一、基本概念
1、Object划分
(1)PO(persistant object)持久对象
PO 就是对应数据库中某个表中的一条记录,多个记录可以用 PO 的集合。 PO中应该不包含任何对数据库的操作。
(2)Do(Domain Object)领域对象
就是从现实世界中抽象出来的有形或无形的业务实体。
(3)To(Transfer Object)数据传输对象
不同的应用程序之间传输的对象
(4)DTO(Data Transfer Object)数据传输对象
这个概念来源于 J2EE 的设计模式,原来的目的是为了 EJB 的分布式应用提供粗粒度的数据实体,以减少分布式调用的次数,从而提高分布式调用的性能和降低网络负载,但在这里,泛指用于展示层与服务层之间的数据传输对象。
(5)VO(value object)值对象
通常用于业务层之间的数据传递,和 PO 一样也是仅仅包含数据而已。但应是抽象出的业务对象 , 可以和表对应 , 也可以不 , 这根据业务的需要 。用 new 关键字创建,由GC 回收的。
View object:视图对象;
接受页面传递来的数据,封装对象
将业务处理完成的对象,封装成页面要用的数据
(6)BO(business object)业务对象
从业务模型的角度看 , 见 UML 元件领域模型中的领域对象。封装业务逻辑的 java 对象 , 通过调用 DAO 方法 , 结合 PO,VO 进行业务操作。business object: 业务对象 主要作用是把业务逻辑封装为一个对象。这个对象可以包括一个或多个其它的对象。 比如一个简历,有教育经历、工作经历、社会关系等等。 我们可以把教育经历对应一个 PO ,工作经历对应一个 PO ,社会关系对应一个 PO 。 建立一个对应简历的 BO 对象处理简历,每个 BO 包含这些 PO 。 这样处理业务逻辑时,我们就可以针对 BO 去处理。
(7)POJO(plain ordinary java object) 简单无规则 java 对象
传统意义的 java 对象。就是说在一些 Object/Relation Mapping 工具中,能够做到维护数据库表记录的 persisent object 完全是一个符合 Java Bean 规范的纯 Java 对象,没有增加别的属性和方法。我的理解就是最基本的 java Bean ,只有属性字段及 setter 和 getter方法!。
POJO 是 DO/DTO/BO/VO 的统称。
(8)DAO(data access object) 数据访问对象
是一个 sun 的一个标准 j2ee 设计模式, 这个模式中有个接口就是 DAO ,它负持久层的操作。为业务层提供接口。此对象用于访问数据库。通常和 PO 结合使用, DAO 中包含了各种数据库的操作方法。通过它的方法 , 结合 PO 对数据库进行相关的操作。夹在业务逻辑与数据库资源中间。配合 VO, 提供数据库的 CRUD 操作.
二、平台属性
2、规格参数新增与VO
(1)处理“平台属性/属性分组”的模糊搜索无效问题
com.atguigu.gulimall.product.service.impl.AttrGroupServiceImpl 修改queryPage方法
/**
* 分类查询分类属性分组
* @param params
* @param catelogId
* @return
*/
@Override
public PageUtils queryPage(Map<String, Object> params, Long catelogId) {
String key = (String) params.get("key");
//select * from pms_attr_group where catelog_id=? and (attr_group_id=key or attr_group_name like %key%)
QueryWrapper<AttrGroupEntity> wrapper = new QueryWrapper<AttrGroupEntity>();
if(!StringUtils.isEmpty(key)){
wrapper.and((obj)->{
obj.eq("attr_group_id", key).or().like("attr_group_name",key);
});
}
if(catelogId == 0){
IPage<AttrGroupEntity> page = this.page(new Query<AttrGroupEntity>().getPage(params), wrapper);
return new PageUtils(page);
}else{
wrapper.eq("catelog_id",catelogId);
IPage<AttrGroupEntity> page = this.page(new Query<AttrGroupEntity>().getPage(params), wrapper);
return new PageUtils(page);
}
}
效果:

(2)新增“规格参数“
(a)新建文件 com.atguigu.gulimall.product.vo.AttrVo
package com.atguigu.gulimall.product.vo;
import com.baomidou.mybatisplus.annotation.TableId;
import lombok.Data;
@Data
public class AttrVo {
/**
* 属性id
*/
private Long attrId;
/**
* 属性名
*/
private String attrName;
/**
* 是否需要检索[0-不需要,1-需要]
*/
private Integer searchType;
/**
* 值类型[0-为单个值,1-可以选择多个值]
*/
private Integer valueType;
/**
* 属性图标
*/
private String icon;
/**
* 可选值列表[用逗号分隔]
*/
private String valueSelect;
/**
* 属性类型[0-销售属性,1-基本属性,2-既是销售属性又是基本属性]
*/
private Integer attrType;
/**
* 启用状态[0 - 禁用,1 - 启用]
*/
private Long enable;
/**
* 所属分类
*/
private Long catelogId;
/**
* 快速展示【是否展示在介绍上;0-否 1-是】,在sku中仍然可以调整
*/
private Integer showDesc;
//属性分组id
private Long attrGroupId;
}
(b)修改 com.atguigu.gulimall.product.controller.AttrController 的save方法
/**
* 保存
*/
@RequestMapping("/save")
//@RequiresPermissions("product:attr:save")
public R save(@RequestBody AttrVo attr){
attrService.saveAttr(attr);
return R.ok();
}
(c)新增接口和实现类方法saveAttr
/**
* 保存属性
* @param attr
*/
@Override
@Transactional
public void saveAttr(AttrVo attr) {
//(1)保存基本数据
AttrEntity attrEntity = new AttrEntity();
//attrEntity.setAttrName(attr.getAttrName());
BeanUtils.copyProperties(attr, attrEntity);
this.save(attrEntity);
//(2)保存关联关系
AttrAttrgroupRelationEntity relationEntity = new AttrAttrgroupRelationEntity();
relationEntity.setAttrGroupId(attr.getAttrGroupId());
relationEntity.setAttrId(attrEntity.getAttrId());
relationDao.insert(relationEntity);
}
3、规格参数列表
(1)新建文件 com.atguigu.gulimall.product.vo.AttrRespVo
package com.atguigu.gulimall.product.vo;
import lombok.Data;
/**
* @Description:
* @Created: with IntelliJ IDEA.
