import Vue from 'vue';
import { Uploader } from 'vant';
Vue.use(Uploader)
添加图片
<van-uploader @delete="deleteChange" v-model="fileList" multiple :max-count="4" :after-read="afterRead" :before-read="beforeRead" capture="camera" />
1. 在data 中声明 fileList: [],
fileList 是个数组: 动态绑定添加数组的每个图片的信息: 其中每个对象里面的content 是我们获取的图片的base64 二进制码,需要传给后台的;
:after-read 事件在每次添加图片的时候触发;不添加不触发;
@delete 事件在每次点击删除图片的时候触发;
所以: 我们要把[图片base64二进制码,...]传给后台
fileListNewArr: [], // 上传照片base64数组
afterRead(file) {
this.fileListNewArr = [] ;
if (this.fileList.length > 0 ){
for (var i = 0 ;i < this.fileList.length ; i++){
this.fileListNewArr.push(this.fileList[i].content)
}
}
},
deleteChange(file){
console.log(file,"点击删除按钮开始触发,file 里面是每个删除图片的相关信息")
this.fileListNewArr = [] ;
if (this.fileList.length > 0 ){
for (var i = 0 ;i < this.fileList.length ; i++){
this.fileListNewArr.push(this.fileList[i].content)
}
}
},
//补充说明
beforeRead (file) { //上传之前校验
if (file.type !== 'image/jpeg' && file.type !== 'image/png') {
Toast('只允许上传jpg/png格式的图片!')
return false
}
return true
},
// 需要注意的是;"data:image/jpeg;base64,/9j/4AAQSkZJRgABAQEAYABgAAD....
传给后端的base64码,需要去掉 data:image/jpeg;base64 部分;一般我选择后端去掉
在调用上传图片接口时进行验证:
if (this.fileListNewArr.length == 0 ){
Toast.fail('请上传图片');
return
}
传给后台的关于图片的参数就是
pictureList: this.fileListNewArr,
一般值得注意的是 :
capture="camera" 需要加上,安卓或IOS 开放了相册的图片就可以直接调用;
后端返回的是 {pictureId: 209, pictureUrl: "group1/M00/13/97/CgEUdl7rE7aAEqdKAEy1PIez7yo252.jpg}
其中 group1/M00/13/97/CgEUdl7rE7aAEqdKAEy1PIez7yo252.jpg 和 imgurl 进行拼接即可在浏览器上进行图片查看
2,修改图片上传
2-1:在图片修改之前首先要解决的是图片预览问题
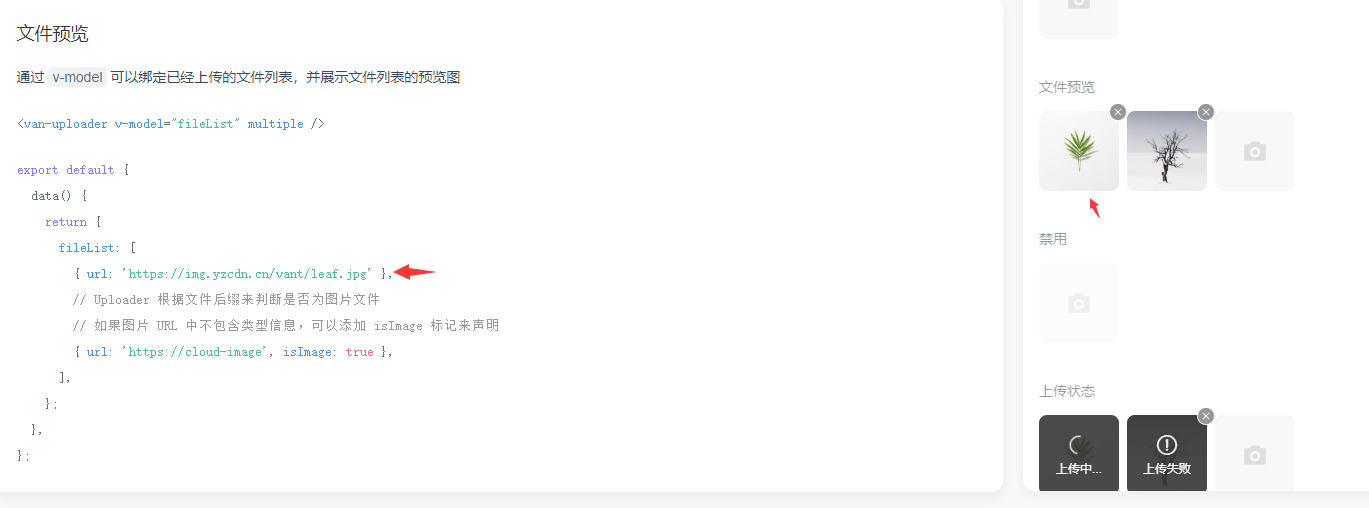
如果想要图片预览,那么 v-model 绑定的list 必须是包含已经上传图片的拼接完整之后的url,而且封装后的数组里面的对象,包含 key : url ;
由于后台返回的接口是;[
{pictureId: 209, pictureUrl: "group1/M00/13/97/CgEUdl7rE7aAEqdKAEy1PIez7yo252.jpg} ,
{pictureId: 211, pictureUrl: "group1/M00/13/97/CgEUdl7rE7aAEqdKAEy1PIez7yo252.jpg}
]
则接口拿到返回结果可以对其进行处理:
this.pictureUrlArr = res.data.pictures ; //接口返回结果
var imgUrl = "https://assettest.hzncc.cn/HncWeb/"
this.pictureUrlArrWithImgUrl = []; // 赋值给v-model 进行显示
this.pictureIdsBeforeChange = []; // 已经上传的图片的id 的汇总,之后修改有用
for (var i = 0 ; i< this.pictureUrlArr.length ; i++){
var obj = {};
obj.url = imgUrl + this.pictureUrlArr[i].pictureUrl ;
