Vuex 的结构图
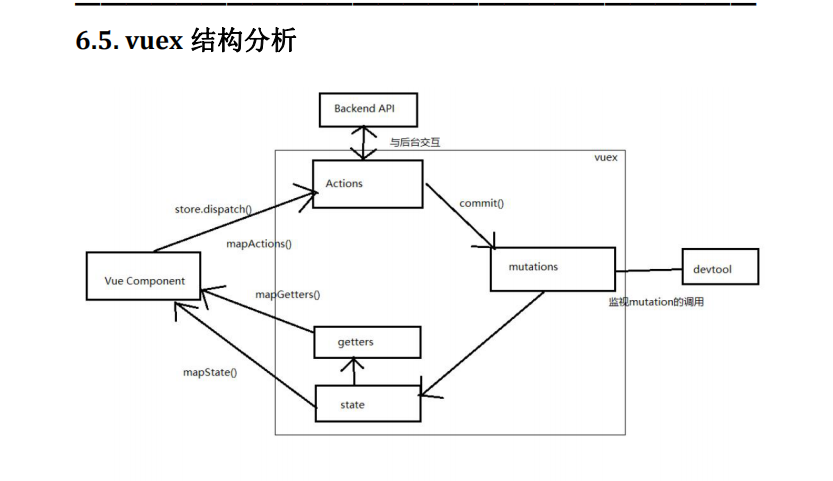
工程组织
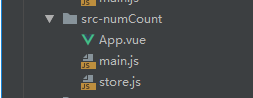
Vuex的核心管理程序 store.js
/*
vuex的核心管理程序
*/
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
//1. 状态
const state = { // 初始化状态
count: 0
}
//2. 包含多个更新state函数的对象
const mutations = {
//+1,-1 两个mutation
INCREMENT (state) {
state.count++
},
DECREMENT (state) {
state.count--
}
}
//3. 包含多个对应事件回调函数的对象
const actions = {
// 1) 增加的action
increment ({commit} ) {
// 提交一个mutation
commit('INCREMENT')
},
// 2) 减少的action
decrement ({commit}) {
commit('DECREMENT')
},
// 3) 带条件的action
incrementIfOdd ({commit,state}) {
if(state.count%2==1) {
//提交增加的mutation
commit('INCREMENT')
}
},
// 4) 异步的action
incrementAsync ({commit}) {
// 在action中直接可以执行异步的代码
setTimeout(()=>{
//500s后提交增加的mutation
commit('INCREMENT')
},500)
}
}
//4. 包含多个getter 计算属性函数的对象
const getters = {
evenOrOdd (state) { //state默认就是传入的,不需要手动加载
return state.count%2==0 ? '偶数':'奇数'
}
}
export default new Vuex.Store({
state, // 状态
mutations, // 包含多个更新state函数的对象
actions, // 包含多个对应事件回调函数的对象
getters, // 包含多个getter 计算属性函数的对象
})
main.js 进行全局注册,比如store组件,所有的组件对象都多了一个属性: $store
/*
入口JS
*/
import Vue from 'vue'
import App from './App.vue'
import store from './store'
// import '../static/base.css'
// 创建vm ,进行全局注册!!
new Vue({
el: '#app',
components: {App}, // 映射组件标签
template: '<App/>', // 指定需要渲染到页面的模板
store // 所有的组件对象都多了一个属性: $store
})
App.vue
<template>
<div style="text-align:center">
<p > click {{count}} times, count is {{evenOrOdd}} </p>
<!--老版写法: <p > click {{ $store.state.count}} times, count is {{evenOrOdd}} </p>-->
<button @click="increment">+</button>
<button @click="decrement">-</button>
<button @click="incrementIfOdd">increment If Odd</button>
<button @click="incrementAsync">increment Async</button>
</div>
</template>
<script>
import {mapState,mapGetters,mapActions} from 'vuex'
export default {
computed: {
...mapState(['count']),
...mapGetters(['evenOrOdd']),// mapGetters 返回值类型是对象: ,
//如下为麻烦写法/老版写法::::
// evenOrOdd () { // 在这里返回一个函数的对象,而不是返回函数的值,不用加()
// return this.$store.getters.evenOrOdd
// }
// count () {
// return this.$store.state.count
// }
},
methods: {
...mapActions(['increment','decrement','incrementIfOdd','incrementAsync'])
//如下为麻烦写法/老版写法::::
// 增加
// increment () {
// //通知Vuex去增加
// this.$store.dispatch('increment') // 触发store中对应的action
// },
// decrement () {
// this.$store.dispatch('decrement')
// },
// incrementIfOdd () {
// this.$store.dispatch('incrementIfOdd')
// },
// incrementAsync () {
// this.$store.dispatch('incrementAsync')
// }
}
}
</script>
<style>
</style>
浏览器效果展示
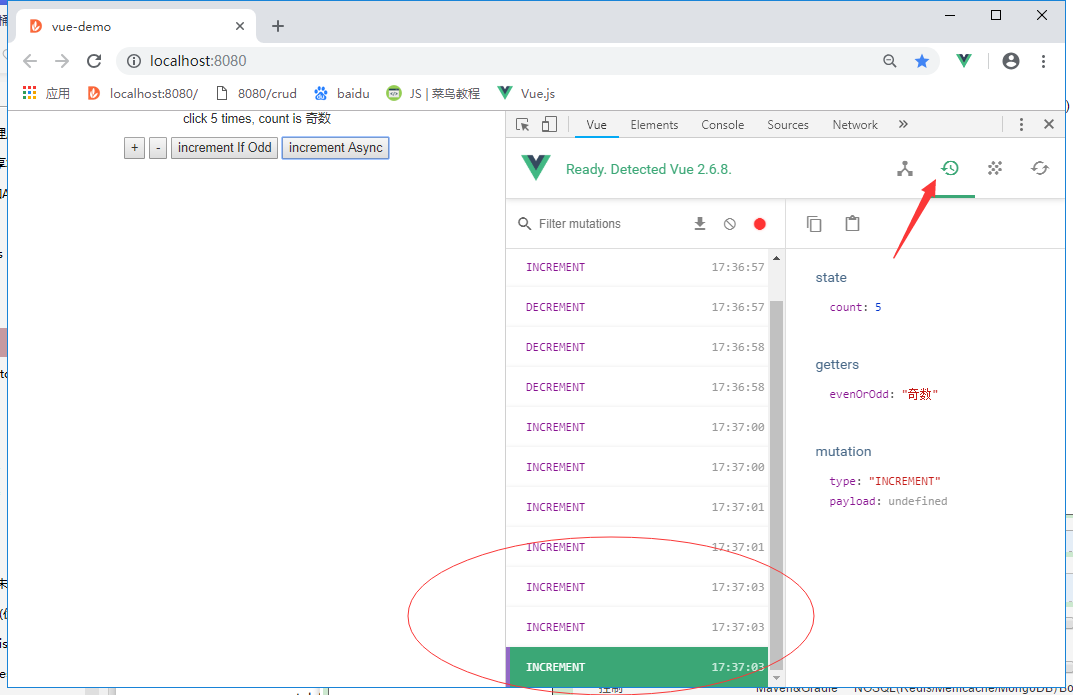