* @author: 夏沫止水
* @createTime: 2020-05-29 09:26
**/
@Data
public class AttrRespVo extends AttrVo {
/**
*分类名称
*/
private String catelogName;
/**
* 分组名称
*/
private String groupName;
}
(2)com.atguigu.gulimall.product.controller.AttrController 新增方法baseAttrList
/**
* 获取分类规格属性
* @param params
* @param catelogId
* @return
*/
///product/attr/base/list/{catelogId}
@GetMapping("/base/list/{catelogId}")
public R baseAttrList(@RequestParam Map<String, Object> params,
@PathVariable("catelogId")Long catelogId){
PageUtils data = attrService.queryBaseAttrPage(params, catelogId);
return R.ok().put("page", data);
}
(3)新增接口和实现类方法queryBaseAttrPage
/**
* 获取分类销售属性
* @param params
* @param catelogId
* @return
*/
@Override
public PageUtils queryBaseAttrPage(Map<String, Object> params, Long catelogId) {
QueryWrapper<AttrEntity> queryWrapper = new QueryWrapper<>();
if(catelogId != 0){
queryWrapper.eq("catelog_id", catelogId);
}
String key = (String) params.get("key");
if(!StringUtils.isEmpty(key)){
queryWrapper.and((wrapper)->{
wrapper.eq("attr_id",key).or().like("attr_name",key);
});
}
IPage<AttrEntity> page = this.page(
new Query<AttrEntity>().getPage(params),
queryWrapper
);
PageUtils pageUtils = new PageUtils(page);
List<AttrEntity> records = page.getRecords();
List<AttrRespVo> respVos = records.stream().map((attrEntity) -> {
AttrRespVo attrRespVo = new AttrRespVo();
BeanUtils.copyProperties(attrEntity, attrRespVo);
//设置分类和分组的名字
AttrAttrgroupRelationEntity attrId = relationDao.selectOne(new QueryWrapper<AttrAttrgroupRelationEntity>().eq("attr_id", attrEntity.getAttrId()));
if (attrId != null) {
AttrGroupEntity attrGroupEntity = attrGroupDao.selectById(attrId.getAttrGroupId());
attrRespVo.setGroupName(attrGroupEntity.getAttrGroupName());
}
CategoryEntity categoryEntity = categoryDao.selectById(attrEntity.getCatelogId());
if (categoryEntity != null) {
attrRespVo.setCatelogName(categoryEntity.getName());
}
return attrRespVo;
