileys) > 0:
pop.play()
count_popped += len(clicked_smileys)
if event.type == pygame.MOUSEBUTTONUP:
mousedown = False
screen.fill(BLACK)
sprite_list.update()
sprite_list.draw(screen)
clock.tick(60)
draw_string = "Bubbles created: " + str(count_smileys)
draw_string += " - Bubbles popped: " + str(count_popped)
if (count_smileys > 0):
draw_string += " - Percent: "
draw_string += str(round(count_popped/count_smileys*100, 1))
draw_string += "%"
text = font.render(draw_string, True, WHITE)
text_rect = text.get_rect()
text_rect.centerx = screen.get_rect().centerx
text_rect.y = 10
screen.blit (text, text_rect)
pygame.display.update()
if mousedown:
speedx = random.randint(-5, 5)
speedy = random.randint(-5, 5)
newSmiley = Smiley(pygame.mouse.get_pos(), speedx, speedy)
sprite_list.add(newSmiley)
count_smileys += 1
pygame.quit()