概述
看了一个数据结构的教程,是用C++写的,可自己C#还是一个菜鸟,更别说C++了,但还是大胆尝试用C#将其中的环形队列的实现写出来,先上代码:
1 public class MyQueue<T> : IDisposable 2 { 3 private T[] queue; 4 private int length; 5 private int capacity; 6 private int head = 0; 7 private int tail = 0; 8 9 public MyQueue(int capacity) { 10 this.capacity = capacity; 11 this.head = 0; 12 this.tail = 0; 13 this.length = 0; 14 this.queue = new T[capacity]; 15 } 16 17 public void Clear() { 18 head = 0; 19 tail = 0; 20 length = 0; 21 } 22 23 public bool IsEmpty() { 24 return length == 0; 25 } 26 27 public bool IsFull() { 28 return length == capacity; 29 } 30 31 public int Length() { 32 return length; 33 } 34 35 public bool EnQueue(T node) { 36 if (!IsFull()) { 37 queue[tail] = node; 38 tail = (++tail) % capacity; 39 length++; 40 return true; 41 } 42 return false; 43 } 44 45 public T DeQueue() { 46 T node = default(T); 47 if (!IsEmpty()) { 48 node = queue[head]; 49 head = (++head) % capacity; 50 length--; 51 } 52 return node; 53 } 54 55 public void Traverse() { 56 for (int i = head; i < length + head; i++) { 57 Console.WriteLine(queue[i % capacity]); 58 Console.WriteLine($"前面还有{i - head}个"); 59 } 60 } 61 62 public void Dispose() { 63 queue = null; 64 } 65 }
为了能够通用,所以用的是泛型来实现环形队列类。这里最重要的是进队(EnQueue)和出队(DeQueue)两个方法,进队或出队后头和尾的位置都要通过取模运算来获得,因为是环形队列嘛,你懂的。
一、简单类型队列
好了,测试下入队:
1 class Program 2 { 3 static void Main(string[] args) { 4 MyQueue<int> queue = new MyQueue<int>(4); 5 queue.EnQueue(10); 6 queue.EnQueue(16); 7 queue.EnQueue(18); 8 queue.EnQueue(12); 9 queue.Traverse(); 10 Console.Read(); 11 } 12 }
显示结果:
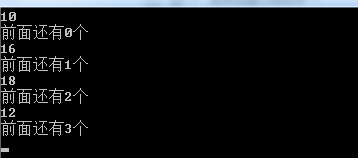
再测试下出队:
1 class Program 2 { 3 static void Main(string[] args) { 4 MyQueue<int> queue = new MyQueue<int>(4); 5 queue.EnQueue(10); 6 queue.EnQueue(16); 7 queue.EnQueue(18); 8 queue.EnQueue(12); 9 queue.Traverse(); 10 11 Console.WriteLine("弹两个出去"); 12 queue.DeQueue(); 13 queue.DeQueue(); 14 Console.WriteLine(); 15 queue.Traverse(); 16 Console.Read(); 17 } 18 }
运行结果:
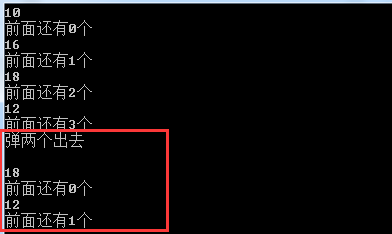
二、复杂类型队列
之前也说了,这个队列类是用的泛型写的,对应于C++的模板了,那就意味着任何类型都可以使用这个队列类,来测试个自定义的类试试,如下先定义一个Customer类:
1 public class Customer 2 { 3 public string Name { get; set; } 4 5 public int Age { get; set; } 6 7 public void PringInfo() { 8 Console.WriteLine("姓名:" + Name); 9 Console.WriteLine("年龄:" + Age); 10 Console.WriteLine(); 11 } 12 }
然后进行入队,如下:
1 class Program 2 { 3 static void Main(string[] args) { 4 MyQueue<Customer> queue = new MyQueue<Customer>(5); 5 queue.EnQueue(new Customer() { Name = "宋小二", Age = 29 }); 6 queue.EnQueue(new Customer() { Name = "陈小三", Age = 28 }); 7 queue.EnQueue(new Customer() { Name = "王小四", Age = 26 }); 8 queue.EnQueue(new Customer() { Name = "朱小五", Age = 48 }); 9 for (int i = 0; i < queue.Length(); i++) { 10 queue[i].PringInfo(); 11 } 12 Console.Read(); 13 } 14 }
上面的代码 queue[i].PringInfo();是通过索引来实现,所以我们得在队列类中实现索引,添加如下代码到MyQueue.cs类中,如下:
1 public T this[int index] { 2 get { 3 return queue[index]; 4 } 5 }
感觉用for循环来遍历还是不够好,想用foreach,那就给MyQueue类加个遍历接口,如下:
然后实现这个接口,如下:
1 public IEnumerator<T> GetEnumerator() { 2 foreach(T node in queue) { 3 if(node != null) { 4 yield return node; 5 } 6 } 7 } 8 9 IEnumerator IEnumerable.GetEnumerator() { 10 return GetEnumerator(); 11 }
这样遍历的地方就可以改成foreach了,如下:
执行结果:
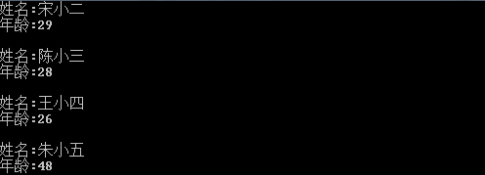
总结:
编程的思想才是最重要的,无关语言。
因为自己的水平有限,所以写的代码都很简单,如果哪写的不好或需要改进的,希望路过的高手不吝指教,如果能推荐就更完美了,谢谢阅读。