}).collect(Collectors.toList());
pageUtils.setList(respVos);
return pageUtils;
}
最终效果:
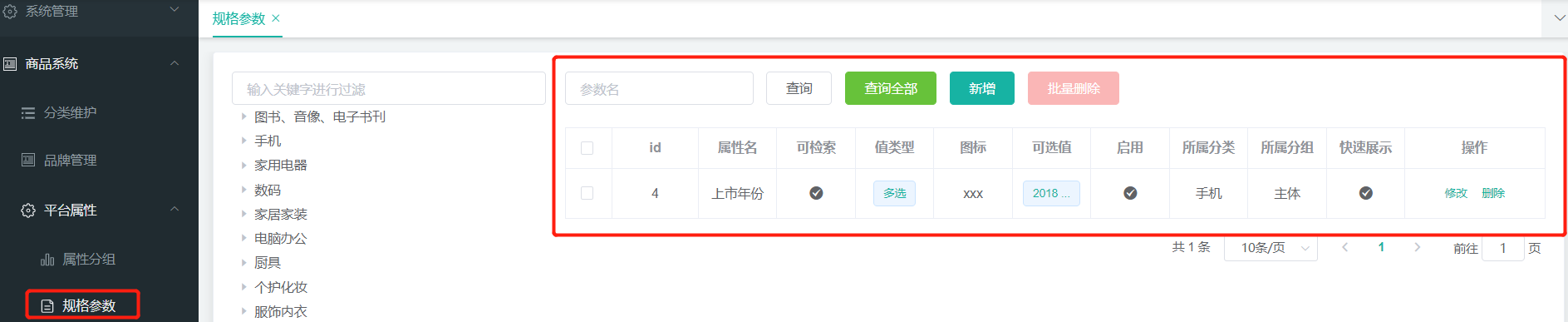
4、规格修改
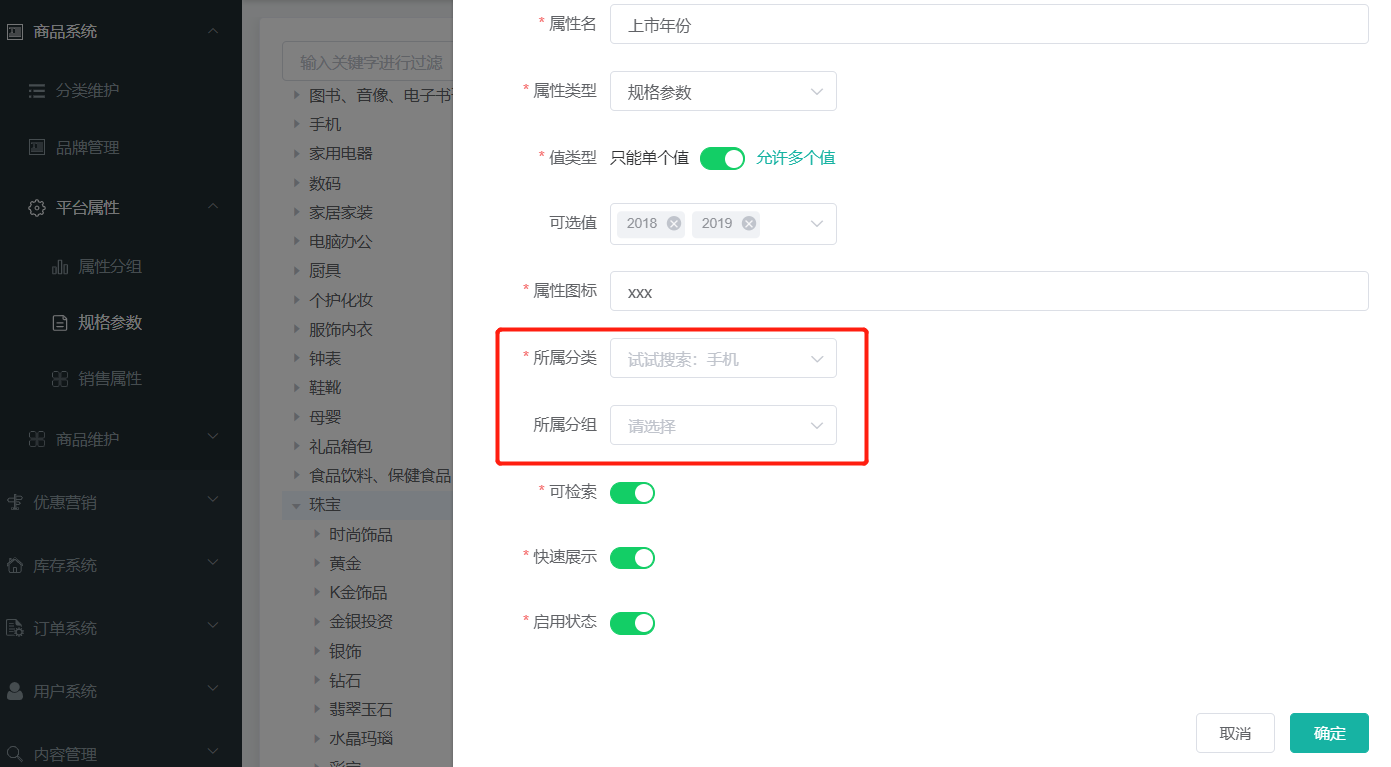
可以看到所属分类和所属分组没有回显出来
(1)回显“所属分类”和“所属分组”修改 (a)com.atguigu.gulimall.product.controller.AttrController 修改 info 方法 /** * 信息 */ @RequestMapping("/info/{attrId}") //@RequiresPermissions("product:attr:info") public R info(@PathVariable("attrId") Long attrId){ //AttrEntity attr = attrService.getById(attrId); AttrRespVo respVo = attrService.getAttrInfo(attrId); return R.ok().put("attr", respVo); } (b)新增接口和实现类方法 getAttrInfo /** * 查询属性详情 * @param attrId * @return */ @Override public AttrRespVo getAttrInfo(Long attrId) { AttrRespVo attrRespVo = new AttrRespVo(); AttrEntity attrEntity = this.getById(attrId); BeanUtils.copyProperties(attrEntity, attrRespVo); //1、设置分组信息 AttrAttrgroupRelationEntity attrgroupRelation = relationDao.selectOne(new QueryWrapper<AttrAttrgroupRelationEntity>().eq("attr_id", attrEntity.getAttrId())); if(attrgroupRelation!=null){ attrRespVo.setAttrGroupId(attrgroupRelation.getAttrGroupId()); AttrGroupEntity attrGroupEntity = attrGroupDao.selectById(attrgroupRelation.getAttrGroupId()); if(attrGroupEntity!=null){ attrRespVo.setGroupName(attrGroupEntity.getAttrGroupName()); } } //2、设置分类信息 Long catelogId = attrEntity.getCatelogId(); Long[] catelogPath = categoryService.findCatelogPath(catelogId); attrRespVo.setCatelogPath(catelogPath); CategoryEntity categoryEntity = categoryDao.selectById(catelogId); if(categoryEntity!=null){ attrRespVo.setCatelogName(categoryEntity.getName()); } return attrRespVo; } 最终效果
(2)修改“所属分类”和“所属分组”
(a)com.atguigu.gulimall.product.controller.AttrController 修改 update 方法
/**
* 修改
*/
@RequestMapping("/update")
//@RequiresPermissions("product:attr:update")
public R update(@RequestBody AttrVo attr){
//attrService.updateById(attr);
attrService.updateAttr(attr);
return R.ok();
}
(b)新增接口和实现类方法 updateAttr
/**
* 修改属性
* @param attr
*/
