抄书 (二分查找+贪心)
提示:二分查找一般写成非递归形式
时间复杂度:O(logn)
题目链接:http://acm.hust.edu.cn/vjudge/contest/view.action?cid=85904#problem/B
Description
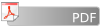
Before the invention of book-printing, it was very hard to make a copy of a book. All the contents had to be re-written by hand by so called scribers. The scriber had been given a book and after several months he finished its copy. One of the most famous scribers lived in the 15th century and his name was Xaverius Endricus Remius Ontius Xendrianus (Xerox). Anyway, the work was very annoying and boring. And the only way to speed it up was to hire more scribers.
Once upon a time, there was a theater ensemble that wanted to play famous Antique Tragedies. The scripts of these plays were divided into many books and actors needed more copies of them, of course. So they hired many scribers to make copies of these books. Imagine you have m books (numbered ) that may have different number of pages (
) and you want to make one copy of each of them. Your task is to divide these books among k scribes,
. Each book can be assigned to a single scriber only, and every scriber must get a continuous sequence of books. That means, there exists an increasing succession of numbers
such that i-th scriber gets a sequence of books with numbers between bi-1+1 and bi. The time needed to make a copy of all the books is determined by the scriber who was assigned the most work. Therefore, our goal is to minimize the maximum number of pages assigned to a single scriber. Your task is to find the optimal assignment.
Input
The input consists of N cases. The first line of the input contains only positive integer N. Then follow the cases. Each case consists of exactly two lines. At the first line, there are two integers m and k, . At the second line, there are integers
separated by spaces. All these values are positive and less than 10000000.
Output
For each case, print exactly one line. The line must contain the input succession divided into exactly k parts such that the maximum sum of a single part should be as small as possible. Use the slash character (`/') to separate the parts. There must be exactly one space character between any two successive numbers and between the number and the slash.
If there is more than one solution, print the one that minimizes the work assigned to the first scriber, then to the second scriber etc. But each scriber must be assigned at least one book.
Sample Input
2
9 3
100 200 300 400 500 600 700 800 900
5 4
100 100 100 100 100
Sample Output
100 200 300 400 500 / 600 700 / 800 900 100 / 100 / 100 / 100 100
题意:
输入多组案例T,给你N本书,一个有M个人抄写,使M个人所抄写书所用的时间最少,输出分配方案。(1<=N<=100000,1<=M<=N,每个数在1到10000之间) 如果有多种可能的话,尽量在前面进行划分。
分析:
1.经典的最大值最小化问题,采用 贪心+二分查找
2.输出的时候用到贪心的思想,既尽量往前进行划分
3.利用二分查找找出满足题意的最大值,再利用贪心从后往前的方式划分成段,如果剩余可划分段与i+1的值相等(尽量靠前),则将剩余的段往前划分
代码:

1 #include <cstdio> 2 #include <iostream> 3 #include <cstring> 4 using namespace std; 5 const int MAXN = 501; 6 7 int n, m; 8 int p[MAXN], use[MAXN]; //标记数组use表示是否划分 9 long long low_bound, high_bound; //由于high_bound可能很多,用long long 来存储 10 11 12 void init() 13 { 14 low_bound=-1; 15 high_bound=0; 16 memset(use,0,sizeof(use)); 17 } 18 19 int solve(int mid) //判断最大值所在区间是左还是右 20 { 21 int sum=0,group=1; 22 for(int i=n-1;i>=0;i--) 23 { 24 if(sum+p[i]>mid) 25 { 26 sum=p[i]; 27 group++; 28 if(group>m) //看可以分成几组,若group>m,舍弃 29 return 0; 30 } 31 else sum+=p[i]; 32 } 33 return 1; 34 } 35 36 void print(int high_bound) 37 { 38 int group=1,sum=0; 39 for(int i=n-1;i>=0;i--) 40 { 41 if(sum+p[i]>high_bound) //从右边开始确定划分 42 { 43 use[i]=1; 44 sum=p[i]; 45 group++; 46 } 47 else sum+=p[i]; 48 if(m-group==i+1) 49 { 50 for(int j=0;j<=i;j++) 51 use[j]=1; 52 break; 53 } 54 } 55 for(int i=0;i<n-1;i++) 56 { 57 printf("%d ",p[i]); 58 if(use[i]) //确定‘/’的位置 59 printf("/ "); 60 } 61 printf("%d ",p[n-1]); 62 } 63 64 int main() 65 { 66 int T; 67 scanf("%d",&T); 68 while(T--) 69 { 70 init(); 71 scanf("%d%d",&n,&m); 72 for(int i=0;i<n;i++) 73 { 74 scanf("%d",&p[i]); 75 if(low_bound<p[i]) 76 low_bound=p[i]; 77 high_bound+=p[i]; 78 } 79 long long x=low_bound,y=high_bound; 80 while(x<=y) //二分,判断最小的最大值的区间 81 { 82 long long mid=x+(y-x)/2; 83 if(solve(mid)) 84 y=mid-1; 85 else x=mid+1; 86 } 87 print(x); 88 } 89 return 0; 90 }
之前上选修课的时候听过贪心算法,但之前没有做过类似的题,感觉做的不是很好。