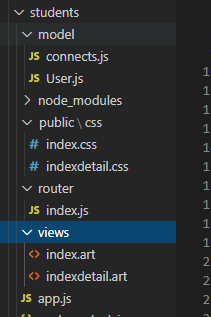
app01
// 引入http模块
const http = require('http');
//连接数据库
require('./model/connects');
// 创建网站服务器
const app = http.createServer();
//引入模板引擎
const template = require('art-template');
// 引入path模块
const path = require('path');
// 引入处理日期的第三方模块
const dateformat = require('dateformat')
// 引入静态资源访问模块
const serveStatic = require('serve-static');
// 引入querystring模块
const querystring = require('querystring');
const router = require('./router/index')
//实现静态资源访问服务
const serve = serveStatic(path.join(__dirname, 'public'))
// 配置模板的根目录
template.defaults.root = path.join(__dirname, 'views')
//处理日期格式的方法
template.defaults.imports.dateformat = dateformat
//当客户端访问服务器端的时候
app.on('request', (req, res) => {
// 启用路由功能
router(req, res, () => {})
// 启用静态资源访问服务功能
serve(req, res, () => {})
})
app.listen(3000);
console.log('服务器启动成功')
index.js
//引入router模块
const getRouter = require('router');
//引入模板引擎
const template = require('art-template');
// 引入querystring模块
const querystring = require('querystring');
//创建集合规则
const Student = require('../model/User')
// 获取路由对象
const router = getRouter();
//呈递学生档案信息页面
router.get('/add', (req, res) => {
let html = template('index.art', {});
res.end(html)
})
router.get('/list', async(req, res) => {
//查询学生信息
let student = await Student.find();
console.log(student)
let html = template('indexdetail.art', {
student: student
});
res.end(html)
})
//实现学生信息添加功能
router.post('/add', (req, res) => {
//接受post请求参数
let formData = '';
req.on('data', param => {
formData += param;
});
req.on('end', async() => {
await Student.create(querystring.parse(formData))
res.writeHead(301, {
Location: '/list'
});
res.end()
})
})
module.exports = router
connects.js
const mongoose = require('mongoose')
// 连接数据库
mongoose.connect('mongodb://localhost/playground', { useNewUrlParser: true })
.then(() => console.log('数据库连接成功'))
.catch(() => console.log('数据库连失败'))
Users.js
const mongoose = require('mongoose')
// 创建学生集合规则
const studentsSchema = new mongoose.Schema({
name: {
type: String,
require: true,
minlength: 2,
maxlength: 10
},
age: {
type: Number,
min: 10,
max: 25
},
sex: {
type: String
},
email: String,
hobbies: [String],
collage: String,
enterDate: {
type: Date,
default: Date.now
}
})
// 创建集合规则
const Student = mongoose.model('Student', studentsSchema)
module.exports = Student