@Override
public void updateAttr(AttrVo attr) {
AttrEntity attrEntity = new AttrEntity();
BeanUtils.copyProperties(attr, attrEntity);
this.updateById(attrEntity);
//1、修改分组关联
AttrAttrgroupRelationEntity relationEntity = new AttrAttrgroupRelationEntity();
relationEntity.setAttrGroupId(attr.getAttrGroupId());
relationEntity.setAttrId(attr.getAttrId());
Integer selectCount = relationDao.selectCount(new QueryWrapper<AttrAttrgroupRelationEntity>().eq("attr_id", attr.getAttrId()));
if(selectCount>0){
relationDao.update(relationEntity, new UpdateWrapper<AttrAttrgroupRelationEntity>().eq("attr_id", attr.getAttrId()));
}else{
relationDao.insert(relationEntity);
}
}
5、销售属性维护
(1)gulimall-common 新建常量类 com.atguigu.common.constant.ProductConstant
package com.atguigu.common.constant;
public class ProductConstant {
public enum AttrEnum{
ATTR_TYPE_BASE(1,"基本属性"),ATTR_TYPE_SALE(0,"销售属性");
private int code;
private String msg;
AttrEnum(int code, String msg){
this.code = code;
this.msg = msg;
}
public int getCode() {
return code;
}
public String getMsg() {
return msg;
}
}
}
(2)修改 com.atguigu.gulimall.product.controller.AttrController 的 baseAttrList 方法
/**
* 获取分类规格参数
* @param params
* @param catelogId
* @return
*/
///product/attr/base/list/{catelogId}
@GetMapping("/{attrType}/list/{catelogId}")
public R baseAttrList(@RequestParam Map<String, Object> params,
@PathVariable("catelogId")Long catelogId,
@PathVariable("attrType")String type){
PageUtils data = attrService.queryBaseAttrPage(params, catelogId, type);
return R.ok().put("page", data);
}
(3)修改 com.atguigu.gulimall.product.service.impl.AttrServiceImpl 的 queryBaseAttrPage 方法
/**
* 获取分类销售属性
* @param params
* @param catelogId
* @param attrType
* @return
*/
@Override
public PageUtils queryBaseAttrPage(Map<String, Object> params, Long catelogId, String attrType) {
QueryWrapper<AttrEntity> queryWrapper = new QueryWrapper<AttrEntity>()
.eq("attr_type","base".equalsIgnoreCase(attrType) ?
