/*
Flutter AspectRatio、Cart卡片组件:
AspectRatio的作用是根据设置调整子元素child的宽高比。
AspectRatio首先会在布局限制条件允许的范围内尽可能的扩展,widget的高度是由宽度和比率决定的。
类似于BoxFit中的contain,按照固定比率去尽量占满区域。
如果在满足所有限制条件过后无法找到一个可行的尺寸,AspectRatio最终将会去优先适应布局限制条件,而忽略所设置的比率。
*/
main.dart
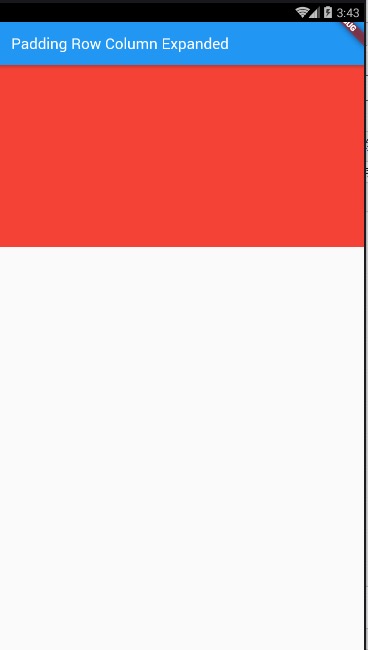
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { // TODO: implement build return MaterialApp( home: Scaffold( appBar: AppBar( title: Text(' AspectRatio、Cart卡片组件'), ), body: HomeContent(), ), ); } } class HomeContent extends StatelessWidget { @override Widget build(BuildContext context) { //Stack结合align实现布局: return AspectRatio( aspectRatio: 2.0/1.0, child: Container( color: Colors.red, ), ); } }
Cart卡片组件:
demo1:
main.dart
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { // TODO: implement build return MaterialApp( home: Scaffold( appBar: AppBar( title: Text(' AspectRatio、Cart卡片组件'), ), body: HomeContent(), ), ); } } class HomeContent extends StatelessWidget { @override Widget build(BuildContext context) { //Stack结合align实现布局: return ListView( children: <Widget>[ Card( child: Column( children: <Widget>[ ListTile(title: Text('中国'), subtitle: Text('山东')), ListTile( title: Text('青岛'), ), ListTile( title: Text('烟台'), ) ], ), ), Card( child: Column( children: <Widget>[ ListTile(title: Text('中国'), subtitle: Text('山东')), ListTile( title: Text('青岛'), ), ListTile( title: Text('烟台'), ) ], ), ) ], ); } }
demo2:
main.dat
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
// TODO: implement build
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text(' AspectRatio、Cart卡片组件'),
),
body: HomeContent(),
),
);
}
}
class HomeContent extends StatelessWidget {
@override
Widget build(BuildContext context) {
//Stack结合align实现布局:
return ListView(
children: <Widget>[
Card(
margin: EdgeInsets.all(10),
child: Column(
children: <Widget>[
AspectRatio(
aspectRatio: 16 / 9,
child: Image.network(
'https://www.itying.com/images/flutter/1.png',fit: BoxFit.cover),
),
ListTile(
leading: ClipOval(
child: Image.network('https://www.itying.com/images/flutter/1.png',fit: BoxFit.cover, 50,height: 50),
),
title: Text('data'),
subtitle: Text('data'))
],
),
),
Card(
margin: EdgeInsets.all(10),
child: Column(
children: <Widget>[
AspectRatio(
aspectRatio: 16 / 9,
child: Image.network(
'https://www.itying.com/images/flutter/1.png',fit: BoxFit.cover),
),
ListTile(
leading: CircleAvatar(
backgroundImage: NetworkImage(
'https://www.itying.com/images/flutter/1.png'),
),
title: Text('data'),
subtitle: Text('data'))
],
),
)
],
);
}
}
demo3:
main.dart
import 'package:flutter/material.dart'; import 'res/listData.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { // TODO: implement build return MaterialApp( home: Scaffold( appBar: AppBar( title: Text(' AspectRatio、Cart卡片组件'), ), body: HomeContent(), ), ); } } class HomeContent extends StatelessWidget { @override Widget build(BuildContext context) { //Stack结合align实现布局: return ListView( children: listData.map((value) { return Card( margin: EdgeInsets.all(10), child: Column( children: <Widget>[ AspectRatio( aspectRatio: 16 / 9, child: Image.network( "${value['imageUrl']}", fit: BoxFit.cover), ), ListTile( leading: CircleAvatar( backgroundImage: NetworkImage( "${value['imageUrl']}"), ), title: Text("${value['title']}"), subtitle: Text("${value['descripts']}",maxLines:2,overflow: TextOverflow.ellipsis,)) ], ), ); }).toList(), ); } }
listData.dart
List listData=[ { "title":"Candy Shop", "author":"Mohamed Chahin", "imageUrl":"https://www.itying.com/images/flutter/1.png", "descripts":"MohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahin" }, { "title":"Candy Shop", "author":"Mohamed Chahin", "imageUrl":"https://www.itying.com/images/flutter/2.png", "descripts":"MohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahin" }, { "title":"Candy Shop", "author":"Mohamed Chahin", "imageUrl":"https://www.itying.com/images/flutter/3.png", "descripts":"MohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahin" }, { "title":"Candy Shop", "author":"Mohamed Chahin", "imageUrl":"https://www.itying.com/images/flutter/4.png", "descripts":"MohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahin" },{ "title":"Candy Shop", "author":"Mohamed Chahin", "imageUrl":"https://www.itying.com/images/flutter/5.png", "descripts":"MohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahinMohamedChahin" } ];