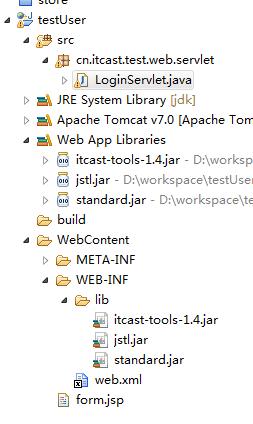
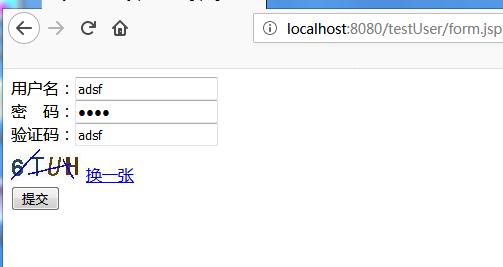
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>testUser</display-name> <servlet> <servlet-name>VerifyCodeServlet</servlet-name> <servlet-class>cn.itcast.vcode.servlet.VerifyCodeServlet</servlet-class> </servlet> <servlet> <servlet-name>LoginServlet</servlet-name> <servlet-class>cn.itcast.test.web.servlet.LoginServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>VerifyCodeServlet</servlet-name> <url-pattern>/VerifyCodeServlet</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>LoginServlet</servlet-name> <url-pattern>/LoginServlet</url-pattern> </servlet-mapping> <!-- 处理全站请求编码,无论是GET还是POST,默认是UTF-8 --> <filter> <filter-name>EncodingFilter</filter-name> <filter-class>cn.itcast.filter.EncodingFilter</filter-class> <init-param> <param-name>charset</param-name> <param-value>utf-8</param-value> </init-param> </filter> <filter-mapping> <filter-name>EncodingFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <title>My JSP 'form.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> <script type="text/javascript"> function _hyz() { /* 1. 获取<img>元素 2. 给它的src指向为/tools/VerifyCodeServlet */ var img = document.getElementById("imgVerifyCode"); // 需要给出一个参数,这个参数每次都不同,这样才能干掉浏览器缓存! img.src = "/testUser/VerifyCodeServlet?a=" + new Date().getTime(); } </script> </head> <body> <%-- 1. 写表单,其中包含图片(验证码) 2. 让图片显示出来: 把<img>的src指向VerifyCodeServlet,你需要在web.xml中部署VerfiyCodeServlet 3. 换一张 --%> <form action="/testUser/LoginServlet" method="post"> <%-- 添加一个参数:method=login --%> <input type="hidden" name="method" value="login"> 用户名:<input type="text" name="name"/><br/> 密 码:<input type="password" name="pwd"/><br/> 验证码:<input type="text" name="verifyCode"/><br/> <img src="/testUser/VerifyCodeServlet" id="imgVerifyCode"/> <a href="javascript:_hyz()">换一张</a> <br/> <input type="submit" value="提交"/> </form> </body> </html>
package cn.itcast.test.web.servlet; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import cn.itcast.servlet.BaseServlet; public class LoginServlet extends BaseServlet { public String login(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { /* * 校验验证码 * 1. 获取表单中的验证码 * 2. 获取图片上的文字 */ String verifyCode = request.getParameter("verifyCode"); // VerifyCodeServlet会把真正的验证码保存到session中 String vcode = (String) request.getSession().getAttribute("vCode"); System.out.println(verifyCode.equalsIgnoreCase(vcode)); return "/form.jsp"; } }
第二种实现二维码验证
package com.atguigu.javaweb; import java.io.IOException; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class CheckCodeServlet extends HttpServlet { private static final long serialVersionUID = 1L; protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //1. 获取请求参数: CHECK_CODE_PARAM_NAME String paramCode = request.getParameter("CHECK_CODE_PARAM_NAME"); //2. 获取 session 中的 CHECK_CODE_KEY 属性值 String sessionCode = (String)request.getSession().getAttribute("CHECK_CODE_KEY"); System.out.println(paramCode); System.out.println(sessionCode); //3. 比对. 看是否一致, 若一致说明验证码正确, 若不一致, 说明验证码错误 if(!(paramCode != null && paramCode.equals(sessionCode))){ request.getSession().setAttribute("message", "验证码不一致!"); response.sendRedirect(request.getContextPath() + "/index.jsp"); return; } System.out.println("受理请求!"); } }