ProductConstant.AttrEnum.ATTR_TYPE_BASE.getCode() : ProductConstant.AttrEnum.ATTR_TYPE_SALE.getCode());
//根据catelogId查询信息
if(catelogId != 0){
queryWrapper.eq("catelog_id", catelogId);
}
String key = (String) params.get("key");
if(!StringUtils.isEmpty(key)){
queryWrapper.and((wrapper)->{
wrapper.eq("attr_id",key).or().like("attr_name",key);
});
}
IPage<AttrEntity> page = this.page(
new Query<AttrEntity>().getPage(params),
queryWrapper
);
PageUtils pageUtils = new PageUtils(page);
List<AttrEntity> records = page.getRecords();
List<AttrRespVo> respVos = records.stream().map((attrEntity) -> {
AttrRespVo attrRespVo = new AttrRespVo();
BeanUtils.copyProperties(attrEntity, attrRespVo);
//设置分类和分组的名字
if("base".equalsIgnoreCase(attrType)){
AttrAttrgroupRelationEntity attrId = relationDao.selectOne(new QueryWrapper<AttrAttrgroupRelationEntity>().eq("attr_id", attrEntity.getAttrId()));
if (attrId != null && attrId.getAttrGroupId()!=null) {
AttrGroupEntity attrGroupEntity = attrGroupDao.selectById(attrId.getAttrGroupId());
attrRespVo.setGroupName(attrGroupEntity.getAttrGroupName());
}
}
CategoryEntity categoryEntity = categoryDao.selectById(attrEntity.getCatelogId());
if (categoryEntity != null) {
attrRespVo.setCatelogName(categoryEntity.getName());
}
return attrRespVo;
}).collect(Collectors.toList());
pageUtils.setList(respVos);
return pageUtils;
}
(4)修改 com.atguigu.gulimall.product.service.impl.AttrServiceImpl 的 saveAttr 方法
/**
* 保存属性
* @param attr
*/
@Override
@Transactional
public void saveAttr(AttrVo attr) {
//(1)保存基本数据
AttrEntity attrEntity = new AttrEntity();
//attrEntity.setAttrName(attr.getAttrName());
BeanUtils.copyProperties(attr, attrEntity);
this.save(attrEntity);
//(2)保存关联关系
if(attr.getAttrType() == ProductConstant.AttrEnum.ATTR_TYPE_BASE.getCode() && attr.getAttrGroupId!=null){
AttrAttrgroupRelationEntity relationEntity = new AttrAttrgroupRelationEntity();
relationEntity.setAttrGroupId(attr.getAttrGroupId());
relationEntity.setAttrId(attrEntity.getAttrId());
relationDao.insert(relationEntity);
}
}
(5)修改 com.atguigu.gulimall.product.service.impl.AttrServiceImpl 的 getAttrInfo 方法
/**
* 查询属性详情
* @param attrId
* @return
*/
@Override
public AttrRespVo getAttrInfo(Long attrId) {
AttrRespVo attrRespVo = new AttrRespVo();
AttrEntity attrEntity = this.getById(attrId);
BeanUtils.copyProperties(attrEntity, attrRespVo);
