Sudoku
Time Limit: 2000MS | Memory Limit: 65536K | |||
Total Submissions: 17953 | Accepted: 8688 | Special Judge |
Description
Sudoku is a very simple task. A square table with 9 rows and 9 columns is divided to 9 smaller squares 3x3 as shown on the Figure. In some of the cells are written decimal digits from 1 to 9. The other cells are empty. The goal is to fill the empty cells with decimal digits from 1 to 9, one digit per cell, in such way that in each row, in each column and in each marked 3x3 subsquare, all the digits from 1 to 9 to appear. Write a program to solve a given Sudoku-task.
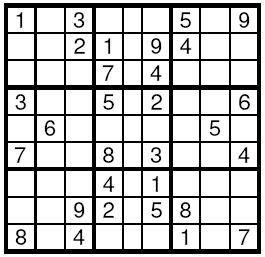
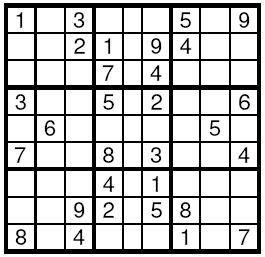
Input
The input data will start with the number of the test cases. For each test case, 9 lines follow, corresponding to the rows of the table. On each line a string of exactly 9 decimal digits is given, corresponding to the cells in
this line. If a cell is empty it is represented by 0.
Output
For each test case your program should print the solution in the same format as the input data. The empty cells have to be filled according to the rules. If solutions is not unique, then the program may print any one of them.
Sample Input
1 103000509 002109400 000704000 300502006 060000050 700803004 000401000 009205800 804000107
Sample Output
143628579 572139468 986754231 391542786 468917352 725863914 237481695 619275843 854396127
Source
最近重装电脑,换成linux的ubuntu系统,还学了emacs的一点皮毛,被弄的晕头转向。。。
题目要ac倒是没有什么难度,最裸的暴力,没有加什么剪枝。分分钟水过。
15854066 | ksq2013 | 2676 | Accepted | 704K | 844MS | G++ | 1849B | 2016-07-30 21:30:13 |
#include<cstdio> #include<cstdlib> #include<cstring> #include<iostream> using namespace std; int thd[4]={0,3,6,9},sdk[10][10]; bool col[10][10],row[10][10]; bool jud(int x,int y,int z) { int xs,xt,ys,yt; for(int i=1;i<=3;i++) if(x<=thd[i]){ xs=thd[i-1]+1; xt=thd[i]; break; } for(int i=1;i<=3;i++) if(y<=thd[i]){ ys=thd[i-1]+1; yt=thd[i]; break; } for(int i=xs;i<=xt;i++) for(int j=ys;j<=yt;j++) if(!(sdk[i][j]^z)) return false;//this answer is ; return true; } bool dfs() { for(int i=1;i<=9;i++) for(int j=1;j<=9;j++) if(!sdk[i][j]){ for(int k=1;k<=9;k++){ if((!col[j][k])&&(!row[i][k])&&jud(i,j,k)){ sdk[i][j]=k; col[j][k]=row[i][k]=1; if(dfs())return true;//selected the right answer; col[j][k]=row[i][k]=0; sdk[i][j]=0; } } return false;//it was impossible to deduce an answer in a certain unit,then it's unreachable; } return true;//the units were filled correctly; } int main() { int T; scanf("%d",&T); while(T--){ memset(col,false,sizeof(col)); memset(row,false,sizeof(row)); for(int i=1;i<=9;i++) for(int j=1;j<=9;j++){ char ch; cin>>ch; sdk[i][j]=ch-'0'; col[j][sdk[i][j]]=true; row[i][sdk[i][j]]=true;//Init(); } dfs(); for(int i=1;i<=9;i++){ for(int j=1;j<=9;j++) printf("%d",sdk[i][j]); putchar(' '); } } return 0; }
看了网上的题解后,发现反着搜,即从右下角向左上角搜更快逼近正解,于是稍稍修改了程序,果然快了许多倍。
15854143 | ksq2013 | 2676 | Accepted | 704K | 16MS | G++ | 1849B | 2016-07-30 21:44:56 |
#include<cstdio> #include<cstdlib> #include<cstring> #include<iostream> using namespace std; int thd[4]={0,3,6,9},sdk[10][10]; bool col[10][10],row[10][10]; bool jud(int x,int y,int z) { int xs,xt,ys,yt; for(int i=1;i<=3;i++) if(x<=thd[i]){ xs=thd[i-1]+1; xt=thd[i]; break; } for(int i=1;i<=3;i++) if(y<=thd[i]){ ys=thd[i-1]+1; yt=thd[i]; break; } for(int i=xs;i<=xt;i++) for(int j=ys;j<=yt;j++) if(!(sdk[i][j]^z)) return false;//this answer is ; return true; } bool dfs() { for(int i=9;i>=1;i--) for(int j=9;j>=1;j--) if(!sdk[i][j]){ for(int k=1;k<=9;k++){ if((!col[j][k])&&(!row[i][k])&&jud(i,j,k)){ sdk[i][j]=k; col[j][k]=row[i][k]=1; if(dfs())return true;//selected the right answer; col[j][k]=row[i][k]=0; sdk[i][j]=0; } } return false;//it was impossible to deduce an answer in a certain unit,then it's unreachable; } return true;//the units were filled correctly; } int main() { int T; scanf("%d",&T); while(T--){ memset(col,false,sizeof(col)); memset(row,false,sizeof(row)); for(int i=1;i<=9;i++) for(int j=1;j<=9;j++){ char ch; cin>>ch; sdk[i][j]=ch-'0'; col[j][sdk[i][j]]=true; row[i][sdk[i][j]]=true;//Init(); } dfs(); for(int i=1;i<=9;i++){ for(int j=1;j<=9;j++) printf("%d",sdk[i][j]); putchar(' '); } } return 0; }