管道是一种把两个进程之间的标准输入和标准输出连接起来的机制,从而提供一种让多个进程间通信的方法,当进程创建管道时,每次
都需要提供两个文件描述符来操作管道。其中一个对管道进行写操作,另一个对管道进行读操作。对管道的读写与一般的IO系统函数一
致,使用write()函数写入数据,使用read()读出数据。
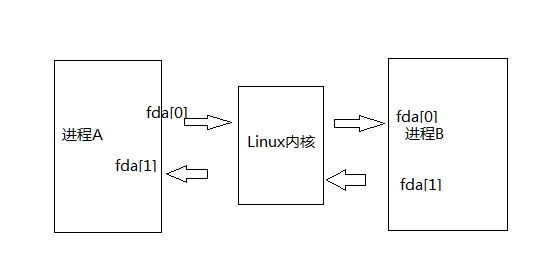
#include<unistd.h>
int pipe(int filedes[2]);
返回值:成功,返回0,否则返回-1。参数数组包含pipe使用的两个文件的描述符。fd[0]:读管道,fd[1]:写管道。
必须在fork()中调用pipe(),否则子进程不会继承文件描述符。两个进程不共享祖先进程,就不能使用pipe。但是可以使用命名管道。
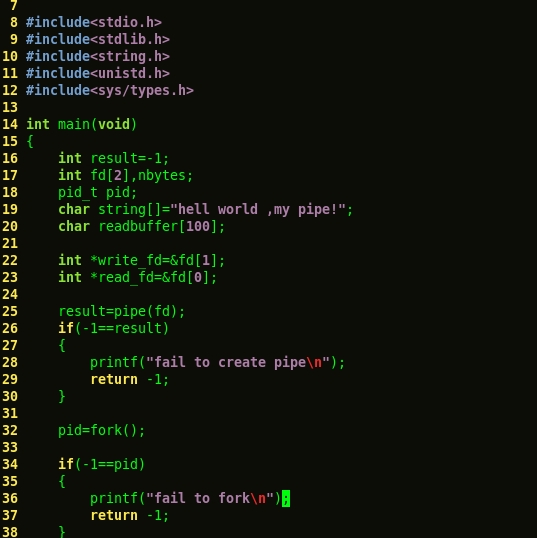
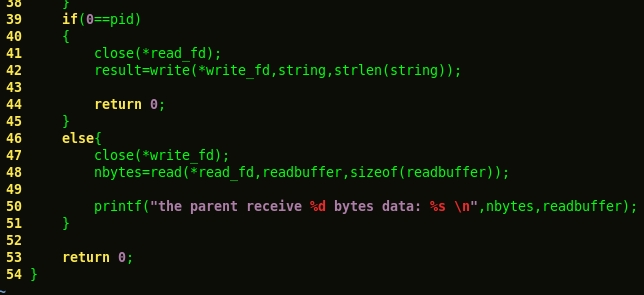

当管道进行写入操作的时候,如果写入的数据小于128K则是非原子的,如果大于128K字节,缓冲区的数据将被连续地写入
管道,直到全部数据写完为止,如果没有进程读取数据,则将一直阻塞,如下:
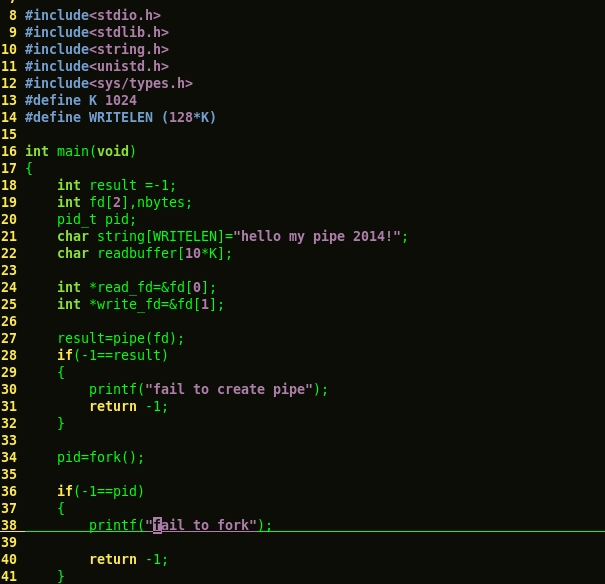
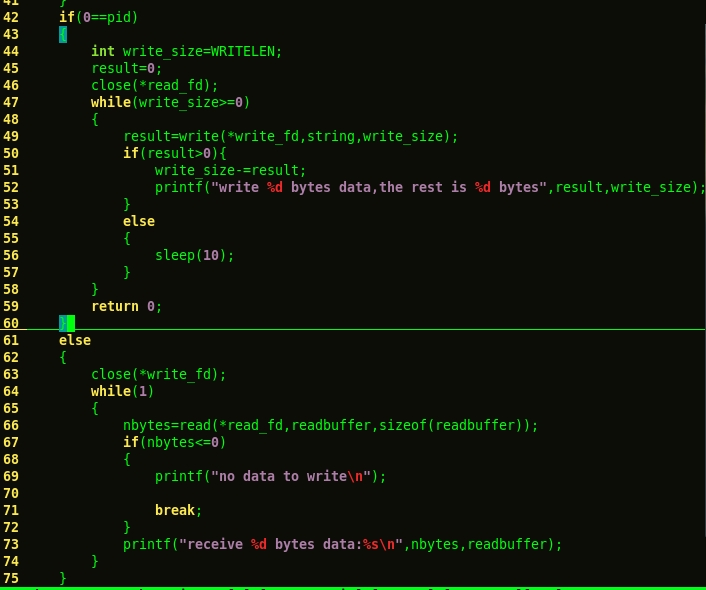

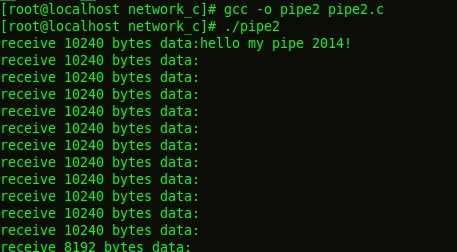
在上例程序中,子进程一次性写入128K数据,当父进程将全部数据读取完毕的时候,子进程的write()函数才结束阻塞并且
返回写入信息。
命名管道FIFO
管道最大的劣势就是没有名字,只能用于有一个共同祖先进程的各个进程之间。FIFO代表先进先出,单它是一个单向数据流,也就是半双工,和
管道不同的是:每个FIFO都有一个路径与之关联,从而允许无亲缘关系的进程访问。
#include <sys/types.h>
#include <sys/stat.h>
int mkfifo(const char *pathname, mode_t mode);
这里pathname是路径名,mode是sys/stat.h里面定义的创建文件的权限.