if(attrEntity.getAttrType() == ProductConstant.AttrEnum.ATTR_TYPE_BASE.getCode()){
//1、设置分组信息
AttrAttrgroupRelationEntity attrgroupRelation = relationDao.selectOne(new QueryWrapper<AttrAttrgroupRelationEntity>().eq("attr_id", attrEntity.getAttrId()));
if(attrgroupRelation!=null){
attrRespVo.setAttrGroupId(attrgroupRelation.getAttrGroupId());
AttrGroupEntity attrGroupEntity = attrGroupDao.selectById(attrgroupRelation.getAttrGroupId());
if(attrGroupEntity!=null){
attrRespVo.setGroupName(attrGroupEntity.getAttrGroupName());
}
}
}
//2、设置分类信息
Long catelogId = attrEntity.getCatelogId();
Long[] catelogPath = categoryService.findCatelogPath(catelogId);
attrRespVo.setCatelogPath(catelogPath);
CategoryEntity categoryEntity = categoryDao.selectById(catelogId);
if(categoryEntity!=null){
attrRespVo.setCatelogName(categoryEntity.getName());
}
return attrRespVo;
}
(6)修改 com.atguigu.gulimall.product.service.impl.AttrServiceImpl 的 updateAttr 方法
/**
* 修改属性
* @param attr
*/
@Override
public void updateAttr(AttrVo attr) {
AttrEntity attrEntity = new AttrEntity();
BeanUtils.copyProperties(attr, attrEntity);
this.updateById(attrEntity);
if(attrEntity.getAttrType() == ProductConstant.AttrEnum.ATTR_TYPE_BASE.getCode()){
//1、修改分组关联
AttrAttrgroupRelationEntity relationEntity = new AttrAttrgroupRelationEntity();
relationEntity.setAttrGroupId(attr.getAttrGroupId());
relationEntity.setAttrId(attr.getAttrId());
Integer selectCount = relationDao.selectCount(new QueryWrapper<AttrAttrgroupRelationEntity>().eq("attr_id", attr.getAttrId()));
if(selectCount>0){
relationDao.update(relationEntity, new UpdateWrapper<AttrAttrgroupRelationEntity>().eq("attr_id", attr.getAttrId()));
}else{
relationDao.insert(relationEntity);
}
}
}
注意:只有基本属性才有分组,销售属性不存在分组
效果:
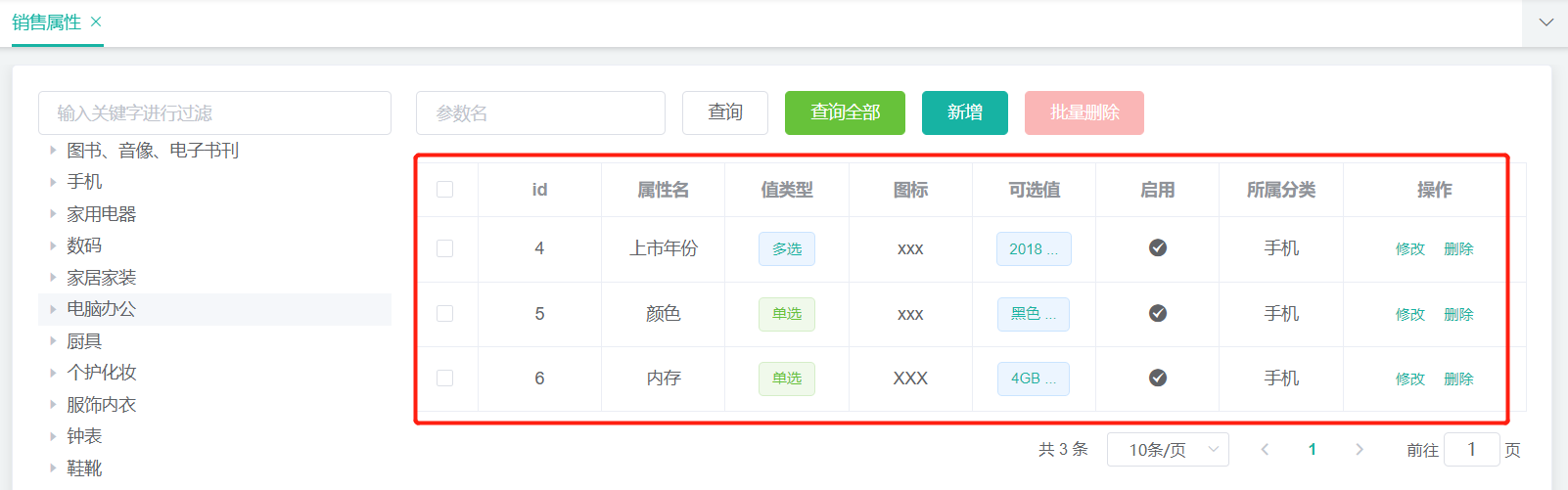
6、查询分组关联属性 & 删除关联
(1)查询分组关联属性
(a)com.atguigu.gulimall.product.controller.AttrGroupController 新加方法
/**
* 获取属性分组的关联的所有属性
* @param attrgroupId
* @return
*/
///product/attrgroup/{attrgroupId}/attr/relation
@GetMapping("/{attrgroupId}/attr/relation")
public R attrRelation(@PathVariable("attrgroupId")Long attrgroupId){
List<AttrEntity> entities = attrService.getRelationAttr(attrgroupId);
return R.ok().put("data", entities);
}
(b)新增接口和实现类方法 getRelationAttr
/**
* 获取属性分组的关联的所有属性
* @param attrgroupId
* @return
*/
@Override
