Win32 程序开发
新建 Win32 Application
MessageBox,按F1,查看函数说明。
MessageBox The MessageBox function creates, displays, and operates a message box. The message box contains an application-defined message and title, plus any combination of predefined icons and push buttons. int MessageBox( HWND hWnd, // handle of owner window LPCTSTR lpText, // address of text in message box LPCTSTR lpCaption, // address of title of message box UINT uType // style of message box );
Flag Meaning MB_ABORTRETRYIGNORE The message box contains three push buttons: Abort, Retry, and Ignore. 消息框包含三个按钮:中止、重试和忽略。 MB_OK The message box contains one push button: OK. This is the default. 消息框包含一个按钮:OK。这是默认设置。 MB_OKCANCEL The message box contains two push buttons: OK and Cancel. 消息框包含两个按钮:OK和Cancel。 MB_RETRYCANCEL The message box contains two push buttons: Retry and Cancel. 消息框包含两个按钮:retry和cancel。 MB_YESNO The message box contains two push buttons: Yes and No. MB_YESNOCANCEL The message box contains three push buttons: Yes, No, and Cancel.
MB_RETRYCANCEL右键,转到定义,或者按F12
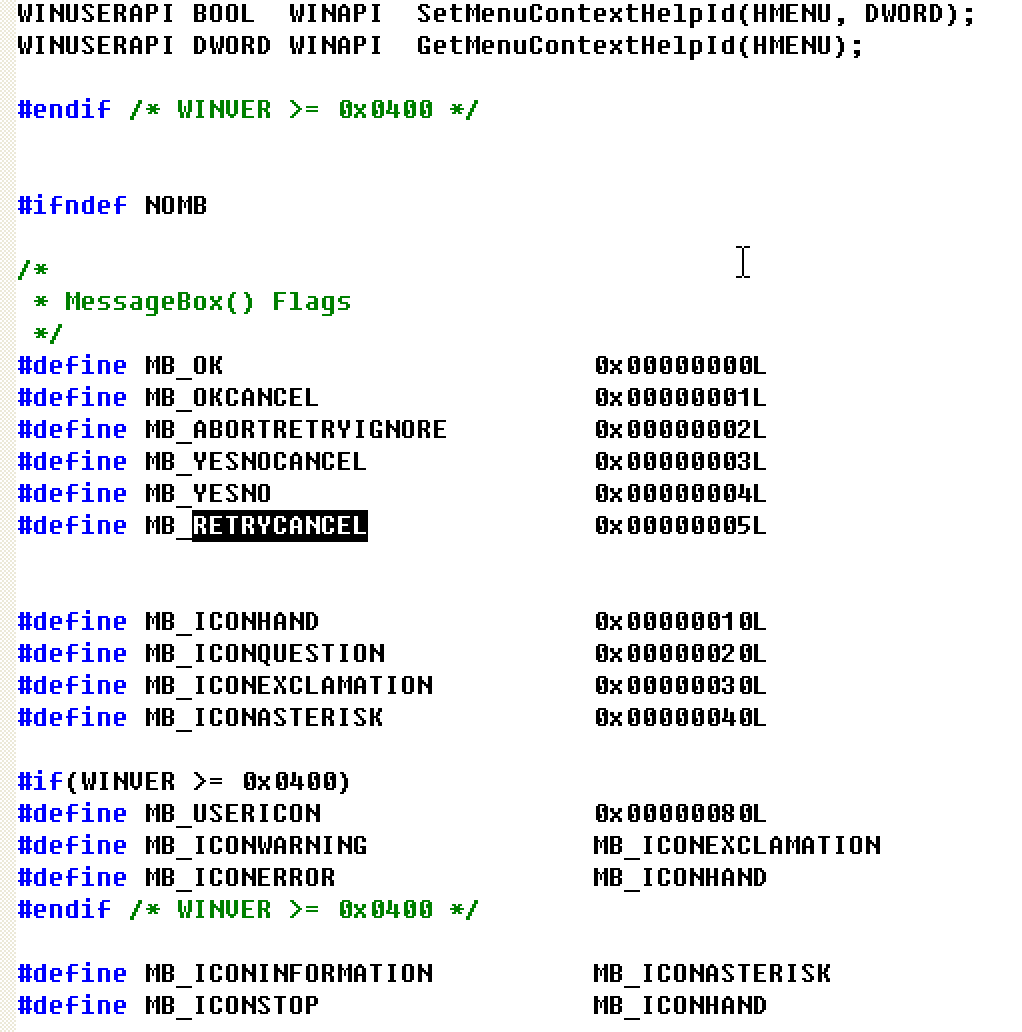
8个位一个字节,16个位2个字节
一个位存储的只能是0或者1,一个字节的变量值是0到255之间。
Return Values
返回值部分
Return Values The return value is zero if there is not enough memory to create the message box. If the function succeeds, the return value is one of the following menu-item values returned by the dialog box: Value Meaning IDABORT Abort button was selected. IDCANCEL Cancel button was selected. IDIGNORE Ignore button was selected. IDNO No button was selected. IDOK OK button was selected. IDRETRY Retry button was selected. IDYES Yes button was selected.