以下示例程序来自:http://blog.chinaunix.net/uid-20498361-id-1940238.html
有亲缘关系进程间的fifo的例子
/* * 有亲缘关系的进程间的fifo的使用 * fifo 使用的简单例子 */
#include "../all.h"
#define FIFO_PATH "/tmp/hover_fifo"
void do_sig(int signo) { if (signo == SIGCHLD) while (waitpid(-1, NULL, WNOHANG) > 0) ; }
int main(void) { int ret; int fdr, fdw; pid_t pid;
char words[10] = "123456789"; char buf[10] = {' '}; // 创建它,若存在则不算是错误, // 若想修改其属性需要先打开得到fd,然后用fcntl来获取属性,然后设置属性.
if (((ret = mkfifo(FIFO_PATH, FILE_MODE)) == -1)
&& (errno != EEXIST)) perr_exit("mkfifo()"); fprintf(stderr, "fifo : %s created successfully!
", FIFO_PATH);
signal(SIGCHLD, do_sig);
pid = fork(); if (pid == 0) { // child
if ((fdr = open(FIFO_PATH, O_WRONLY)) < 0) // 打开fifo用来写 perr_exit("open()"); sleep(2);
// 写入数据 if (write(fdr, words, sizeof(words)) != sizeof(words)) perr_exit("write"); fprintf(stderr, "child write : %s
", words); close(fdw); } else if (pid > 0) { // parent
if ((fdr = open(FIFO_PATH, O_RDONLY)) < 0) // 打开fifo用来读
perr_exit("open()");
fprintf(stderr, "I father read, waiting for child ...
