Problem Description
A tree is a well-known data structure that is either empty (null, void, nothing) or is a set of one or more nodes connected by directed edges between nodes satisfying the following properties.
There is exactly one node, called the root, to which no directed edges point.
Every node except the root has exactly one edge pointing to it.
There is a unique sequence of directed edges from the root to each node.
For example, consider the illustrations below, in which nodes are represented by circles and edges are represented by lines with arrowheads. The first two of these are trees, but the last is not.
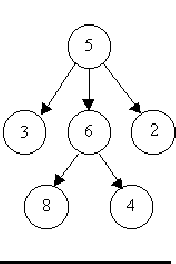
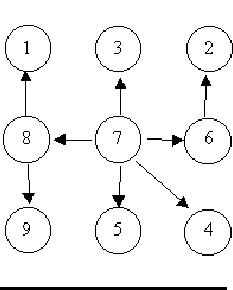

In this problem you will be given several descriptions of collections of nodes connected by directed edges. For each of these you are to determine if the collection satisfies the definition of a tree or not.
There is exactly one node, called the root, to which no directed edges point.
Every node except the root has exactly one edge pointing to it.
There is a unique sequence of directed edges from the root to each node.
For example, consider the illustrations below, in which nodes are represented by circles and edges are represented by lines with arrowheads. The first two of these are trees, but the last is not.
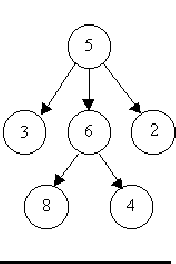
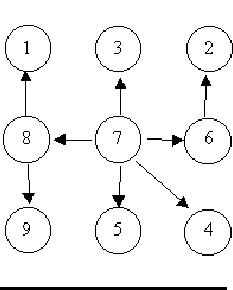

In this problem you will be given several descriptions of collections of nodes connected by directed edges. For each of these you are to determine if the collection satisfies the definition of a tree or not.
Input
The input will consist of a sequence of descriptions (test cases) followed by a pair of negative integers. Each test case will consist of a sequence of edge descriptions followed by a pair of zeroes Each edge description will consist of a pair of integers; the first integer identifies the node from which the edge begins, and the second integer identifies the node to which the edge is directed. Node numbers will always be greater than zero.
Output
For each test case display the line ``Case k is a tree." or the line ``Case k is not a tree.", where k corresponds to the test case number (they are sequentially numbered starting with 1).
Sample Input
6 8 5 3 5 2 6 4
5 6 0 0
8 1 7 3 6 2 8 9 7 5
7 4 7 8 7 6 0 0
3 8 6 8 6 4
5 3 5 6 5 2 0 0
-1 -1
Sample Output
Case 1 is a tree.
Case 2 is a tree.
Case 3 is not a tree.
题意:给你一些有向边,问是否能构成一棵树。。
思路:和HDU 1272 小希的迷宫不同,本题是有向图,那道题是无向图
1、把树的边当成是无向边,则所有的点属于同一个集合,使用并查集
2、所有点至多只能有一个入度。
3、根节点只能有一个
4、特判0,0,
1 #include <stdio.h> 2 #include <string.h> 3 #define MAXN (1002) 4 5 int set[MAXN]; 6 int flag, tcase; 7 8 void init() 9 { 10 int i; 11 memset(set, -1, sizeof(set)); 12 flag = 0; 13 } 14 15 int find(int x) 16 { 17 if (set[x] == -1) 18 set[x] = x; 19 if (set[x] == x) 20 return x; 21 return set[x] = find(set[x]); 22 } 23 24 int main() 25 { 26 int i, j; 27 int x, y; 28 tcase = 1; 29 init(); 30 while (scanf("%d %d", &x, &y) != EOF) 31 { 32 if (x == -1 && y == -1) 33 break; 34 if (x == 0 && y == 0) 35 { 36 printf ("Case %d", tcase++); 37 if (!flag) 38 { 39 j = 0; 40 for (i = 0; i < MAXN; ++i) 41 if (set[i] == i) 42 ++j; 43 if (j > 1) // not a tree: 1 2 3 4 0 0, a tree: 0 0 44 flag = 1; 45 } 46 if (!flag) 47 { 48 printf (" is a tree. "); 49 } 50 else 51 printf (" is not a tree. "); 52 init(); 53 continue; 54 } 55 int px = find(x); 56 int py = find(y); 57 58 if (py != y && px != py || x == y || (x != y && px == py))// not a tree: 1 2 1 3 2 1 0 0 59 {// not a tree: 1 1 0 0 60 flag = 1; 61 } 62 else 63 set[py] = set[px]; 64 } 65 return 0; 66 }