ID的数值,按钮的编号
#include "stdafx.h" int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { // TODO: Place code here. int nRet = MessageBox(NULL,"这是我开发的第一个windows软件!","温馨提示",MB_OKCANCEL|MB_ICONEXCLAMATION); if(nRet==IDOK) MessageBox(NULL,"你点击了OK按钮","返回值",0); else MessageBox(NULL,"你点击了Cancel按钮","返回值",1); return 0; }
按F10开始调试,
#include "stdafx.h" int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { // TODO: Place code here. /* int nRet = MessageBox(NULL,"这是我开发的第一个windows软件!","温馨提示",MB_OKCANCEL|MB_ICONEXCLAMATION); if(nRet==IDOK) MessageBox(NULL,"你点击了OK按钮","返回值",0); else MessageBox(NULL,"你点击了Cancel按钮","返回值",1); */ HWND hwnd = ::FindWindow(NULL,"无标题 - 记事本"); int nRet = MessageBox(hwnd,"这是我开发的第一个windows软件!","温馨提示",MB_OKCANCEL|MB_ICONEXCLAMATION); if(nRet==IDOK) MessageBox(hwnd,"你点击了OK按钮","返回值",0); else MessageBox(hwnd,"你点击了Cancel按钮","返回值",1); return 0; }
HWND hWnd, // handle of owner window
附着于某些窗口作为子窗口
/////////////////////////////////////////////////
File➡️New命令,添加资源
选中Resource Script ,填写资源文件名,与工程名相同。
Workspace区域中多了一个ResourceView分页,资源视图。
插入
图标资源
画图标,然后F7编译
工程目录下,增加resource .h文件 .rc文件。
打开resource .h
图标的编号是101,执行程序适用ID最小的图标,作为应用程序的图标。
电脑搜索*.ico
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
对话框
int DialogBox( HINSTANCE hInstance, // handle to application instance LPCTSTR lpTemplate, // identifies dialog box template HWND hWndParent, // handle to owner window DLGPROC lpDialogFunc // pointer to dialog box procedure );
int DialogBox(
int DialogBox
HINSTANCE hInstance, // handle to application instance
hinstance hinstance,//处理应用程序实例
LPCTSTR lpTemplate, // identifies dialog box template
lpctstr lptemplate,//标识对话框模板,string类型的字符串,利用模版的ID
模版的ID都是1⃣️数字的形式存在的(VC6.0都是数字形式,以前是字符串形式)
但是要求代入的是一个字符串,强制转化为旧的模式。
第二个参数:
(LPCTSTR)IDD_DIALOG1
第一个参数,
HINSTANCE hInstance, // handle to application instance
hinstance hinstance,//处理应用程序实例
application instance,进程句柄,就是ResourcesView内的所有内容,包括图标光标。程序运行的时候,加载对话框,加载图标,光标。程序运行的时候,都存储在hInstance中。
加载对话框,加载图标,光标,都要指定程序的句柄,才能加载资源。
程序启动时,会在加载这个程序之后,当你告诉操作系统之后,操作系统加载了整个的exe可执行文件,在执行文件exe中又提取出所有的rc,这些rc统统打入hInstance中。
第二个参数,在进程句柄中所有的范围内,选择对话框的ID进行加载。
第三个参数,代入NULL。自己就是主窗口,不附着于其他的窗口。
第四个参数,回调函数。
HWND hWndParent, // handle to owner window
hwnd hwndparent,//句柄到所有者窗口,需要制定owner window,查找到一个窗口,附着于某个窗口。如果为空,自己就是主窗口。代入某个窗口的句柄,就会附着于某个窗口。
DLGPROC lpDialogFunc // pointer to dialog box procedure
dlgproc lpdialogfunc//指向对话框过程的指针
);
;
lpDialogFunc 回调函数,在DOS,控制台软件中很少使用的一种回调函数的方式。
BOOL CALLBACK DialogProc( HWND hwndDlg, // handle to dialog box UINT uMsg, // message WPARAM wParam, // first message parameter LPARAM lParam // second message parameter );
DialogProc函数名,随便起一个函数名,比如,MainProc,(ClassView中会看到增加了一个函数),把函数名放进来,把函数的地址传给DialogBox。
(所有在windows程序中调用的函数,无论是Findwindow,MessageBox,DialogBox,实际上,把这些参数还是要传给操作系统,操作系统执行真正的功能。)

宏定义不存在,在resources.h中,需要包含 #include "resourse.h"
#include "stdafx.h" #include "resource.h" BOOL CALLBACK MainProc( HWND hwndDlg, // handle to dialog box UINT uMsg, // message WPARAM wParam, // first message parameter LPARAM lParam // second message parameter ) { return FALSE; } int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { DialogBox(hInstance,(LPCSTR)IDD_DIALOG1,NULL,MainProc); return 0; }
消息回调函数是干嘛的?
