Description
In this problem, you have to analyze a particular sorting algorithm. The algorithm processes a sequence of n distinct integers by swapping two adjacent sequence elements until the sequence is sorted in ascending
order. For the input sequence
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.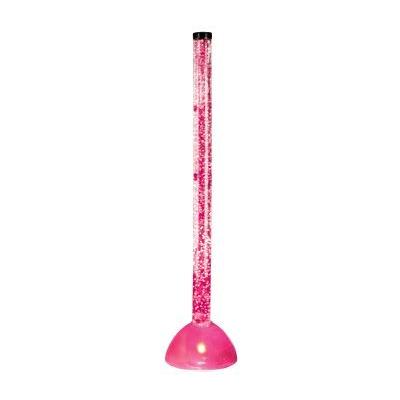
Ultra-QuickSort produces the output
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
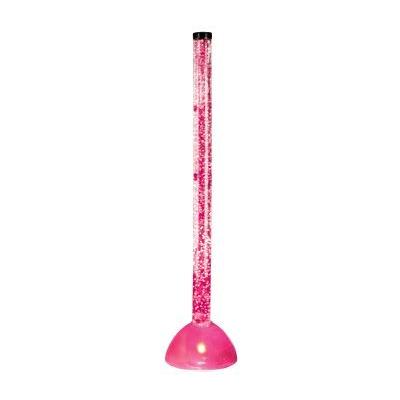
Input
The input contains several test cases. Every test case begins with a line that contains a single integer n < 500,000 -- the length of the input sequence. Each of the the following n lines contains a single
integer 0 ≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is terminated by a sequence of length n = 0. This sequence must not be processed.
Output
For every input sequence, your program prints a single line containing an integer number op, the minimum number of swap operations necessary to sort the given input sequence.
Sample Input
5 9 1 0 5 4 3 1 2 3 0
Sample Output
6 0
题意:给你一个n个整数组成的序列,每次只能交换相邻的两个元素,问你最少要进行多少次交换才能使得整个整数序列上升有序。 普通方法肯定超时 用树状数组+离散化
因为数据大小范围为0~999,999,999,若用此数作为数组下标肯定会超出内存限制,所以采用离散化的方法先将数据范围缩小
输入的数据:9 1 0 5 4
排序的数据:0 1 4 5 9
排序的编码:3 2 5 4 1
离散化之后:1 2 3 4 5
最终的编码:1 2 3 4 5
最终的数据:5 2 1 4 3
树状数组:
输入一个数据看看前面比他大的数据有几个;求他们的和
#include<cstdio>
#include<cstring>
#include<cmath>
#include<queue>
#include<algorithm>
using namespace std;
int n;
struct node
{
int val,zb;
}a[500001];//初始数组
int b[500001];//离散化后新数组
int id[500001];//离散化后的新坐标
bool cmp(node a,node b)
{
return a.val <b.val ;
}
int lowbit(int x)
{
return x&-x;
}
void add(int x,int d)
{
while(x<=n)
{
b[x]+=d;
x+=lowbit(x);
}
}
int sum(int x)
{
int s=0;
while(x>0)
{
s+=b[x];
x-=lowbit(x);
}
return s;
}
int main()
{
while(scanf("%d",&n)!=EOF)
{
if(n==0)
{
break;
}
for(int i=1;i<=n;i++)
{
b[i]=0;
scanf("%d",&a[i].val );
a[i].zb =i;
}
sort(a+1,a+n+1,cmp);
for(int i=1;i<=n;i++)
{
id[a[i].zb ]=i;
}
long long ans=0;
for(int i=1;i<=n;i++)
{
add(id[i],1);
ans+=(i-sum(id[i]));
}
printf("%lld
",ans);
}
return 0;
}