"); if (read(fdr, buf, 9) != 9) //读数据 perr_exit("read");
fprintf(stderr, "father get buf : %s
", buf); close(fdr); } // 到这里fifo管道并没有被删除,必须手动调用函数unlink或remove删除.
return 0; }
|
从例子上可以看出使用fifo时需要注意:
*fifo管道是先调用mkfifo创建,然后再用open打开得到fd来使用.
*在打开fifo时要注意,它是半双工的的,一般不能使用O_RDWR打开,而只能用只读或只写打开.
fifo可以用在非亲缘关系的进程间,而它的真正用途是在服务器和客户端之间. 由于它是半双工的所以,如果要进行客户端和服务器双方的通信的话,
每个方向都必须建立两个管道,一个用于读,一个用于写.
下面是一个服务器,对多个客户端的fifo的例子:
server 端的例子:
/* * FIFO server */
#include "all.h"
int main(void) { int fdw, fdw2; int fdr; char clt_path[PATH_LEN] = {' '}; char buf[MAX_LINE] = {' '}; char *p; int n; if (mkfifo(FIFO_SVR, FILE_MODE) == -1 && errno != EEXIST) perr_exit("mkfifo()"); if ((fdr = open(FIFO_SVR, O_RDONLY)) < 0) perr_exit("open()"); /* * 根据fifo的创建规则, 若从一个空管道或fifo读,
* 而在读之前管道或fifo有打开来写的操作, 那么读操作将会阻塞 * 直到管道或fifo不打开来读, 或管道或fifo中有数据为止.
*
* 这里,我们的fifo本来是打开用来读的,但是为了,read不返回0,
* 让每次client端读完都阻塞在fifo上,我们又打开一次来读. * 见unpv2 charper 4.7 */ if ((fdw2 = open(FIFO_SVR, O_WRONLY)) < 0) fprintf(stderr, "open()"); while (1) { /* read client fifo path from FIFO_SVR */
/* 这里由于FIFO_SVR有打开来写的操作,所以当管道没有数据时,
* read会阻塞,而不是返回0.
*/ if (read(fdr, clt_path, PATH_LEN) < 0) { fprintf(stderr, "read fifo client path error : %s
", strerror(errno)); break; } if ((p = strstr(clt_path, "
")) == NULL) { fprintf(stderr, "clt_path error: %s
", clt_path); break; } *p = ' '; DBG("clt_path", clt_path); if (access(clt_path, W_OK) == -1) { // client fifo ok, but no permission
perror("access()"); continue; } /* open client fifo for write */ if ((fdw = open(clt_path, O_WRONLY)) < 0) { perror("open()"); continue; } if ((n = read(fdr, buf, WORDS_LEN)) > 0) { /* read server words is ok */ printf("server read words : %s
", buf); buf[n] = ' '; write(fdw, buf, strlen(buf)); } } close(fdw); unlink(FIFO_SVR); exit(0); }
|
客户端的例子:
/* * Fifo client * */ #include "all.h"
int main(void) { int fdr, fdw; pid_t pid; char clt_path[PATH_LEN] = {' '}; char buf[MAX_LINE] = {' '}; char buf_path[MAX_LINE] = {' '}; snprintf(clt_path, PATH_LEN, FIFO_CLT_FMT, (long)getpid()); DBG("clt_path1 = ", clt_path); snprintf(buf_path, PATH_LEN, "%s
", clt_path);
if (mkfifo(clt_path, FILE_MODE) == -1 && errno != EEXIST) perr_exit("mkfifo()");
/* client open clt_path for read * open server for write */ if ((fdw = open(FIFO_SVR, O_WRONLY)) < 0) perr_exit("open()"); /* write my fifo path to server */ if (write(fdw, buf_path, PATH_LEN) != PATH_LEN) perr_exit("write()"); if (write(fdw, WORDS, WORDS_LEN) < 0) /* write words to fifo server */ perr_exit("error");
if ((fdr = open(clt_path, O_RDONLY)) < 0) perr_exit("open()"); if (read(fdr, buf, WORDS_LEN) > 0) { /* read reply from fifo server */ buf[WORDS_LEN] = ' '; printf("server said : %s
", buf); } close(fdr); unlink(clt_path); exit(0); }
|