第一步:编写application.yml配置文件
spring:
datasource:
system:
jdbc-url: jdbc:oracle:thin:@localhost:1521/orcl
driver-class-name: oracle.jdbc.OracleDriver
username: system
password: 1234
initial-size: 5
min-idle: 5
max-active: 20
min-evictable-idle-time-millis: 300000
validation-query: SELECT 1 FROM DUAL
test-while-idle: true
kllogt:
jdbc-url: jdbc:oracle:thin:@localhost:1521/orcl
driver-class-name: oracle.jdbc.OracleDriver
username: kllogt
password: kllogt
initial-size: 5
min-idle: 5
max-active: 20
min-evictable-idle-time-millis: 300000
validation-query: SELECT 1 FROM DUAL
test-while-idle: true
logging:
level:
org.springframework.web: debug
第二部编写两个数据源的配置类
配置类SystemResource
package www.it.com.springbootresource.util;
import org.apache.ibatis.io.VFS;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.mybatis.spring.SqlSessionTemplate;
import org.mybatis.spring.annotation.MapperScan;
import org.mybatis.spring.boot.autoconfigure.SpringBootVFS;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import javax.sql.DataSource;
//表示这个类为一个配置类
@Configuration
// 配置mybatis的接口类放的地方
@MapperScan(basePackages = "www.it.com.springbootresource.system.dao", sqlSessionFactoryRef = "SystemSqlSessionFactory")
public class SystemResource {
// 将这个对象放入Spring容器中
@Bean(name = "SystemDataSource")
// 表示这个数据源是默认数据源
@Primary
// 读取application.properties中的配置参数映射成为一个对象
// prefix表示参数的前缀
@ConfigurationProperties(prefix = "spring.datasource.system")
public DataSource getDateSource1() {
return DataSourceBuilder.create().build();
}
@Bean(name = "SystemSqlSessionFactory")
// 表示这个数据源是默认数据源
@Primary
// @Qualifier表示查找Spring容器中名字为SystemDataSource的对象
public SqlSessionFactory SystemSqlSessionFactory(@Qualifier("SystemDataSource") DataSource datasource)
throws Exception {
SqlSessionFactoryBean bean = new SqlSessionFactoryBean();
bean.setDataSource(datasource);
VFS.addImplClass(SpringBootVFS.class);//mybatis的facroty需要加载SpringBoot独特的虚拟文件系统,才能识别类路
PathMatchingResourcePatternResolver resolver = new PathMatchingResourcePatternResolver();
//bean.setConfigLocation(resolver.getResource("classpath:mybatis-config.xml"));
//bean.setMapperLocations(
// 设置mybatis的xml所在位置
//new PathMatchingResourcePatternResolver().getResources("classpath:mybatis/mapper/m1/*.xml"));
return bean.getObject();
}
@Bean("SystemSqlSessionTemplate")
// 表示这个数据源是默认数据源
@Primary
public SqlSessionTemplate Systemsqlsessiontemplate(
@Qualifier("SystemSqlSessionFactory") SqlSessionFactory sessionfactory) {
return new SqlSessionTemplate(sessionfactory);
}
}
KllogtResource配置类
package www.it.com.springbootresource.util;
import org.apache.ibatis.io.VFS;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.mybatis.spring.SqlSessionTemplate;
import org.mybatis.spring.annotation.MapperScan;
import org.mybatis.spring.boot.autoconfigure.SpringBootVFS;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import javax.sql.DataSource;
/**
* @author wangjie
* @date 2019/11/3 16:36
* @description
* @company 石文软件有限公司
*/
//表示这个类为一个配置类
@Configuration
// 配置mybatis的接口类放的地方
@MapperScan(basePackages = "www.it.com.springbootresource.kllogt.dao", sqlSessionFactoryRef = "KllogtSqlSessionFactory")
public class KllogtResource {
@Bean(name = "KllogtDataSource")
@ConfigurationProperties(prefix = "spring.datasource.kllogt")
public DataSource getDateSource2() {
return DataSourceBuilder.create().build();
}
@Bean(name = "KllogtSqlSessionFactory")
public SqlSessionFactory KllogtSqlSessionFactory(@Qualifier("KllogtDataSource") DataSource datasource)
throws Exception {
SqlSessionFactoryBean bean = new SqlSessionFactoryBean();
bean.setDataSource(datasource);
VFS.addImplClass(SpringBootVFS.class);//mybatis的facroty需要加载SpringBoot独特的虚拟文件系统,才能识别类路
PathMatchingResourcePatternResolver resolver = new PathMatchingResourcePatternResolver();
//bean.setConfigLocation(resolver.getResource("classpath:mybatis-config.xml"));
// bean.setMapperLocations(
// new PathMatchingResourcePatternResolver().getResources("classpath:mybatis/mapper/m2/*.xml"));
return bean.getObject();
}
@Bean("KllogtSqlSessionTemplate")
public SqlSessionTemplate Kllogtsqlsessiontemplate(
@Qualifier("KllogtSqlSessionFactory") SqlSessionFactory sessionfactory) {
return new SqlSessionTemplate(sessionfactory);
}
}
项目结构
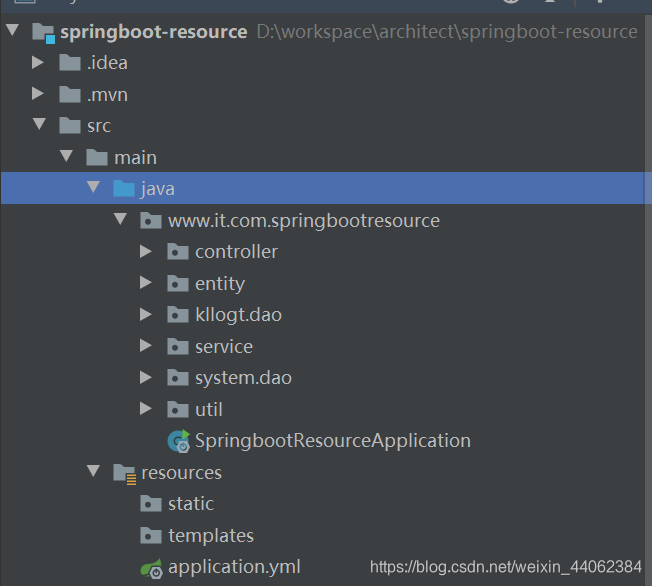
自己的pom文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.0.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>www.it.cpm</groupId>
<artifactId>springboot-resource</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot-resource</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>com.oracle</groupId>
<artifactId>ojdbc6</artifactId>
<version>11.2.0.3</version>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.persistence/persistence-api -->
<dependency>
<groupId>javax.persistence</groupId>
<artifactId>persistence-api</artifactId>
<version>1.0.2</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.10</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>