package com.atguigu.javaweb; import java.awt.Color; import java.awt.Font; import java.awt.Graphics2D; import java.awt.image.BufferedImage; import java.io.IOException; import java.util.Random; import javax.imageio.ImageIO; import javax.servlet.ServletException; import javax.servlet.ServletOutputStream; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class ValidateColorServlet extends HttpServlet { public static final String CHECK_CODE_KEY = "CHECK_CODE_KEY"; private static final long serialVersionUID = 1L; //设置验证图片的宽度, 高度, 验证码的个数 private int width = 152; private int height = 40; private int codeCount = 6; //验证码字体的高度 private int fontHeight = 4; //验证码中的单个字符基线. 即:验证码中的单个字符位于验证码图形左上角的 (codeX, codeY) 位置处 private int codeX = 0; private int codeY = 0; //验证码由哪些字符组成 char [] codeSequence = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz23456789".toCharArray(); //初始化验证码图形属性 public void init(){ fontHeight = height - 2; codeX = width / (codeCount + 2); codeY = height - 4; } public void service(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { //定义一个类型为 BufferedImage.TYPE_INT_BGR 类型的图像缓存 BufferedImage buffImg = null; buffImg = new BufferedImage(width, height, BufferedImage.TYPE_3BYTE_BGR); //在 buffImg 中创建一个 Graphics2D 图像 Graphics2D graphics = null; graphics = buffImg.createGraphics(); //设置一个颜色, 使 Graphics2D 对象的后续图形使用这个颜色 graphics.setColor(Color.WHITE); //填充一个指定的矩形: x - 要填充矩形的 x 坐标; y - 要填充矩形的 y 坐标; width - 要填充矩形的宽度; height - 要填充矩形的高度 graphics.fillRect(0, 0, width, height); //创建一个 Font 对象: name - 字体名称; style - Font 的样式常量; size - Font 的点大小 Font font = null; font = new Font("", Font.BOLD, fontHeight); //使 Graphics2D 对象的后续图形使用此字体 graphics.setFont(font); graphics.setColor(Color.BLACK); //绘制指定矩形的边框, 绘制出的矩形将比构件宽一个也高一个像素 graphics.drawRect(0, 0, width - 1, height - 1); //随机产生 15 条干扰线, 使图像中的认证码不易被其它程序探测到 Random random = null; random = new Random(); graphics.setColor(Color.GREEN); for(int i = 0; i < 55; i++){ int x = random.nextInt(width); int y = random.nextInt(height); int x1 = random.nextInt(20); int y1 = random.nextInt(20); graphics.drawLine(x, y, x + x1, y + y1); } //创建 randomCode 对象, 用于保存随机产生的验证码, 以便用户登录后进行验证 StringBuffer randomCode; randomCode = new StringBuffer(); for(int i = 0; i < codeCount; i++){ //得到随机产生的验证码数字 String strRand = null; strRand = String.valueOf(codeSequence[random.nextInt(36)]); //把正在产生的随机字符放入到 StringBuffer 中 randomCode.append(strRand); //用随机产生的颜色将验证码绘制到图像中 graphics.setColor(Color.BLUE); graphics.drawString(strRand, (i + 1)* codeX, codeY); } //再把存放有所有随机字符的 StringBuffer 对应的字符串放入到 HttpSession 中 request.getSession().setAttribute(CHECK_CODE_KEY, randomCode.toString()); //禁止图像缓存 response.setHeader("Pragma", "no-cache"); response.setHeader("Cache-Control", "no-cache"); response.setDateHeader("Expires", 0); //将图像输出到输出流中 ServletOutputStream sos = null; sos = response.getOutputStream(); ImageIO.write(buffImg, "jpeg", sos); sos.close(); } }
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>Validate</display-name> <servlet> <servlet-name>validateColorServlet</servlet-name> <servlet-class>com.atguigu.javaweb.ValidateColorServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>validateColorServlet</servlet-name> <url-pattern>/validateColorServlet</url-pattern> </servlet-mapping> <servlet> <description></description> <display-name>CheckCodeServlet</display-name> <servlet-name>CheckCodeServlet</servlet-name> <servlet-class>com.atguigu.javaweb.CheckCodeServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>CheckCodeServlet</servlet-name> <url-pattern>/checkCodeServlet</url-pattern> </servlet-mapping> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
<%@page import="java.util.Date"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Insert title here</title> </head> <body> <font color="red"> <%= session.getAttribute("message") == null ? "" : session.getAttribute("message")%> </font> <form action="<%= request.getContextPath() %>/checkCodeServlet" method="post"> name: <input type="text" name="name"/> checkCode: <input type="text" name="CHECK_CODE_PARAM_NAME"/> <a href="javascript:getImger()">换一张</a> <img alt="" src="<%= request.getContextPath() %>/validateColorServlet" id="ims"> <input type="submit" value="Submit"/> </form> </body> <script type="text/javascript"> function getImger(){ var img=document.getElementById("ims"); img.src="<%= request.getContextPath() %>/validateColorServlet?a="+new Date().getTime(); } </script> </html>