这是在看侯捷C++视频的时候,他提出的一个问题,称之为单例模式(singleton),有两种实现方式(懒汉与饿汉),还有多线程,使用场景(工厂模式)等等一些相关的东西,更多了解可以去百度一下。在此就简单做一个总结。
方法一共有n个。根据侯老师所言,目前这两种是比较好的实现方式,所以找出来与大家分享一下。一是:
#include<iostream>
#include<vector>
#include<string>
using namespace std;
class A{
public:
static A& getObject() {
return a ;
}
void set(int t){
temp = t ;
}
void print(){
cout << "temp == "<< temp << endl ;
}
private:
A() = default ;
A(const A& rhs);
static A a ;
int temp ;
};
A A::a ;
int main(void){
A::getObject().set(666);
A::getObject().print();
return 0 ;
}
运行结果:
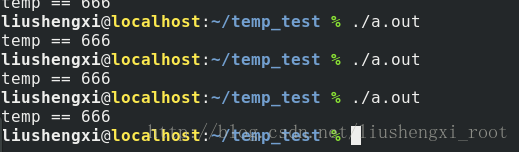
另外还有下面的这种设计模式。相较于上面的,它就是只要用到就实例化出来,不用到就不浪费内存空间。
#include<iostream>
#include<vector>
#include<string>
using namespace std;
class A{
public:
static A& getObject() {
static A a ;
return a ;
}
void set(int t){
temp = t ;
}
void print(){
cout << "temp == "<< temp << endl ;
}
private:
A() = default ;
A(const A& rhs) ;
int temp ;
};
int main(void){
A::getObject().set(45);
A::getObject().print();
return 0 ;
}
运行结果:

当然了,使用new实现也是完全可以的。但是我觉得不是很建议。
#include<iostream>
#include<vector>
#include<string>
using namespace std;
class A{
public:
static A* getObject();
void set(int t){
temp = t ;
}
void print(){
cout << "temp == "<< temp << endl ;
}
private:
A() = default ;
A(const A& rhs) ;
~A(){
delete p_A ;
}
A& operator=(const A&);
static A *p_A ;
int temp ;
};
A* A::p_A = new A();
A* A::getObject(){
return p_A;
}
int main(void){
A* A1 = A::getObject();
A* A2 = A::getObject();
if (A1 == A2)
fprintf(stderr,"A1 = A2
");
A1->set(888);
A1->print();
return 0;
}
运行结果:
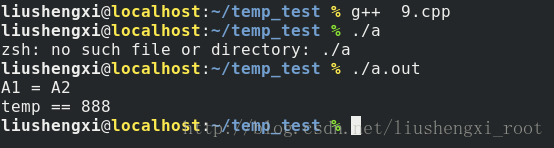