Description
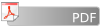
Instant Complexity
Instant Complexity |
Analyzing the run-time complexity of algorithms is an important tool for designing efficient programs that solve a problem. An algorithm that runs in linear time is usually much faster than analgorithm that takes quadratic time for the same task, and thus should be preferred.
Generally, one determines the run-time of an algorithm in relation to the `size'
n of the input,which could be the number of objects to be sorted, the number of points in a given polygon, and soon. Since determining a formula dependent on
n for the run-time of an algorithm is no easy task, itwould be great if this could be automated. Unfortunately, this is not possible in general, but in thisproblem we will consider programs of a very simple nature, for which it is possible. Our programsare
built according to the following rules (given in BNF), where < number > can be any non-negative integer:
- < Program > ::= "BEGIN" < Statementlist > "END"
- < Statementlist > ::= < Statement > | < Statement > < Statementlist >
- < Statement > ::= < LOOP-Statement > | < OP-Statement >
- < LOOP-Statement > ::= < LOOP-Header > < Statementlist > "END"
- < LOOP-Header > ::= "LOOP" < number > | "LOOP n"
- < OP-Statement > ::= "OP" < number >
The run-time of such a program can be computed as follows: the execution of an OP-statementcosts as many time-units as its parameter specifies. The statement list enclosed by a LOOP-statementis executed as many times as the parameter of the statement indicates, i.e., the given constant numberof times, if a number is given, and n times, if n is given. The run-time of a statement list is the sum ofthe times of its constituent parts. The total run-time therefore generally depends on n.
Input
The input file starts with a line containing the number k of programs in the input. Following this arek programs which are constructed according to the grammar given above. Whitespace and newlinescan appear anywhere in a program, but not within the keywords BEGIN, END, LOOP and OP or in aninteger value. The nesting depth of the LOOP-operators will be at most 10.Output
For each program in the input, first output the number of the program, as shown in the sampleoutput. Then output the run-time of the program in terms of n; this will be a polynomial of degree 
Runtime =
a*n^10+
b*n^9+ . . . +
i*n^2+
j*n+k
'', where terms with zero coefficients are leftout, and factors of 1 are not written. If the runtime is zero, just print ``Runtime = 0''.
Output a blank line after each test case.
Sample Input
2 BEGIN LOOP n OP 4 LOOP 3 LOOP n OP 1 END OP 2 END OP 1 END OP 17 END BEGIN OP 1997 LOOP n LOOP n OP 1 END END END
Sample Output
Program #1 Runtime = 3*n^2+11*n+17 Program #2 Runtime = n^2+1997
题意:模拟求时间复杂度,OP表示运行几次,每个“LOOP”相应一个“END”,在“LOOP”到“END”范围内的语句都要循环运行“LOOP”后面的数字的次数,这个数字有可能是常数。有可能是变量“n”。
思路:模拟递归调用,记录多项式的系数值
#include <iostream> #include <cstdio> #include <cstring> #include <algorithm> #include <vector> using namespace std; const int MAXN = 15; char str1[MAXN], str2[MAXN]; int ans[MAXN]; void solve(int a[], int n) { int m; while (scanf("%s", str1) && str1[0] != 'E') { if (str1[0] == 'O') { scanf("%d", &m); a[0] += m; } else { scanf("%s", str2); if (str2[0] == 'n') m = -1; else sscanf(str2, "%d", &m); int b[MAXN]; memset(b, 0, sizeof(b)); solve(b, m); for (int i = 0; i < MAXN; i++) a[i] += b[i]; } } if (n == -1) { for (int i = MAXN-1; i >= 1; i--) a[i] = a[i-1]; a[0] = 0; } else { for (int i = 0; i < MAXN; i++) a[i] *= n; } } void print() { int flag = 0; for (int i = MAXN-1; i >= 1; i--) if (ans[i]) { if (!flag) flag = 1; else printf("+"); if (ans[i] > 1) printf("%d*", ans[i]); if (i == 1) printf("n"); else printf("n^%d", i); } if (ans[0]) { if (!flag) flag = 1; else printf("+"); printf("%d", ans[0]); } if (!flag) printf("0"); printf(" "); } int main() { int t, cas = 1;; scanf("%d", &t); while (t--) { memset(ans, 0, sizeof(ans)); scanf("%s", str1); solve(ans, 1); printf("Program #%d Runtime = ", cas++); print(); } return 0; }
版权声明:本文博主原创文章,博客,未经同意,不得转载。