按F9设置断点,不停的按F5,会不停的传入一些数据,反复的进来。这叫消息回调函数。无论在对话框中输入数字还是点击按钮,都会被这个函数所截获,然后处理。
BOOL CALLBACK DialogProc(
HWND hwndDlg, // handle to dialog box
本对话框句柄
UINT uMsg, // message
消息的号码(种类),双击,按钮,移动鼠标等,一种消息机制。
WPARAM wParam, // first message parameter
窗口消息的相关数据,如单击按钮。
LPARAM lParam // second message parameter
);
窗口消息的相关数据,如单击按钮。为了防止一个数据不够用。
UINT,按住F12查看
无符号整型变量
做一个简单测试。
有个函数专门用于程序运行期间,在Debug输出窗口中,输出程序运行过程中的变化,
OutputDebugString()
OutputDebugString("测试运行状态 ");
代表换行,编译,按F5,以调试模式运行。
把 uMsg ,wParam打印出来,s字符串数组变量。把程序运行中的数据打印到字符串中。sprintf是把变量打印到字符串数组中
#include "stdafx.h" #include "resource.h" #include <stdio.h> BOOL CALLBACK MainProc( HWND hwndDlg, // handle to dialog box UINT uMsg, // message WPARAM wParam, // first message parameter LPARAM lParam // second message parameter ) { char s[256]; sprintf(s,"uMsg=%d,wParam=%d,lParam=%d ",uMsg,wParam,lParam); OutputDebugString(s); return FALSE; } int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { DialogBox(hInstance,(LPCSTR)IDD_DIALOG1,NULL,MainProc); return 0; }
error C2065: 'sprintf' : undeclared identifier,所以加上#include <stdio.h>
测试消息的分流,消息的识别。
当uMsg等于命令操作的时候
WM_COMMAND ,Windows Message,WM开头就是一个消息种类
WM_COMMAND
wNotifyCode = HIWORD(wParam); // notification code
wID = LOWORD(wParam); // item, control, or accelerator identifier
hwndCtl = (HWND) lParam; // handle of control
Parameters
wNotifyCode
Value of the high-order word of wParam. Specifies the notification code if the message is from a control. If the message is from an accelerator, this parameter is 1. If the message is from a menu, this parameter is 0.
wID
Value of the low-order word of wParam. Specifies the identifier of the menu item, control, or accelerator.
hwndCtl
Value of lParam. Handle to the control sending the message if the message is from a control. Otherwise, this parameter is NULL.
如果这个消息是来自点击一个点击按钮产生的,就会把句柄记录在lParam中,否则是点击一个菜单项或者按一个快捷键,那么产生的这个消息,lParam记录的值是零。
再看wParam,分为HIWORD,LOWORD两部分。
wParam,lParam都是四个字节的变量。wParam中高两个字节时HIWORD,低两个字节是LOWORD
(notification通知)当你按下一个快捷键时,产生的WM_COMMAND消息,在高两位字节上记录1,当点击按钮,在高两位字节上记录0
LOWORD,无论是菜单项还是窗口的按钮,还是一个快捷键,三种类型都有一个编号,按钮的编号,菜单项的编号.