public List<AttrEntity> getRelationAttr(Long attrgroupId) {
List<AttrAttrgroupRelationEntity> entities = relationDao.selectList(new QueryWrapper<AttrAttrgroupRelationEntity>().eq("attr_group_id", attrgroupId));
List<Long> attrIds = entities.stream().map((attr) -> {
return attr.getAttrId();
}).collect(Collectors.toList());
//如果attrIds为空就直接返回一个null值出去
if (attrIds == null || attrIds.size() == 0) {
return null;
}
//根据attrIds查找所有的属性信息
Collection<AttrEntity> attrEntities = this.listByIds(attrIds);
return (List<AttrEntity>) attrEntities;
}
效果:
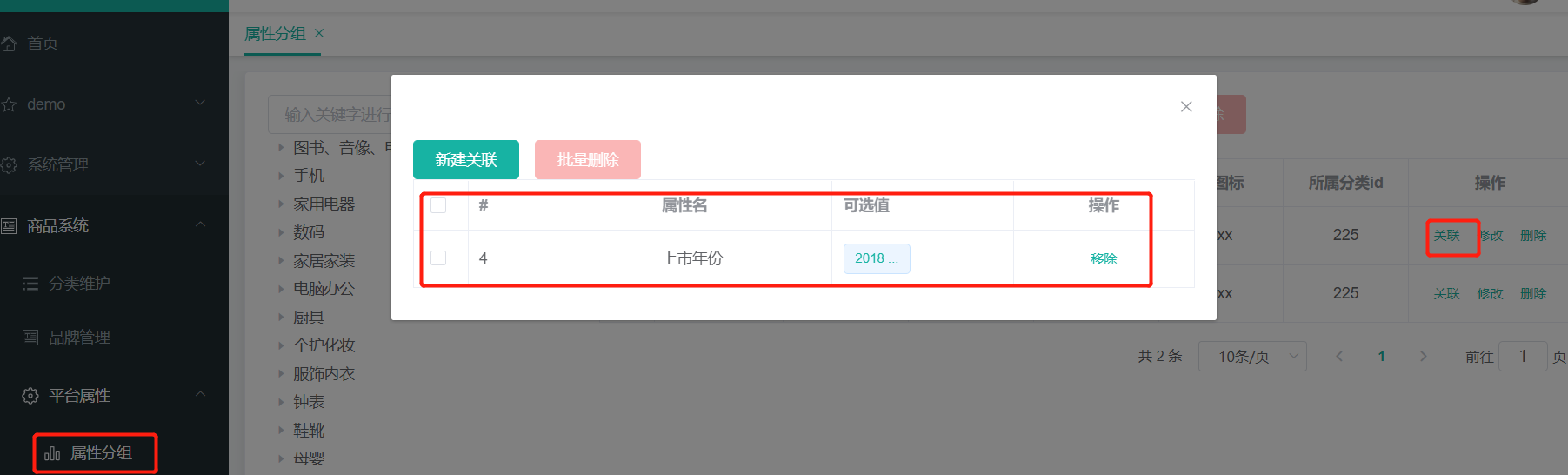
(2)删除关联
(a)新建vo类 com.atguigu.gulimall.product.vo.AttrGroupRelationVo
package com.atguigu.gulimall.product.vo;
import lombok.Data;
@Data
public class AttrGroupRelationVo {
//[{"attrId":1,"attrGroupId":2}]
private Long attrId;
private Long attrGroupId;
}
(b)com.atguigu.gulimall.product.controller.AttrGroupController 新加方法 deleteRelation
/**
* 删除属性与分组的关联关系
* @param vos
* @return
*/
///product/attrgroup/attr/relation/delete
@PostMapping("/attr/relation/delete")
public R deleteRelation(@RequestBody AttrGroupRelationVo[] vos){
attrService.deleteRelation(vos);
return R.ok();
}
(c)新增接口和实现类方法 deleteRelation
/**
* 删除属性与分组的关联关系
* @param vos
*/
@Override
public void deleteRelation(AttrGroupRelationVo[] vos) {
//批量删除
List<AttrAttrgroupRelationEntity> entities = Arrays.asList(vos).stream().map((item) -> {
AttrAttrgroupRelationEntity relationEntity = new AttrAttrgroupRelationEntity();
BeanUtils.copyProperties(item, relationEntity);
return relationEntity;
}).collect(Collectors.toList());
relationDao.deleBatchRelation(entities);
}
(d)com.atguigu.gulimall.product.dao.AttrAttrgroupRelationDao 新增接口
void deleBatchRelation(@Param("entities") List<AttrAttrgroupRelationEntity> entities);
(e)AttrAttrgroupRelationDao.xml 新增xml方法 deleBatchRelation
<delete id="deleBatchRelation">
DELETE FROM pms_attr_attrgroup_relation WHERE
<foreach collection="entities" item="item" separator=" OR ">
(attr_id=#{item.attrId} AND attr_group_id=#{item.attrGroupId})
</foreach>
</delete>
7、查询分组未关联的属性
(1)com.atguigu.gulimall.product.controller.AttrGroupController 新增方法 noattrRelation
/**
* 获取属性分组没有关联的其他属性
* @param attrgroupId
* @param params
* @return
*/
///product/attrgroup/{attrgroupId}/noattr/relation
@GetMapping("/{attrgroupId}/noattr/relation")
