Understanding the JavaScript Engine — Part 1

I have been a Ruby on Rails developer for the last 2 years. I have used JavaScript both in its vanilla form and in some frameworks. However, I learned JavaScript as most new programmers do, by going through a course, without quite understanding how the JavaScript engine works.
Before diving deep into JavaScript, I decided to take some time off and understand its core working principles. I’ll be sharing what I’ve learned so far in this, and subsequent blog posts.
First, let me define some terms you’ll come across. I’ll add examples where necessary.
Syntax Parser
When you write code, a compiler converts your code into a set of instructions that the computer can understand. Part of the compiler is what is known as a syntax parser. The syntax parser goes through your code character by character, and determines if the syntax is valid or not.
Lexical Environment
In simple terms, a Lexical environment refers to where something sits physically in your code. Where something is written gives an idea of how the computer will interpret it and how it will interact with other variables and functions e.g
function hello() { var greet = "Hello world" }
In the function above, we can say that var greet
sits lexically in the function.
Execution Context
This is a wrapper that helps manage the code that is running. Looking at the gif below, you can see there’s an execution context stack and in it, we have a Global execution context. When functionA()
is called, it is added to the Stack meaning that functionA()
is currently being executed. The same goes for functionB()
.
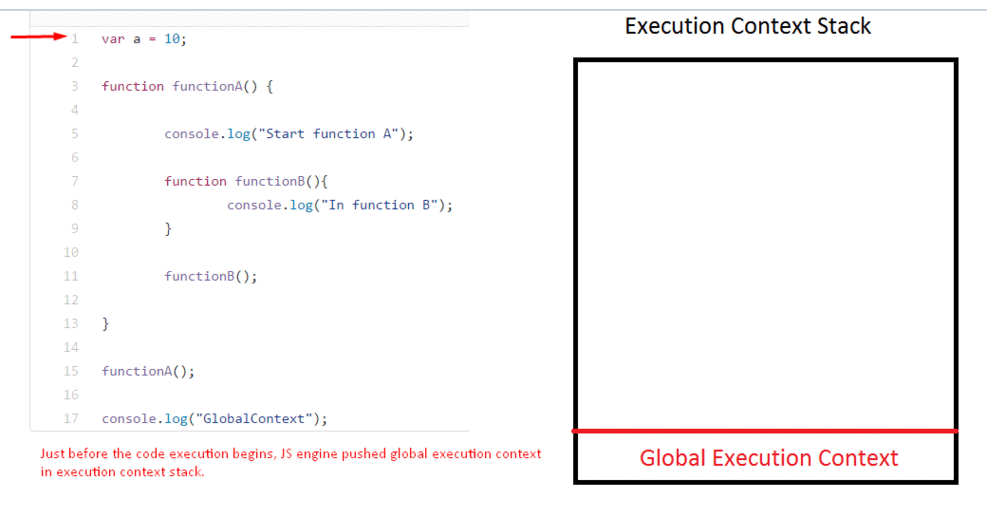
The execution context is created in two phases:
The first phase is called the creation phase. The global execution context creates two things for you, that you don’t have in your code; a global object(window)
and a special variable called this
. The window
object is a global object inside a browser. This object is different depending on whether you are using node or running JavaScript on the server. But there is always a global object when you’re running JavaScript. Take a look at the following image from a browser console:
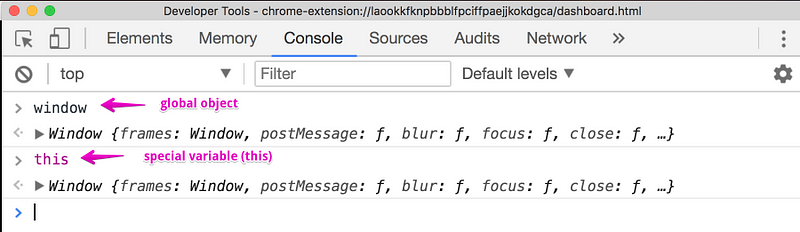
When you create a variable and function that is not inside a function, those variables and functions get attached to the global object.
var name = "Debby"; function greet() { console.log("Hello", name) }
If you run the above JavaScript code in the browser and you inspect the global object, you will see that the variable and the function were added to it.
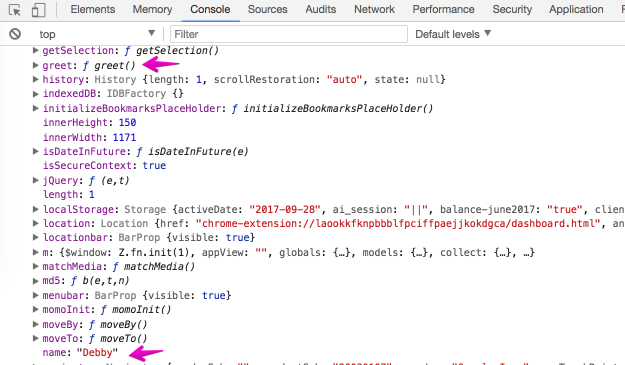
During the creation phase, the syntax parser recognizes where you have defined variables and functions. It therefore sets up memory space for the variables and functions. It’s not actually moving code to the top of the page. What this means is that before your code begins to be executed line by line, the JavaScript engine has already set aside memory space for the variables and functions that you’ve written. This is what is called Hoisting.
The next phase is the execution phase, where assignments are made. When the JavaScript engine sets up memory space for variables, it doesn’t know which values will be stored in them. Therefore, it puts a placeholder called undefined
. That placeholder means; I don’t know what this value is yet. All variables in JavaScript are initially set to undefined
while functions sit in memory in their entirety. This is why it’s possible to declare a variable without assigning it and the JavaScript engine will not throw any error.
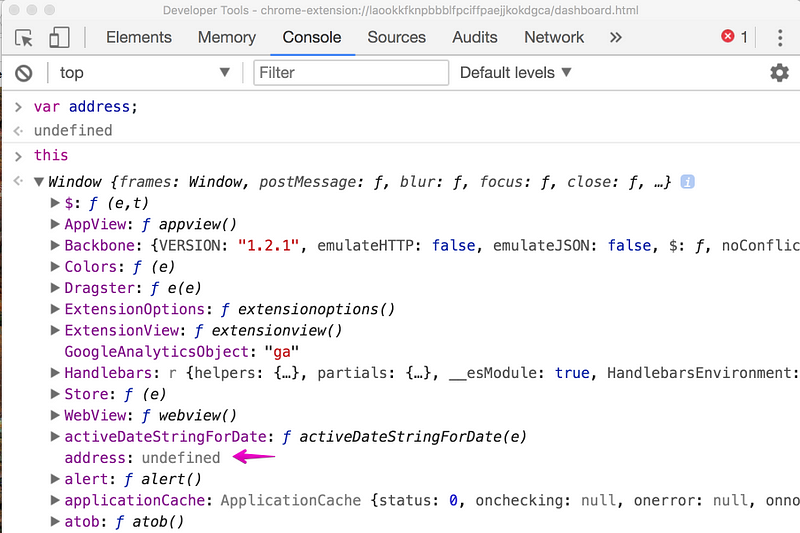
This is just a brief introduction to how the JavaScript engine executes the code you write. To know more, you can use the resources below:
JavaScript: Understanding the Weird Parts by Anthony Alicea on Udemy.Execution context, Scope chain and JavaScript internals by Rupesh Mishra.