// 20190331.cpp : Defines the entry point for the application. // #include "stdafx.h" #include "resource.h" #include <stdio.h> BOOL CALLBACK MainProc( HWND hwndDlg, // handle to dialog box UINT uMsg, // message WPARAM wParam, // first message parameter LPARAM lParam // second message parameter ) { char s[256]; sprintf(s,"uMsg=%d,wParam=%d,lParam=%d ",uMsg,wParam,lParam); OutputDebugString(s); if(WM_COMMAND==uMsg) { if(LOWORD(wParam)==IDCANCEL) { EndDialog(hwndDlg,IDCANCEL); return TRUE; } if(LOWORD(wParam)==IDOK) { MessageBox(hwndDlg,"测试了计算","测试",0); } } return FALSE; } int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { DialogBox(hInstance,(LPCSTR)IDD_DIALOG1,NULL,MainProc); return 0; }
插入断点,点击计算按钮,弹出消息框
点击关闭按钮,wParam值为2.
消息类型没有变化,都是273,但是消息ID发生了变化
16进制的111,就是273
现在要用一个函数,来获取Edit中的变量数值,
GetDlgItemInt这个函数,有四个参数。指定对话框的句柄,从对话框中的某一个控件中获取,
第一个是对话框的句柄,第二个是这个控件的ID,
查看帮助
GetDlgItemInt The GetDlgItemInt function translates the text of a specified control in a dialog box into an integer value. UINT GetDlgItemInt( HWND hDlg, // handle to dialog box int nIDDlgItem, // control identifier BOOL *lpTranslated, // points to variable to receive success/failure // indicator BOOL bSigned // specifies whether value is signed or unsigned ); Parameters
hDlg
Handle to the dialog box that contains the control of interest.
nIDDlgItem
Dialog item identifier that specifies the control whose text is to be translated.
lpTranslated
Pointer to a Boolean variable that receives a function success/failure value. TRUE indicates success, FALSE indicates failure.
This parameter is optional: it can be NULL. In that case, the function returns no information about success or failure.
bSigned
Specifies whether the function should examine the text for a minus sign at the beginning and return a signed integer value if it f inds one. TRUE specifies that this should be done, FALSE that it should not.
把文字转成数值类型
第三个参数,返回型参数,也叫输出型参数。指向一个布尔型变量,用来接受是否成功还是失败。一般代入NULL
第四个参数,有符号变量在translates过程中,把负号取出来,无符号变量在遇见负号时无法识别。
int nLeft = GetDlgItemInt(hwndDlg,IDC_LEFT,NULL,TRUE);
设置断点,编译,F5以调试模式运行,点击计算,F10单步执行。
如果是FALSE,负数的时候,就不可识别,当0处理。
Get和Set函数
一个用于获取,一个用于输出。
SetDlgItemInt(hwndDlg,IDC_RESULT,nResult,TRUE);
#include "stdafx.h" #include "resource.h" #include <stdio.h> BOOL CALLBACK MainProc( HWND hwndDlg, // handle to dialog box UINT uMsg, // message WPARAM wParam, // first message parameter LPARAM lParam // second message parameter ) { char s[256]; sprintf(s,"uMsg=%d,wParam=%d,lParam=%d ",uMsg,wParam,lParam); OutputDebugString(s); if(WM_COMMAND==uMsg) { if(LOWORD(wParam)==IDCANCEL) { EndDialog(hwndDlg,IDCANCEL); return TRUE; } if(LOWORD(wParam)==IDOK) { //MessageBox(hwndDlg,"测试了计算","测试",0); int nLeft = GetDlgItemInt(hwndDlg,IDC_LEFT,NULL,TRUE); int nRight = GetDlgItemInt(hwndDlg,IDC_RIGHT,NULL,TRUE); int nResult = nLeft + nRight; SetDlgItemInt(hwndDlg,IDC_RESULT,nResult,TRUE); } } return FALSE; } int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { DialogBox(hInstance,(LPCSTR)IDD_DIALOG1,NULL,MainProc); return 0; }
