原文:http://www.jianshu.com/p/ef3f1731a353
如果我们要遍历一个数组, 上过编程课程的童鞋都会想到For语句去循环.
Objective C 提供一个Block的遍历方法, 那么用它还是用For好呢?
遍历一个数组看谁快
参赛选手 ForLoop
, For - in
, enumerateObjectsUsingBlock
这个三个方法:
NSMutableArray *test = [NSMutableArray array];
for (int i = 0; i < 1000000; i ++) {
[test addObject:@(i)];
}
__block int sum = 0;
double date_s = CFAbsoluteTimeGetCurrent();
for (int i = 0; i < test.count; i ++) {
sum += [test[i] integerValue];
}
double date_current = CFAbsoluteTimeGetCurrent() - date_s;
NSLog(@"Sum : %d ForLoop Time: %f ms",sum,date_current * 1000);
sum = 0;
date_s = CFAbsoluteTimeGetCurrent();
for (NSNumber *num in test) {
sum += [num integerValue];
}
date_current = CFAbsoluteTimeGetCurrent() - date_s;
NSLog(@"Sum : %d For-in Time: %f ms",sum,date_current * 1000);
sum = 0;
date_s = CFAbsoluteTimeGetCurrent();
[test enumerateObjectsUsingBlock:^(id obj, NSUInteger idx, BOOL *stop) {
sum += [obj integerValue];
}];
date_current = CFAbsoluteTimeGetCurrent() - date_s;
NSLog(@"Sum : %d enumrateBlock Time: %f ms",sum,date_current * 1000);
最后输出如下:

-
For-in方法最快速
结论:
当只是遍历一个数组的时候使用For-in会比较快速, 推荐使用For-in遍历数组.
通过Value查找Index看谁快
假如现在我们要查找一个Value, 这个Value 值是100001, 找出它的index (数组的序列号).
那么现在我们来比较一下
参赛选手 For - in
, enumerateObjectsUsingBlock
, enumerateObjectsWithOptions
这个三个方法: (ForLoop已经不再继续讨论了)
NSMutableArray *test = [NSMutableArray array];
for (int i = 0; i < 10000000; i ++) {
[test addObject:@(i + 10)];
}
//For-in
__block NSInteger index = 0;
double date_s = CFAbsoluteTimeGetCurrent();
for (NSNumber *num in test) {
if ([num integerValue] == 9999999) {
index = [test indexOfObject:num];
break;
}
}
double date_current = CFAbsoluteTimeGetCurrent() - date_s;
NSLog(@"index : %ld For-in Time: %f ms",(long)index,date_current * 1000);
//enumerateObjectsUsingBlock
index = 0;
date_s = CFAbsoluteTimeGetCurrent();
[test enumerateObjectsUsingBlock:^(id num, NSUInteger idx, BOOL *stop) {
if ([num integerValue] == 9999999) {
index = idx;
*stop = YES;
}
}];
date_current = CFAbsoluteTimeGetCurrent() - date_s;
NSLog(@"index : %ld enumerateBlock Time: %f ms",(long)index,date_current * 1000);
//enumerateObjectsWithOptions
index = 0;
date_s = CFAbsoluteTimeGetCurrent();
[test enumerateObjectsWithOptions:NSEnumerationConcurrent usingBlock:^(id num, NSUInteger idx, BOOL *stop) {
if ([num integerValue] == 9999999) {
index = idx;
*stop = YES;
}
}];
date_current = CFAbsoluteTimeGetCurrent() - date_s;
NSLog(@"index : %ld enumerateObjectsWithOptions Time: %f ms",(long)index,date_current * 1000);
最后输出如下图:

-
enumerateObjectsWithOptions方法最快速
结论:
通过Value查询index的时候, 面对大量的数组推荐使用enumerateObjectsWithOptions
的并行方法.For-in
和enumerateObjectsWithOptions
方法这里我比较喜欢第二种写法简洁直观.
现在咱们要遍历字典
这里我们比较一下使用 For-in
和 enumerateKeysAndObjectsUsingBlock
这个两个方法:
NSDictionary *testDictionary = @{
@"Auther" : @"糖醋排骨",
@"blog" : @"http://www.cnblogs.com/tate-zwt/",
@"name" : @"zwt"
};
//For - in
NSMutableArray *forInArry = [NSMutableArray array];
double date_s = CFAbsoluteTimeGetCurrent();
NSArray *keys = [testDictionary allKeys];
for (NSString *key in keys) {
NSString *Value = testDictionary[key];
[forInArry addObject:Value];
}
double date_current = CFAbsoluteTimeGetCurrent() - date_s;
NSLog(@"index : %ld For-in Time: %f ms",(long)index,date_current * 1000);
//enumerateKeysAndObjectsUsingBlock
date_s = CFAbsoluteTimeGetCurrent();
NSMutableArray *enumArry = [NSMutableArray array];
[testDictionary enumerateKeysAndObjectsUsingBlock:^(id key, id obj, BOOL *stop) {
[enumArry addObject:obj];
}];
date_current = CFAbsoluteTimeGetCurrent() - date_s;
NSLog(@"index : %ld
enumerateKeysAndObjectsUsingBlock
Time: %f ms",(long)index,date_current * 1000); NSLog(@"ForInArr: %@",forInArry); NSLog(@"enumArry: %@",enumArry);
最后输出如下图:
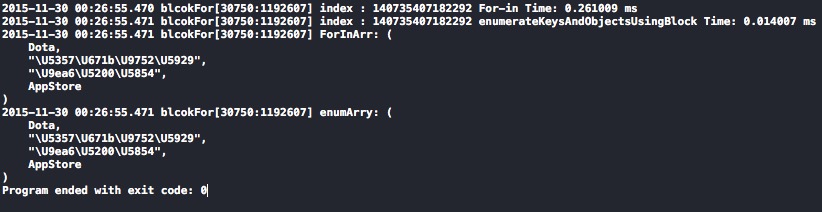
-
enumerateKeysAndObjectsUsingBlock胜出
结论:
当我们想遍历字典类型的时候, 推荐使用enumerateKeysAndObjectsUsingBlock
不仅仅是因为速度快, 更是因为代码更优雅和直观.