golang学习笔记7 使用beego swagger 实现API自动化文档
API 自动化文档 - beego: 简约 & 强大并存的 Go 应用框架
https://beego.me/docs/advantage/docs.md
使用beego开发api server 和前端拆分开发,使用swagger自动化生成API文档
Swagger 是一个规范和完整的框架,用于生成、描述、调用和可视化 RESTful 风格的 Web 服务。总体目标是使客户端和文件系统作为服务器以同样的速度来更新。
项目地址是:http://swagger.io/
使用 beego 开发自带集成了 swagger的东西。
https://beego.me/docs/advantage/docs.md
github 地址:
https://github.com/beego/swagger
到项目目录下执行命令:
bee run -gendoc=true -downdoc=true
会自动下载最新的 swagger 压缩文件
启动成功,直接访问
http://localhost:8080/swagger/
会出来两个url,http://localhost:8080/swagger/swagger.json
http://localhost:8080/swagger/swagger.yml
- 第一开启应用内文档开关,在配置文件中设置:
EnableDocs = true
, - 然后在你的
main.go
函数中引入_ "beeapi/docs"
(beego 1.7.0 之后版本不需要添加该引用)。 - 这样你就已经内置了 docs 在你的 API 应用中,然后你就使用
bee run -gendoc=true -downdoc=true
,让我们的 API 应用跑起来, -gendoc=true
表示每次自动化的 build 文档,-downdoc=true
就会自动的下载 swagger 文档查看器
到 https://github.com/swagger-api/swagger-ui 下载zip包,
解压后把dist文件夹复制到swagger目录下即可访问里面的index.html
为了方便把文件夹改名成show,把index.html 的url改成本地的
//url: "http://petstore.swagger.io/v2/swagger.json",
url:"http://localhost:8080/swagger/swagger.json",
在浏览器访问
http://localhost:8080/swagger/show/index.html
即可看到自己项目的api文档了,示例如下:
注:如果直接在文件夹打开dist下的index会报跨域的错误
注:bee pack时swagger 的他会自动解压文件到swagger目录下,不需要自己再放个目录了,可以把之前的show目录删掉了
添加auth token的方法,需要改造swagger的index.html
网上很多方法都是行不通的,在下面这个才找到可行的方法
Add JWT authorization header in Swagger v3 · Issue #2915 · swagger-api/swagger-ui · GitHub
https://github.com/swagger-api/swagger-ui/issues/2915
head 里面添加meta(这个swagger实际可以不要,其他测试可用):
<meta name="Authorization" id="head_auth" content="" >
body里面的部分内容改成下面代码,主要是添加一个configs配置,直接设置在head里面是没用的,需要设置在SwaggerUIBundle对象里面才有用,最终execute过去的请求是curl:
<script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.min.js"></script> <script> window.onload = function() { // Build a system const ui = SwaggerUIBundle({ url: "swagger.json", dom_id: '#swagger-ui', configs: { preFetch: function(req) { if (authToken) { req.headers["Authorization"] = authToken; } return req; } }, presets: [ SwaggerUIBundle.presets.apis, SwaggerUIStandalonePreset ], plugins: [ SwaggerUIBundle.plugins.DownloadUrl ], layout: "StandaloneLayout" }) window.ui = ui } var authToken = ""; function addApiKeyAuthorization(){ var key = encodeURIComponent(document.getElementById('input_apiKey').value); authToken = "Bearer " + key; //设置请求报文头 //var c1 = $("#head_auth").attr('content'); //console.log("c1 " + c1); $("#head_auth").attr('content',authToken); } </script>
没有设置的时候 验证不通过返回400错误信息
跨域的问题,可以在入口main方法里面加个过滤器filter,允许所有请求都跨域
beego/cors.go at master · astaxie/beego · GitHub
https://github.com/astaxie/beego/blob/master/plugins/cors/cors.go#L23
// Package cors provides handlers to enable CORS support. // Usage // import ( // "github.com/astaxie/beego" // "github.com/astaxie/beego/plugins/cors" // ) // // func main() { // // CORS for https://foo.* origins, allowing: // // - PUT and PATCH methods // // - Origin header // // - Credentials share // beego.InsertFilter("*", beego.BeforeRouter, cors.Allow(&cors.Options{ // AllowOrigins: []string{"https://*.foo.com"}, // AllowMethods: []string{"PUT", "PATCH"}, // AllowHeaders: []string{"Origin"}, // ExposeHeaders: []string{"Content-Length"}, // AllowCredentials: true, // })) // beego.Run() // }
或者单个 API 增加 CORS 支持
c.Ctx.Output.Header("Access-Control-Allow-Origin", "*")
另外https的无法访问http的,这个是协议限制的
要方便测试可以把所有都设置成通配符*
beego.InsertFilter("*", beego.BeforeRouter, cors.Allow(&cors.Options{ AllowOrigins: []string{"*"}, AllowMethods: []string{"*"}, AllowHeaders: []string{"*"}, ExposeHeaders: []string{"*"}, AllowCredentials: true, }))
---------------------------
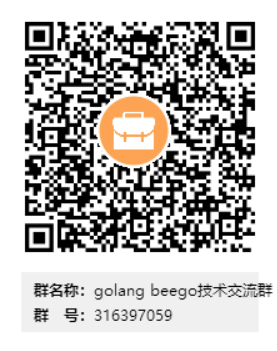