编写代码
1,新建服务端 TcpServerDemo01
package com.xiang.lesson02;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.ServerSocket;
import java.net.Socket;
//服务端
public class TcpServerDemo01 {
public static void main(String[] args) {
ServerSocket socket = null;
Socket accept = null;
InputStream inputStream = null;
ByteArrayOutputStream byteArrayOutputStream = null;
// 1,需要有一个地址
// new 一个地址;
try {
socket = new ServerSocket(999);
while (true){
// 2, 等待客户端连接
accept = socket.accept();
// 3, 读取 客户端的消息
inputStream = accept.getInputStream();
// 管道流
byteArrayOutputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = inputStream.read(buffer)) != -1) {
byteArrayOutputStream.write(buffer, 0, len);
}
System.out.println(byteArrayOutputStream.toString());
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//关闭资源
if (byteArrayOutputStream != null) {
try {
byteArrayOutputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (accept != null) {
try {
accept.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket != null) {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
2,新建服务端 TcpClientDemo01
package com.xiang.lesson02;
import java.io.IOException;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.Socket;
import java.net.UnknownHostException;
import java.nio.charset.StandardCharsets;
//客户端
public class TcpClientDemo01 {
public static void main(String[] args) {
Socket socket = null;
OutputStream outputStream = null;
// 1,要知道服务器的地址
// 连这个地址 localhost 999
try {
InetAddress serverIP = InetAddress.getByName("127.0.0.1");
// 2 端口号
int port = 999;
// 3,创建一个 socket 连接
socket = new Socket(serverIP, port);
// 4,发送消息 io 流
// 输出 流
outputStream = socket.getOutputStream();
// 写
outputStream.write("你好,欢迎学习到了网络编程".getBytes(StandardCharsets.UTF_8));
} catch (Exception e) {
e.printStackTrace();
} finally {
if (outputStream != null) {
try {
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (socket != null) {
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
3,运行结果
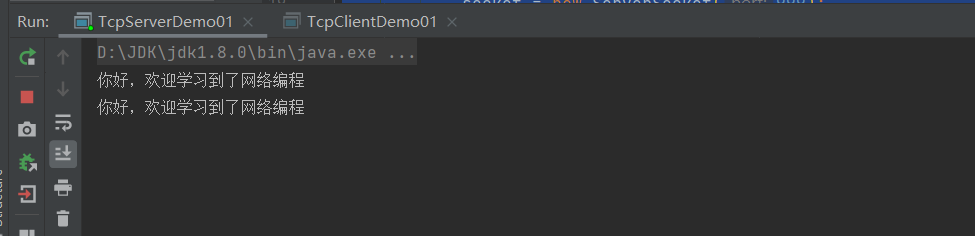