思路:创建一个窗口类、形状类、矩形类、线条类、圆形类,在窗口中实现要求。
package mt.util;
import java.awt.Color;
/**
* 工具
* @author mt
*
*/
public final class MyUtil {
private MyUtil() {
}
/**
* 产生指定范围内的随机数
* @param min 最小值(闭区间)
* @param max 最大值(闭区间)
* @return 指定范围内的随机数
*/
public static int random(int min , int max) {
return (int) (Math.random() * (max -min +1) +min);
}
public static Color randomColor() {
int r = random(0,255);
int g = random(0,255);;
int b = random(0,255);;
return new Color(r,g,b);
}
}
package mt.shape;
import java.awt.Color;
import java.awt.Graphics;
/**
* 图形(抽象的不能实例化)
* @author mt
*
*/
public abstract class Shape {
protected int x1, y1; //开始坐标
protected int x2, y2; //结束坐标
protected Color color;
/**
* 绘图(抽象方法留给子类重写)
* @param g 画笔
*/
public abstract void draw(Graphics g);
public int getX1() {
return x1;
}
public void setX1(int x1) {
this.x1 = x1;
}
public int getY1() {
return y1;
}
public void setY1(int y1) {
this.y1 = y1;
}
public int getX2() {
return x2;
}
public void setX2(int x2) {
this.x2 = x2;
}
public int getY2() {
return y2;
}
public void setY2(int y2) {
this.y2 = y2;
}
public void setColor(Color color) {
this.color = color;
}
}
package mt.shape;
import java.awt.Graphics;
/**
* 矩形
* @author mt
*
*/
public class Rectangle extends Shape {
@Override
public void draw(Graphics g) {
int width = Math.abs(x1 - x2);
int height = Math.abs(y1 - y2);
int x = x1 < x2? x1 : x2;
int y = y1 < y2? y1 : y2;
g.setColor(color);
g.drawRect(x, y, width, height);
}
}
package mt.shape;
import java.awt.Graphics;
/**
* 圆形
* @author mt
*
*/
public class Oval extends Shape {
@Override
public void draw(Graphics g) {
int width = Math.abs(x1 - x2);
int height = Math.abs(y1 - y2);
int x = x1 < x2? x1 : x2;
int y = y1 < y2? y1 : y2;
g.setColor(color);
g.drawOval(x, y, width, height);
}
}
package mt.shape;
import java.awt.Graphics;
/**
* 线条
* @author mt
*
*/
public class Line extends Shape {
@Override
public void draw(Graphics g) {
g.setColor(color);
g.drawLine(x1, y1, x2, y2);
}
}
package mt.iu;
import java.awt.FlowLayout;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import mt.shape.Line;
import mt.shape.Oval;
import mt.shape.Rectangle;
import mt.shape.Shape;
import mt.util.MyUtil;
/**
* 我的窗口
* @author mt
*
*/
@SuppressWarnings("serial")
public class MyFrame extends JFrame {
/**
* 创建一个点击按钮后执行行为的类
* @author mt
*
*/
private class ButtonHander implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
String commond = e.getActionCommand();
if (commond.equals("Line")) {
shape = line;
}
else if (commond.equals("oval")) {
shape = oval;
}
else {
shape = rectangle;
}
shape.setX1(MyUtil.random(0, 800));
shape.setY1(MyUtil.random(0, 700));
shape.setX2(MyUtil.random(0, 800));
shape.setY2(MyUtil.random(0, 700));
shape.setColor(MyUtil.randomColor());
repaint();
}
};
private JButton lineButton ; //画线按钮
private JButton ovalButton ; //画圆按钮
private JButton rectangleButton ; //画矩形按钮
private Shape shape = null; //图形
private Line line = new Line(); //线
private Oval oval = new Oval(); //圆形
private Rectangle rectangle = new Rectangle(); //矩形
/**
* 设置窗口属性
*/
public MyFrame() {
this.setTitle("*****绘图窗口*****"); //标题
this.setSize(800,700); //大小
this.setResizable(false); //是否能调整大小
this.setLocationRelativeTo(null); //居中
this.setDefaultCloseOperation(EXIT_ON_CLOSE); //关闭窗口时结束程序
lineButton = new JButton("Line"); //命名画线按钮为“Line”
ovalButton = new JButton("oval"); //命名画圆按钮为“oval”
rectangleButton = new JButton("rectangle");
//*命名画矩按钮为“rectangle”
ActionListener haander = new ButtonHander();
lineButton.addActionListener(haander);
ovalButton.addActionListener(haander);
rectangleButton.addActionListener(haander);
this.setLayout(new FlowLayout());
/**
* 添加按钮
*/
this.add(lineButton);
this.add(ovalButton);
this.add(rectangleButton);
}
@Override
public void paint(Graphics g) { //在窗口用画笔绘制
super.paint(g);
if (shape!= null) {
shape.draw(g);
double a = Math.abs(shape.getX1() - shape.getX2());//横坐标之差
double b = Math.abs(shape.getY1() - shape.getY2());//纵坐标之差
if (shape == line) {
int L = (int) Math.sqrt(a * a + b * b);
g.drawString("线条长度:"+ L, 100, 100);
}
if (shape == oval) {
int c = (int) (( Math.PI * a<b?a:b) + 4 * Math.abs(a/2 - b/2) );
int s = (int) (Math.PI * a * b / 4);
g.drawString("椭圆周长:"+ c, 100, 100);
g.drawString("椭圆面积:"+ s, 100, 120);
}
if (shape == rectangle) {
int s = (int) (a * b) ;
int c = (int) ((a + b) * 2);
g.drawString("矩形面积:"+ s, 100, 100);
g.drawString("矩形周长:"+ c, 100, 120);
}
}
}
}
package mt.tese;
import mt.iu.MyFrame;
public class Test {
public static void main(String[] args) {
new MyFrame().setVisible(true); //匿名对象
}
}
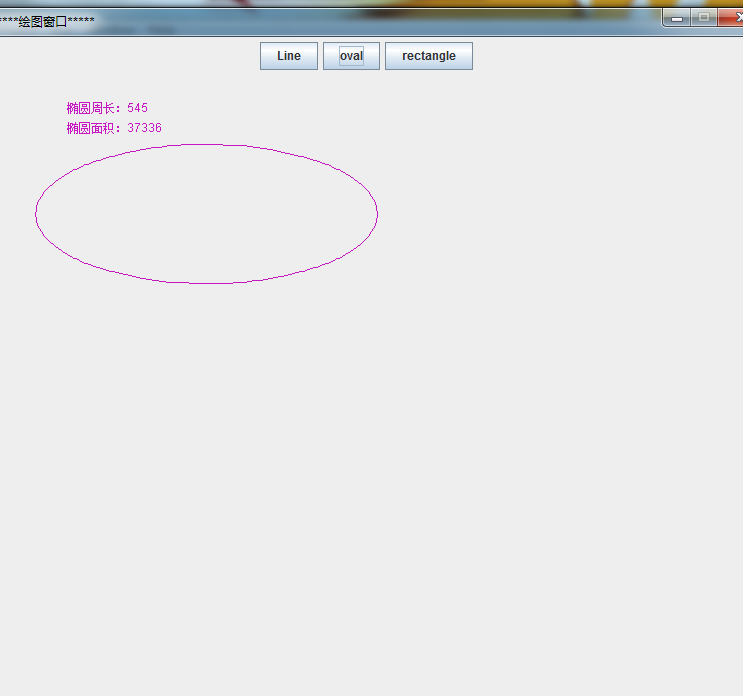