obj.pictureId = this.pictureUrlArr[i].pictureId ;
this.pictureUrlArrWithImgUrl.push(obj);
this.pictureIdsBeforeChange.push(this.pictureUrlArr[i].pictureId) /*获取修改之前的已经存在的图片的ids*/
}
下面开始对图片进行操作:
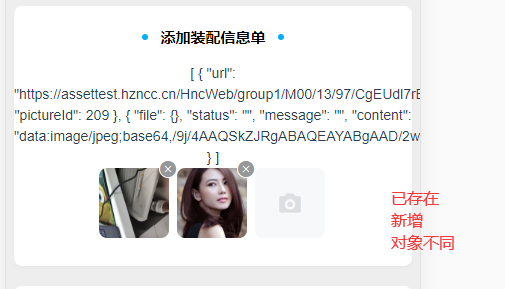
考虑到对于修改: 1 :不修改 2 :混合(已经存在 & 新增) 3 :去掉之前,只剩新增
所以和后台经过沟通,建议传 : 1.已经存在的图片ids 的数组 2.新增图片的数组[base64编码]
所以:
同时由于如果不修改,则情况特殊不会触发 :after-read 和 deleteChange 事件所以 会在data 里面声明一个表示参数 :
imgHasChange :fase 表示图片未对其进行修改;
<van-uploader v-model="pictureUrlArrWithImgUrl" multiple :max-count="4" capture="camera" @delete="deleteChange" :after-read="afterRead" :before-read="beforeRead" />
data里面:pictureUrlArrWithImgUrl :[],
afterRead(file) {
this.imgHasChange = true //表明图片已经发生了改变,进行表示 data 里面初始化未 fase:注意
this.pictureList = [] ; //新增图片base64数组
this.pictureIds = [] ; //图片id数组
if (this.pictureUrlArrWithImgUrl.length > 0 ){
for (var i = 0 ;i < this.pictureUrlArrWithImgUrl.length ; i++){
if (this.pictureUrlArrWithImgUrl[i].hasOwnProperty("pictureId")){
this.pictureIds.push(this.pictureUrlArrWithImgUrl[i].pictureId)
}
if (this.pictureUrlArrWithImgUrl[i].hasOwnProperty("content")){
this.pictureList.push(this.pictureUrlArrWithImgUrl[i].content)
}
}
}
},
deleteChange(){
this.imgHasChange = true //表明图片已经发生了改变
this.pictureList = [] ; //新增图片base64数组
this.pictureIds = [] ; //图片id数组
if (this.pictureUrlArrWithImgUrl.length > 0 ){
for (var i = 0 ;i < this.pictureUrlArrWithImgUrl.length ; i++){
if (this.pictureUrlArrWithImgUrl[i].hasOwnProperty("pictureId")){
this.pictureIds.push(this.pictureUrlArrWithImgUrl[i].pictureId)
}
if (this.pictureUrlArrWithImgUrl[i].hasOwnProperty("content")){
this.pictureList.push(this.pictureUrlArrWithImgUrl[i].content)
}
}
}
},
最后:调用修改接口:
if (this.pictureUrlArrWithImgUrl.length == 0 ){ //需要修改可能根据情况
Toast.fail('请上传图片');
return
}
pictureIds: this.imgHasChange ? this.pictureIds : this.pictureIdsBeforeChange, //图片id
pictureList:this.imgHasChange ? this.pictureList : [], //base64 如果没任何改变则 不会有新的base64的编码产生
3.如果只是对图片进行预览,无需进行编辑则
<van-uploader v-model="pictureUrlArrWithImgUrl" multiple :max-count="pictureUrlArrWithImgUrl.length" :deletable="
isDeleteShow 设置为fase ;表示不可编辑; 同时
:max-count="pictureUrlArrWithImgUrl.length" 就不会有未上传的图标在右边出现;
在此,还有一个问题尚待解决:后台返回图片,有的图片第一次返回404 ,但是第二次访问即可成功;此解决方案待分享;