Windows数据类型
简单的Windows数据类型
再介绍一些指针变量,例如
PINT p = 一个int类型的变量
PINT p = &hCmdShow;
加P或者LP效果几乎一样
字符串指针变量
PSTR str = "sdjiuh";
是一个指针,指向一个字符串的地址
当一个win32程序一启动的时候,这里传入几种变量类型。
第一个hInstance,句柄类型,容纳了所有在资源视图ResourceView中出现的资源,都被这个句柄所包含了。
要加载对话框,就要使用这个句柄hInstance,它里面再去找对话框的ID,比如从5个对话框中找一个ID,那么这5个对话框都要从hInstance中去获取。
第二个参数,前一个进程的句柄,一般没啥用。
第三个参数,一般是用来传命令的,它是一个字符串,既然是字符串,就可以用MessageBox的方式把它显示出来
MessageBox(NULL,lpCmdLine,"测试",0);
命令参数
在程序启动之前,给程序一些数据的通知。
第四个参数,nCmdShow,这个程序是明着启动还是暗着启动。
char s[256]; sprintf(s,"nCmdShow=%d",nCmdShow); MessageBox(NULL,lpCmdLine,"测试",0); DialogBox(hInstance,(LPCSTR)IDD_DIALOG1,NULL,MainProc); return 0;
启动
nCmdShow=1,
以最小化方式启动,nCmdShow=7
最大化启动,nCmdShow=3
后两个参数。。这就是程序启动前 意图的传入,传餐,命令行参数,显示模式
LPSTR str = "dscs";
LPCSTR s1 = "cdsv";
查看定义,一个是可写字符串,一个是只读。
typedef CHAR *LPSTR, *PSTR;
typedef CONST CHAR *LPCSTR, *PCSTR;
第二种,H开头的
HANDLE of Windows => HWND
HANDLE of Instance => HINSTANCE
HANDLE of Icon => HICON
Windows中定义了一系列的句柄类型,用于操作不同的Windows对象。句柄就是一个指针,指向一个被隐藏了内容的结构体的内存地址。
第三种,结构体类型
POINT,x,y坐标
SIZE,高宽尺寸
PECT,上下左右,矩形区域
窗口风格设置
控件风格设置
// 20190331.cpp : Defines the entry point for the application. // #include "stdafx.h" #include "resource.h" #include <stdio.h> BOOL CALLBACK LoginProc( HWND hwndDlg, // handle to dialog box UINT uMsg, // message WPARAM wParam, // first message parameter LPARAM lParam // second message parameter ) { char s[256]; sprintf(s,"LoginPro uMsg=%d,wParam=%d,lParam=%d ",uMsg,wParam,lParam); OutputDebugString(s); switch(uMsg) { case WM_COMMAND: { switch(LOWORD(wParam)) { case IDCANCEL: EndDialog(hwndDlg,IDCANCEL); return TRUE; case IDOK: { char sName[20]; char sPass[20]; GetDlgItemText(hwndDlg,IDC_NAME,sName,sizeof(sName)); GetDlgItemText(hwndDlg,IDC_PASS,sPass,sizeof(sPass)); if(0==strcmp("admin",sName) && 0==strcmp("123456",sPass)) EndDialog(hwndDlg,IDOK); else { MessageBox(hwndDlg,"用户名或密码错误","提示",MB_OK); } return TRUE; } } } } return FALSE; } BOOL CALLBACK MainProc( HWND hwndDlg, // handle to dialog box UINT uMsg, // message WPARAM wParam, // first message parameter LPARAM lParam // second message parameter ) { char s[256]; sprintf(s,"uMsg=%d,wParam=%d,lParam=%d ",uMsg,wParam,lParam); OutputDebugString(s); if(WM_COMMAND==uMsg) { if(LOWORD(wParam)==IDCANCEL) { EndDialog(hwndDlg,IDCANCEL); return TRUE; } if(LOWORD(wParam)==IDOK) { //MessageBox(hwndDlg,"测试了计算","测试",0); int nLeft = GetDlgItemInt(hwndDlg,IDC_LEFT,NULL,TRUE); int nRight = GetDlgItemInt(hwndDlg,IDC_RIGHT,NULL,TRUE); int nResult = nLeft + nRight; SetDlgItemInt(hwndDlg,IDC_RESULT,nResult,TRUE); } } return FALSE; } int APIENTRY WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) { int nRet = DialogBox(hInstance,(LPCSTR)IDD_LOGIN_DLG,NULL,LoginProc); if(nRet == IDCANCEL) return -1; DialogBox(hInstance,(LPCSTR)IDD_MAIN_DLG,NULL,MainProc); return 0; }
按住 ctrl,加D。