public R noattrRelation(@PathVariable("attrgroupId")Long attrgroupId, @RequestParam Map<String, Object> params){
PageUtils page = attrService.getNoRelationAttr(params, attrgroupId);
return R.ok().put("page", page);
}
(2)新增接口和实现类方法 getNoRelationAttr
/**
* 获取属性分组没有关联的其他属性
* @param params
* @param attrgroupId
* @return
*/
@Override
public PageUtils getNoRelationAttr(Map<String, Object> params, Long attrgroupId) {
//1、当前分组只能关联自己所属的分类里面的所有属性
AttrGroupEntity attrGroupEntity = attrGroupDao.selectById(attrgroupId);
Long catelogId = attrGroupEntity.getCatelogId();
//2、当前分组只能关联别的分组没有引用的属性
//(1)当前分类下的其他分组
List<AttrGroupEntity> group = attrGroupDao.selectList(new QueryWrapper<AttrGroupEntity>().eq("catelog_id", catelogId));
List<Long> collect = group.stream().map(item -> {
return item.getAttrGroupId();
}).collect(Collectors.toList());
//(2)这些分组关联的属性
List<AttrAttrgroupRelationEntity> groupId = relationDao.selectList(new QueryWrapper<AttrAttrgroupRelationEntity>().in("attr_group_id",collect));
List<Long> attrIds = groupId.stream().map(item -> {
return item.getAttrId();
}).collect(Collectors.toList());
//(3)从当前分类的所有属性中移除这些属性
QueryWrapper<AttrEntity> wrapper = new QueryWrapper<AttrEntity>().eq("catelog_id", catelogId);
if(attrIds!=null && attrIds.size()>0){
wrapper.notIn("attr_id", attrIds);
}
String key = (String) params.get("key");
if(!StringUtils.isEmpty(key)){
wrapper.and((w)->{
w.eq("attr_id",key).or().like("attr_name",key);
});
}
IPage<AttrEntity> page = this.page(new Query<AttrEntity>().getPage(params), wrapper);
PageUtils pageUtils = new PageUtils(page);
return pageUtils;
}
效果:
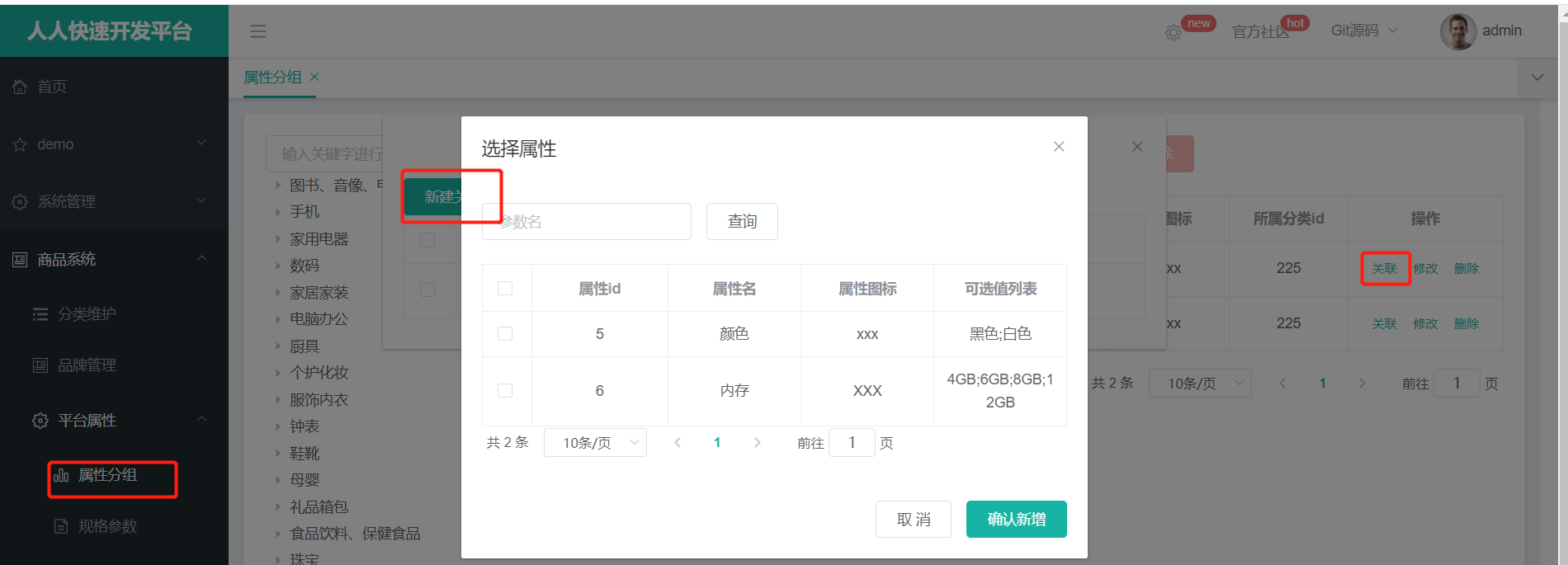
8、新增分组与属性关联
(1)com.atguigu.gulimall.product.controller.AttrGroupController 新增方法 addRelation
/**
* 添加属性与分组关联关系
* @param vos
* @return
*/
//product/attrgroup/attr/relation
@PostMapping("/attr/relation")
public R addRelation(@RequestBody List<AttrGroupRelationVo> vos){
attrAttrgroupRelationService.saveBatch(vos);
return R.ok();
}
(2)新增接口和实现类方法 saveBatch
/**
* 添加属性与分组关联关系
* @param vos
*/
@Override
public void saveBatch(List<AttrGroupRelationVo> vos) {
List<AttrAttrgroupRelationEntity> collect = vos.stream().map(item -> {
AttrAttrgroupRelationEntity relationEntity = new AttrAttrgroupRelationEntity();
BeanUtils.copyProperties(item, relationEntity);
return relationEntity;
}).collect(Collectors.toList());
this.saveBatch(collect);
}