实验任务详情:
完成火车站售票程序的模拟。
要求:
(1)总票数1000张;
(2)10个窗口同时开始卖票;
(3)卖票过程延时1秒钟;
(4)不能出现一票多卖或卖出负数号票的情况。
实验代码:
Thread类
package work6;
public class MyThread implements Runnable{
private int ticket=999;
public int getTicket() {
return ticket;
}
public void setTicket(int ticket) {
this.ticket = ticket;
}
public void run() {
for(int i=0;i<1000;i++) {
synchronized (this) {
if(ticket>=0) {
try {
Thread.sleep(0);
}catch(InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName()+"卖出一张票,还剩"+ticket+"张");
ticket--;
}
}
}
}
}
测试类
package work6;
public class Test {
public static void main(String[] args) {
MyThread my=new MyThread();
Thread w1 = new Thread(my,"一号窗口");
Thread w2 = new Thread(my,"二号窗口");
Thread w3 = new Thread(my,"三号窗口");
Thread w4 = new Thread(my,"四号窗口");
Thread w5 = new Thread(my,"五号窗口");
Thread w6 = new Thread(my,"六号窗口");
Thread w7 = new Thread(my,"七号窗口");
Thread w8 = new Thread(my,"八号窗口");
Thread w9 = new Thread(my,"九号窗口");
Thread w10 = new Thread(my,"十号窗口");
w1.setPriority(Thread.NORM_PRIORITY);
w2.setPriority(Thread.MIN_PRIORITY);
w3.setPriority(Thread.MAX_PRIORITY);
w1.start();
w2.start();
w3.start();
w4.start();
w5.start();
w6.start();
w7.start();
w8.start();
w9.start();
w10.start();
}
}
运行截图:
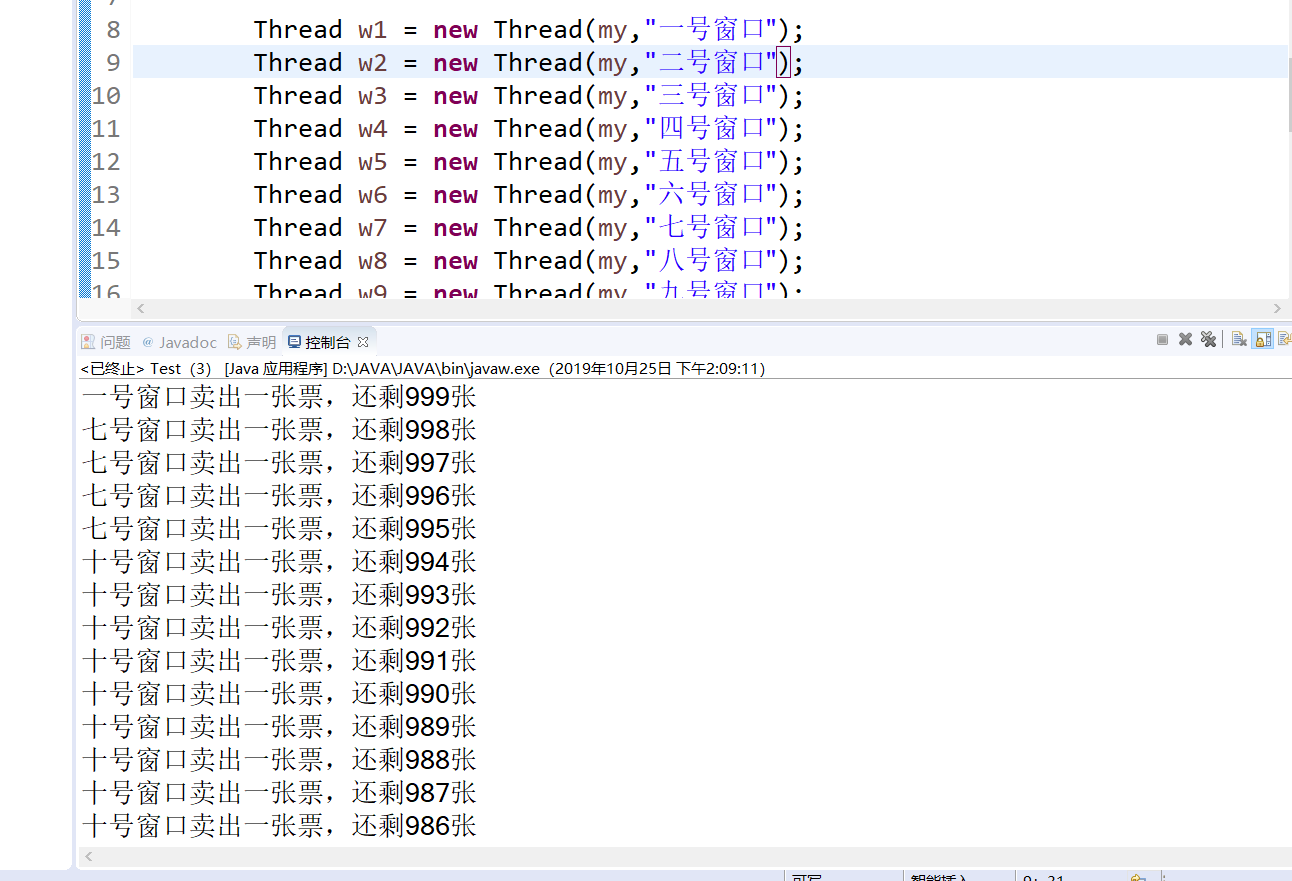
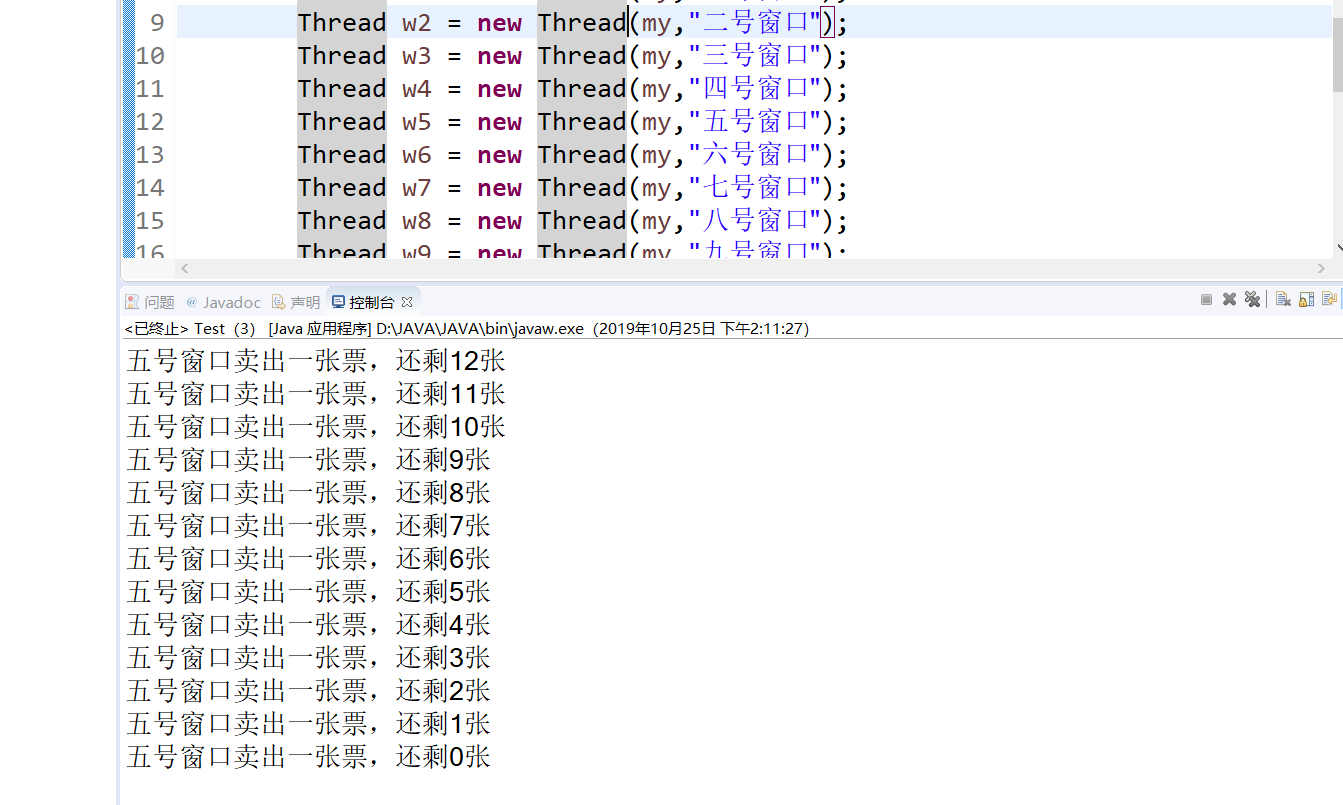
学习总结:
1、同步
同步代码块:
synchronized(同步对象){ ///synchronized(this)this表示当前对象
需要同步的代码块;
}
同步方法:
synchronized 方法返回值 方法名称(参数列表){
方法体
}
2、 Flile的主要用法和常量:
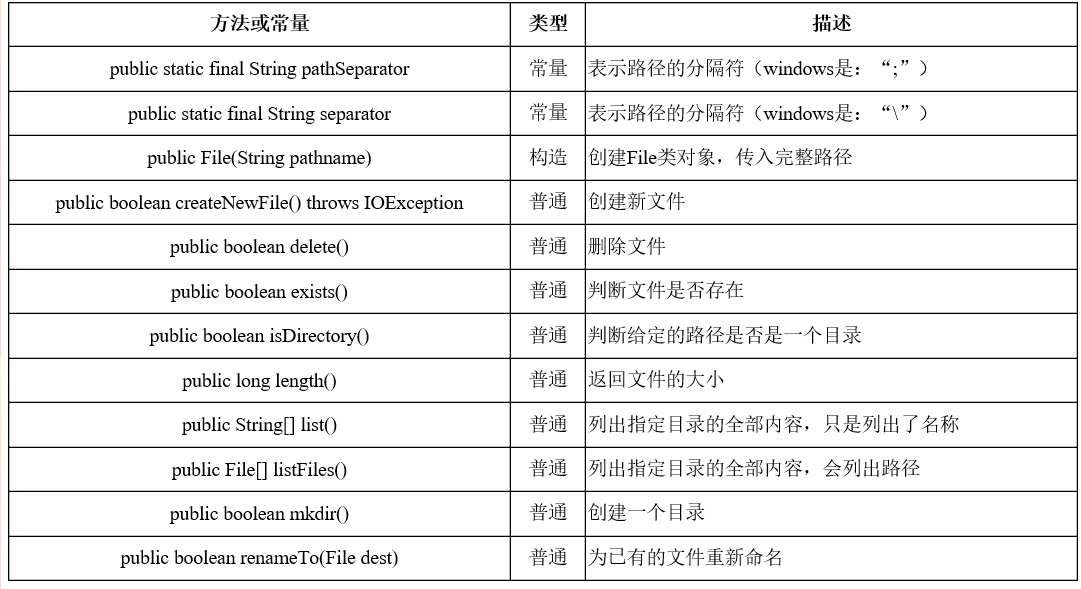
3、使用File类操作文件:
使用createNewFile()
File f = new File("路径");
try{
f.createNewFile();
}catch(IOException e){
e.printStackTrace();
}
使用delete()方法:
File f = new File("路径");
f.delete();
使用exists()方法:
package org.lxh.demo12.filedemo;
import java.io.File;
public class FileDemo05{
public static void mian(String args[]){
File f =new File("d:"+File.separator+"test.txt");
if(f.exist()){
f.delete();
}
}
}
使用mkdir()方法
package org.lxh.demo12.filedemo;
import java.io.File;
public class FileDemo05{
public static void mian(String args[]){
File f =new File("d:"+File.separator+"test.txt");
if(f.getParentFile().exist()){
f.mkdir();
}
}
}
4、多线程的实现主要有两种:
1.继承thread类;
2实现runnable接口;
start()是多线程的启动方法;
run()是多线程的主体;
两种的区别:
thread不能资源共享;
runnable能实现资源共享;