通过win32平台能够开发出所有需要的Windows软件,但是win32开发平台是极其原始而且落后的平台。它是基于C语言面向过程的开发模式。当C++语言流行后,随之而来的是面向对象的开发模式。因此,基于MFC平台的开发模式成为了Windows开发的主体。
基于MFC架构平台的对话框程序。
20190407Dlg.cpp文件中,修改对话框初始化函数。
BOOL CMy20190407Dlg::OnInitDialog() { CDialog::OnInitDialog(); CListCtrl* pList = (CListCtrl*)GetDlgItem(IDC_LIST); pList ->InsertColumn(0,"工号",LVCFMT_LEFT,120); pList ->InsertColumn(1,"姓名",LVCFMT_LEFT,120); pList ->InsertColumn(2,"工资",LVCFMT_LEFT,120);
再修改3个按钮关联的函数代码。
在添加的时候,获取列表控件的一个指针。首先从3个编辑框中获取3个数据,通过所获取到的列表控件指针,把数据作为一行数据插入到列表中去。
CListCtrl* pList = (CListCtrl*)GetDlgItem(IDC_LIST);
获取控件列表指针的函数,作为列表控件,取出这个窗口的指针,指针中含有这个控件窗口的句柄,有了控件的句柄,才能对列表插入数据。
三个按钮的函数代码:
void CMy20190407Dlg::OnAdd() { CString szNumb,szName,szSala; GetDlgItemText(IDC_NUMB,szNumb); GetDlgItemText(IDC_NAME,szName); GetDlgItemText(IDC_SALA,szSala); CListCtrl* pList = (CListCtrl*)GetDlgItem(IDC_LIST); int nCount = pList ->GetItemCount(); pList ->InsertItem(nCount,szNumb); pList ->SetItemText(nCount,1,szName); pList ->SetItemText(nCount,2,szSala); } void CMy20190407Dlg::OnDel() { CListCtrl* pList = (CListCtrl*)GetDlgItem(IDC_LIST); int nSel = pList ->GetSelectionMark(); if(nSel<0) { AfxMessageBox("请选择列表中的员工号码再删除!"); return; } pList ->DeleteItem(nSel); } void CMy20190407Dlg::OnMod() { CListCtrl* pList = (CListCtrl*)GetDlgItem(IDC_LIST); int nSel = pList ->GetSelectionMark(); if(nSel<0) { AfxMessageBox("请选择列表中的员工号码再修改!"); return; } CString szNumb,szName,szSala; GetDlgItemText(IDC_NUMB,szNumb); GetDlgItemText(IDC_NAME,szName); GetDlgItemText(IDC_SALA,szSala); pList ->SetItemText(nSel,0,szNumb); pList ->SetItemText(nSel,1,szName); pList ->SetItemText(nSel,2,szSala); }
或者
void CMy20190407Dlg::OnMod() { CListCtrl* pList = (CListCtrl*)GetDlgItem(IDC_LIST); //int nSel = pList ->GetSelectionMark(); POSITION pos = pList ->GetFirstSelectedItemPosition(); int nSel = pList ->GetNextSelectedItem(pos); if(nSel<0) { AfxMessageBox("请选择列表中的员工号码再修改!"); return; } CString str; GetDlgItemText(IDC_NUMB,szNumb); if(IDNO==AfxMessageBox("确定修改" + str +"的数据吗?",MB_YESNO)) return; GetDlgItemText(IDC_NAME,str); pList ->SetItemText(nSel,1,str); GetDlgItemText(IDC_SALA,str); pList ->SetItemText(nSel,2,str); }
新建一个MFC工程用于测试类成员函数,每个按钮对应测试一个类成员函数。
void CTestStrDlg::OnGetlength() { CString str; GetDlgItemText(IDC_LEFT ,str); int nLen = str.GetLength(); str.Format("长度=%d",nLen); SetDlgItemText(IDC_RESULT,str); } void CTestStrDlg::OnIsempty() { CString str; GetDlgItemText(IDC_LEFT ,str); BOOL b = str.IsEmpty(); if(b) SetDlgItemText(IDC_RESULT,"是空字符串"); else SetDlgItemText(IDC_RESULT,"不是空字符串"); } void CTestStrDlg::OnAdd() { CString szLeft,szRight; GetDlgItemText(IDC_LEFT ,szLeft); GetDlgItemText(IDC_RIGHT,szRight); SetDlgItemText(IDC_RESULT,szLeft+szRight); } void CTestStrDlg::OnEqual() { CString szLeft,szRight; GetDlgItemText(IDC_LEFT ,szLeft); GetDlgItemText(IDC_RIGHT,szRight); if(szLeft == szRight) SetDlgItemText(IDC_RESULT,"相等"); else SetDlgItemText(IDC_RESULT,"不相等"); } void CTestStrDlg::OnButton5() { CRect rect; GetWindowRect(&rect); }