知识点:创建多线程方式、线程的常用方法、线程的生命周期
一:创建线程和启动
(1)extend继承Thread类,创建线程类
//定义一个Thread的子类,重写run()方法
public class Window extends Thread {
@Override
public void run() {}
}
public class WindowTest {
public static void main(String[] args) {
Window w1=new Window(); //创建子类Window的实例
w1.setName("子线程1");
w1.start(); //调用start()方法启动线程
Window w2=new Window();
w2.setName("子线程2");
w2.start();
}
}
(2)implements实现Runnable接口,创建线程类
//定义一个Thread的实现类,重写run()方法
public class Window2 implements Runnable {
@Override
public void run() {
}
}
public class WindowTest {
public static void main(String[] args) {
Window2 window2=new Window2(); //创建子类Window2的实例
Thread th1=new Thread(window2);//将Window2实例作为Thread的target,那么th1成为真正的线程对象
th1.setName("窗口1");
Thread th2=new Thread(window2);
th2.setName("窗口2");
th1.start();//调用start()方法启动线程
th2.start();
}
}
(3)implements实现Callable接口,创建线程类,较Runnable接口,增加了返回值和异常
关于callable接口的详细解释可参考https://www.jianshu.com/p/034604c6f6f5
public class CallableTest {
public static void main(String[] args) {
Task task=new Task();
FutureTask<Integer> futureTask=new FutureTask<>(task);//创建FutureTask类的实例,将task作为参数传入参数中
Thread thread=new Thread(futureTask);//将futureTask作为参数传入线程target中
thread.start();
int result=0;
try {
result=futureTask.get();//get方法获取implement Callable接口的call方法的返回值
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
System.out.println("执行结果result:"+result);
}
}
class Task implements Callable {
@Override
public Integer call() throws Exception {
System.out.println("运行Call()方法");
int result=0;
for(int i=0;i<10;i++){
result=result+i;
}
return result;
}
}
运行结果:
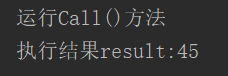
FutureTask是实现RunnableFuture接口,RunnableFuture是继承Runnable和Future接口的,Future用来获取任务结果或者中断任务的
补充:继承和implements两种方式的比较:
a.都实现了 Runnable 方法,public class Thread implements Runnable
b.implements的实现方式要优于继承的方式
(1) 避免了java单继承的局限性
(2) 多线程要操作同一份资源(或者数据),implements实现的方式更适合些(只需new 一个对象,把这个对象作为Thread的构造方法的参数)
二:线程的生命周期
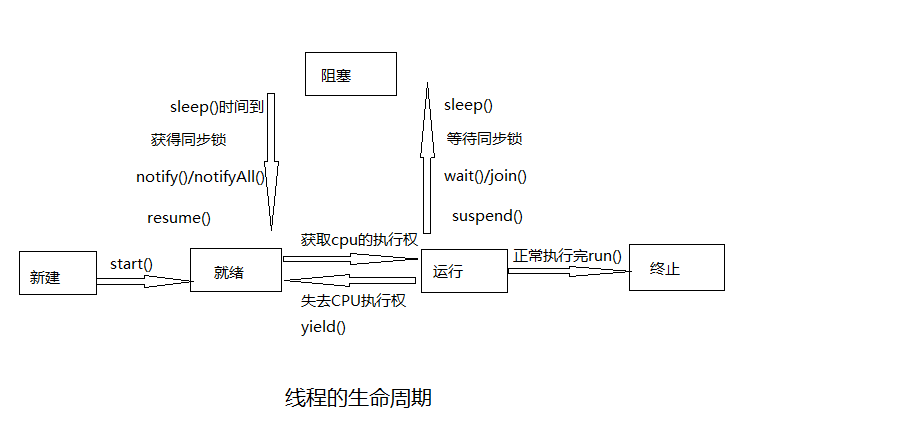
三:线程的常用方法
Thread的常用方法
1.start():启动该线程并执行相应的run()方法
2.run方法:子线程要执行的代码放到run()方法中
3.currentThread():静态的,调取当前的线程
4.getName():获取线程的名字
5.setName():设置此线程的名字
6.yeild():调用此方法的线程释放当前CPU的执行权
7.join():在A线程中,调用B线程的join()方法,表示:当执行到此方法,A线程停止执行,
直到B线程执行完毕,A线程再接着执行join()之后的代码
8.isAlive():判断当前线程是否存活
9.sleep(long l):显示地让当前线程睡眠l 毫秒
10.线程间通信:wait() notify() notifyAll()
11.设置线程的优选级
getPriority():返回线程优先级
setPriority(int NewPriority):设置线程的优先级