中介者模式
一、概述
中介者模式(Mediator Pattern)是用来降低多个对象和类之间的通信复杂性。这种模式提供了一个中介类,该类通常处理不同类之间的通信,并支持松耦合,使代码易于维护。中介者模式属于行为型模式。
二、介绍
用一个中介对象来封装一系列的对象交互,中介者使各对象不需要显式地相互引用,从而使其耦合松散,而且可以独立地改变它们之间的交互。
中介者模式将多个类相互耦合,形成的网状结构,分离为星型结构。
关键代码:对象 Colleague 之间的通信封装到一个类中单独处理。
它主要包含如下几个角色:
- Mediator: 抽象中介者。定义了同事对象到中介者对象之间的接口。
- ConcreteMediator: 具体中介者。实现抽象中介者的方法,它需要知道所有的具体同事类,同时需要从具体的同事类那里接收信息,并且向具体的同事类发送信息。
- Colleague: 抽象同事类。
- ConcreteColleague: 具体同事类。每个具体同事类都只需要知道自己的行为即可,但是他们都需要认识中介者。
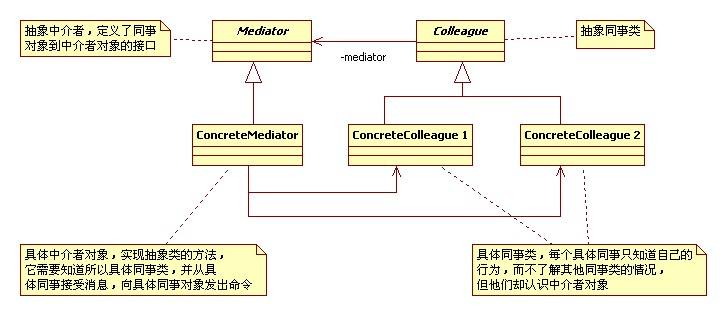
优点:
- 降低了类的复杂度,将一对多转化成了一对一。
- 各个类之间的解耦。
- 符合迪米特原则(一个对象应当对其他对象有尽可能少的了解)。
缺点:中介者会庞大,变得复杂难以维护。
使用场景:
- 系统中对象之间存在比较复杂的引用关系,导致它们之间的依赖关系结构混乱而且难以复用该对象。
- 想通过一个中间类来封装多个类中的行为,而又不想生成太多的子类。
三、代码实现
抽象中介类
package com.designpattern.mediatorPattern;
/**
* 抽象中介类
*
* @author zhongtao on 2018/12/19
*/
public abstract class Mediator {
public abstract void contact(String content, Colleague colleague);
}
抽象同事类
package com.designpattern.mediatorPattern;
/**
* 抽象同事类
*
* @author zhongtao on 2018/12/19
*/
public class Colleague {
protected String name;
protected Mediator mediator;
public Colleague(String name, Mediator mediator) {
this.name = name;
this.mediator = mediator;
}
}
具体同事类A和B
package com.designpattern.mediatorPattern;
/**
* 具体同事类A
*
* @author zhongtao on 2018/12/19
*/
public class ColleagueA extends Colleague {
public ColleagueA(String name, Mediator mediator) {
super(name, mediator);
}
public void getMessage(String message) {
System.out.println("同事A:" + name + " 获取信息:" + message);
}
public void contact(String message) {
mediator.contact(message, this);
}
}
/**
* 具体同事类B
*
* @author zhongtao on 2018/12/19
*/
public class ColleagueB extends Colleague {
public ColleagueB(String name, Mediator mediator) {
super(name, mediator);
}
public void getMessage(String message) {
System.out.println("同事B:" + name + " 获取信息:" + message);
}
public void contact(String message) {
mediator.contact(message, this);
}
}
具体中介类
package com.designpattern.mediatorPattern;
/**
* 具体中介类
*
* @author zhongtao on 2018/12/19
*/
public class ConcreteMediator extends Mediator {
private ColleagueA colleagueA;
private ColleagueB colleagueB;
public ColleagueA getColleagueA() {
return colleagueA;
}
public void setColleagueA(ColleagueA colleagueA) {
this.colleagueA = colleagueA;
}
public ColleagueB getColleagueB() {
return colleagueB;
}
public void setColleagueB(ColleagueB colleagueB) {
this.colleagueB = colleagueB;
}
@Override
public void contact(String content, Colleague colleague) {
if (colleague == colleagueA) {
colleagueB.getMessage(content);
} else {
colleagueA.getMessage(content);
}
}
}
客户端 测试中介者模式
package com.designpattern.mediatorPattern;
/**
* 客户端 测试中介者模式
*
* @author zhongtao on 2018/12/19
*/
public class Client {
public static void main(String[] args) {
ConcreteMediator mediator = new ConcreteMediator();
ColleagueA peter = new ColleagueA("Peter", mediator);
ColleagueB lina = new ColleagueB("Lina", mediator);
mediator.setColleagueA(peter);
mediator.setColleagueB(lina);
peter.contact("我是peter,想请lina晚上看电影");
lina.contact("我是lina,可以滴");
}
}
测试结果
同事B:Lina 获取信息:我是peter,想请lina晚上看电影
同事A:Peter 获取信息:我是lina,可以滴